Detailed explanation of reading and writing CSV files in Java using OpenCSV
Java is a widely used programming language, and developers often need to deal with various data formats. CSV (Comma-Separated Values) is a common data format widely used in data exchange and storage. In Java, we can use the OpenCSV library to read and write CSV files.
OpenCSV is an easy-to-use open source library that provides a convenient API to process CSV data. This article will introduce how to use OpenCSV to read and write CSV files in Java.
1. Add OpenCSV dependency
First, we need to add OpenCSV dependency to the project. Project dependencies can be managed through Maven, just add the following code in the pom.xml file:
<dependency> <groupId>com.opencsv</groupId> <artifactId>opencsv</artifactId> <version>5.5.1</version> </dependency>
2. Read CSV files
To read CSV files, we need to create A CSVReader object and use it to read data from the file. The following is a simple example:
import com.opencsv.CSVReader; public class CSVReaderExample { public static void main(String[] args) { try { CSVReader reader = new CSVReader(new FileReader("data.csv")); String[] line; while ((line = reader.readNext()) != null) { for (String value : line) { System.out.print(value + " "); } System.out.println(); } } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we first create a CSVReader object and pass in the path of the CSV file to be read. We then use the readNext()
method to read the data in the file line by line until we reach the end of the file.
3. Write to CSV file
To write to CSV file, we need to create a CSVWriter object and use it to write data. The following is a simple example:
import com.opencsv.CSVWriter; public class CSVWriterExample { public static void main(String[] args) { try { CSVWriter writer = new CSVWriter(new FileWriter("data.csv")); String[] line1 = {"John", "Doe", "30"}; String[] line2 = {"Jane", "Smith", "25"}; writer.writeNext(line1); writer.writeNext(line2); writer.close(); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we first create a CSVWriter object and pass in the path of the CSV file to be written. Then, we use the writeNext()
method to write a row of data. Finally, we close the CSVWriter object.
4. Using CSVReaderOptions and CSVWriterOptions
In addition to basic read and write operations, OpenCSV also provides some advanced functions, such as CSVReaderOptions and CSVWriterOptions. Using these options, we can set properties such as delimiters, quotes, etc. of the CSV file.
The following is an example of using CSVReaderOptions:
import com.opencsv.CSVReader; import com.opencsv.CSVReaderBuilder; public class CSVReaderOptionsExample { public static void main(String[] args) { try { CSVReaderBuilder builder = new CSVReaderBuilder(new FileReader("data.csv")) .withSeparator(',') .withQuoteChar('"') .withSkipLines(1); CSVReader reader = builder.build(); String[] line; while ((line = reader.readNext()) != null) { for (String value : line) { System.out.print(value + " "); } System.out.println(); } } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we first create a CSVReaderBuilder object and pass in the path of the CSV file to be read. Then, we use the withSeparator()
method to set the separator to comma, the withQuoteChar()
method to set the quotation marks to double quotes, and the withSkipLines()
method to set Ignore the first few lines of data in the file.
Similarly, we can also use CSVWriterOptions to set options for writing CSV files.
Conclusion
OpenCSV is a powerful and easy-to-use library that makes it easy to read and write CSV files. This article presents a guide to reading and writing CSV files using OpenCSV in Java. I hope this article helps you get better at working with CSV data.
The above is the detailed content of Detailed explanation of reading and writing CSV files in Java using OpenCSV. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


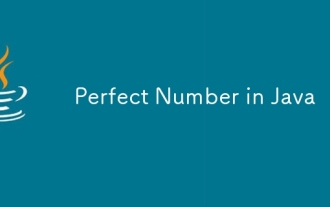
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
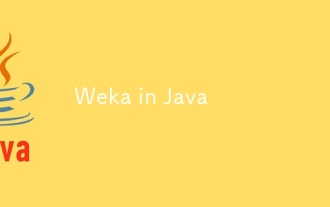
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
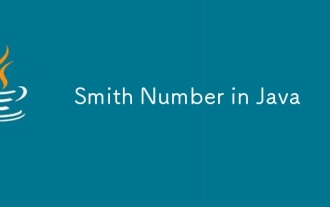
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
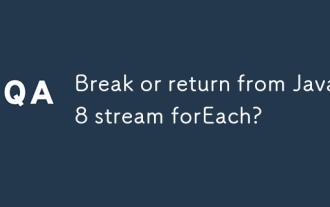
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
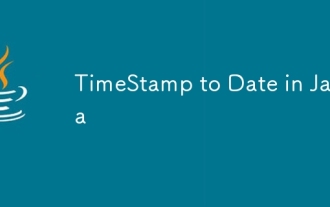
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
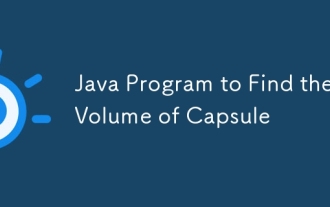
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
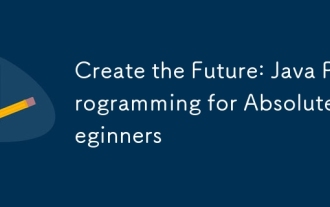
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
