How to implement WebSocket two-way communication in golang
How to implement two-way communication of WebSocket in Golang
WebSockets is a communication protocol that establishes a persistent connection between the client and the server, which allows two-way communication , and performs well in terms of real-time performance and efficiency. In Golang, we can use the net/http
and gorilla/websocket
packages in the standard library to implement WebSocket two-way communication. This article will introduce how to implement WebSocket two-way communication in Golang and provide specific code examples.
Step 1: Import dependency packages
First, we need to import the net/http
and gorilla/websocket
packages. The net/http
package provides HTTP server and client functions, while the gorilla/websocket
package is a WebSocket implementation library.
import ( "log" "net/http" "github.com/gorilla/websocket" )
Step 2: Set the WebSocket processing function
Next, we need to set the WebSocket processing function. This function will handle the client's connection request and process the message sent by the client after the connection is successfully established.
func websocketHandler(w http.ResponseWriter, r *http.Request) { // 将 HTTP 连接升级为 WebSocket 连接 ws, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println("Error upgrading HTTP connection to WebSocket:", err) return } // 在连接结束时关闭 WebSocket defer ws.Close() // 循环处理客户端发来的消息 for { // 读取客户端发来的消息 messageType, message, err := ws.ReadMessage() if err != nil { log.Println("Error reading message from WebSocket:", err) break } // 打印收到的消息 log.Printf("Received message: %s", message) // 回复客户端收到的消息 if err = ws.WriteMessage(messageType, message); err != nil { log.Println("Error writing message to WebSocket:", err) break } } }
Step 3: Set up the WebSocket upgrader
In the processing function, we need to use the upgrader (upgrader
) of the gorilla/websocket
package to upgrade the HTTP connection to a WebSocket connection. The upgrader can also set some parameters such as allowed domains and handshake timeout.
var upgrader = websocket.Upgrader{ // 允许跨域访问 CheckOrigin: func(r *http.Request) bool { return true }, }
Step 4: Set up the HTTP server
Finally, we need to set up the HTTP server and map the processing function to the corresponding path so that the client can connect to WebSocket through the path.
func main() { // 设置 WebSocket 处理函数 http.HandleFunc("/", websocketHandler) // 启动 HTTP 服务器 err := http.ListenAndServe(":8080", nil) if err != nil { log.Fatal("Error starting HTTP server:", err) } }
:8080
in the code example is the listening address and port number of the server. You can modify it as needed.
Using the above code, we have successfully implemented the function of two-way communication through WebSocket in Golang. After the client connection is successful, you can use code similar to the following to send and receive messages:
const socket = new WebSocket("ws://localhost:8080/"); socket.addEventListener("open", (event) => { socket.send("Hello, server!"); }); socket.addEventListener("message", (event) => { console.log("Received message from server:", event.data); }); socket.addEventListener("close", (event) => { console.log("Server connection closed:", event); });
In summary, we have introduced in detail how to implement WebSocket two-way communication in Golang and provided specific Code examples. Through these sample codes, you can quickly get started and implement real-time communication functions based on WebSocket.
The above is the detailed content of How to implement WebSocket two-way communication in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
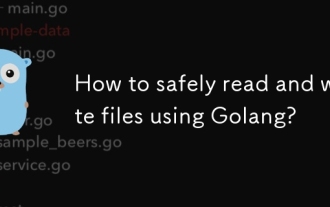
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
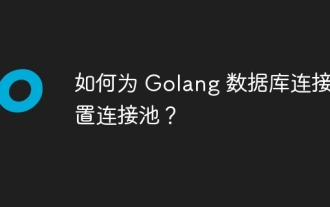
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
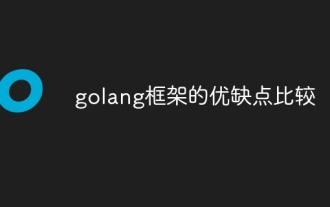
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
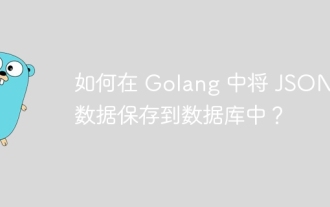
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
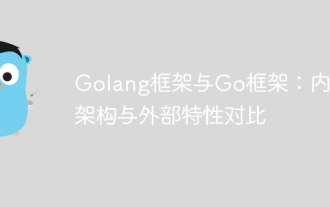
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
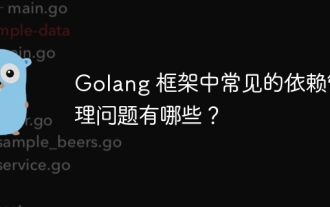
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
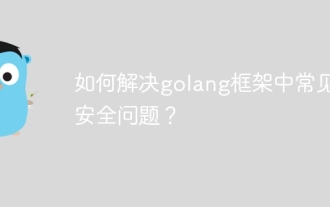
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
