


How to use the setAttribute() function in javascript and its compatibility_javascript skills
May 16, 2016 pm 03:49 PMThe setAttribute() function can set the attribute of the object. If this attribute does not exist, it will be created.
Grammar structure:
el.setAttribute(name,value)
Parameter list:
Parameter Description
name is required. Specifies the attribute name to be set.
value is required. Specifies the property value to be set.
Code example:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); mydiv.setAttribute("id","newid"); alert(mydiv.getAttribute("id")); } </script> </head> <body> <div id="mydiv"></div> </body> </html>
The above code can reset the id attribute value of the div and pop up the newly set id attribute value.
Example 2:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); mydiv.setAttribute("newAttr","attrValue"); alert(mydiv.getAttribute("newAttr")); } </script> </head> <body> <div id="mydiv"></div> </body> </html>
The above code can set the newAttr attribute value of the div and pop up this attribute value. What needs special attention here is that because div does not have the newAttr attribute by default, the setAttribute() function will first create this attribute and then assign a value to it.
The above two code examples can be successfully executed in all mainstream browsers, but this does not mean that the setAttribute() function is compatible with each browser.
Look at another code example:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <style type="text/css"> .textcolor{ font-size:18px; color:red; } </style> <script type="text/javascript"> window.onload=function(){ var mydiv=document.getElementById("mydiv"); mydiv.setAttribute("class","textcolor"); } </script> </head> <body> <div id="mydiv"></div> </body> </html>
The above code can set the font size to 18px and the font color to red in standard browsers, but it does not take effect in IE6 and IE7 browsers.
However, you can still use mydiv.getAttribute("class") to get the attribute value "textcolor".
That is to say, in IE6 or IE7 browsers, the setAttribute() function can be used, but it is not effective for all attributes.
The following attributes have the above problems:
1.class
2.for
3.cellspacing
4.cellpadding
5.tabindex
6.readonly
7.maxlength
8.rowspan
9.colspan
10.usemap
11.frameborder
12.contenteditable
13.style
In order to solve the above problem, we need to write a universal cross-browser interface method for setting element attributes:
dom=(function(){ var fixAttr={ tabindex:'tabIndex', readonly:'readOnly', 'for':'htmlFor', 'class':'className', maxlength:'maxLength', cellspacing:'cellSpacing', cellpadding:'cellPadding', rowspan:'rowSpan', colspan:'colSpan', usemap:'useMap', frameborder:'frameBorder', contenteditable:'contentEditable' }, div=document.createElement('div'); div.setAttribute('class','t'); var supportSetAttr = div.className === 't'; return { setAttr:function(el, name, val){ el.setAttribute(supportSetAttr ? name : (fixAttr[name] || name), val); }, getAttr:function(el, name){ return el.getAttribute(supportSetAttr ? name : (fixAttr[name] || name)); } } })();
First of all, standard browsers directly use the original attribute names; secondly, IE6/7 attributes that are not listed above still use the original attribute names; finally, these special attributes use fixAttr, such as class.
Then the above code example can be modified into the following form:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <style type="text/css"> .textcolor{ font-size:18px; color:red; } </style> <script type="text/javascript"> dom=(function(){ var fixAttr={ tabindex:'tabIndex', readonly:'readOnly', 'for':'htmlFor', 'class':'className', maxlength:'maxLength', cellspacing:'cellSpacing', cellpadding:'cellPadding', rowspan:'rowSpan', colspan:'colSpan', usemap:'useMap', frameborder:'frameBorder', contenteditable:'contentEditable' }, div=document.createElement('div'); div.setAttribute('class','t'); var supportSetAttr = div.className === 't'; return { setAttr:function(el, name, val){ el.setAttribute(supportSetAttr ? name : (fixAttr[name] || name), val); }, getAttr:function(el, name){ return el.getAttribute(supportSetAttr ? name : (fixAttr[name] || name)); } } })(); window.onload=function(){ var mydiv=document.getElementById("mydiv"); dom.setAttr(mydiv, 'class', 'textcolor'); } </script> </head> <body> </body> </html>
The above code is valid in all major browsers, and can set the font size to 18px and the color to red.
As for the style attribute, you can use el.style.color="xxx" for compatibility.
The above is the entire content of this article, I hope you all like it.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
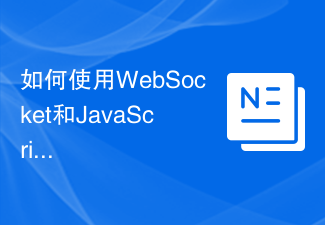
How to implement an online speech recognition system using WebSocket and JavaScript
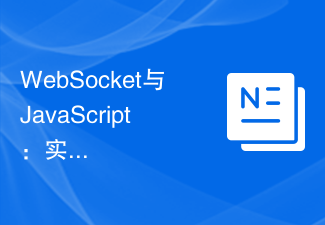
WebSocket and JavaScript: key technologies for implementing real-time monitoring systems
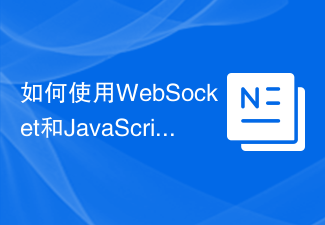
How to implement an online reservation system using WebSocket and JavaScript
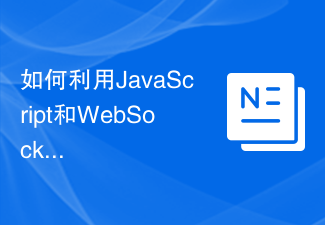
How to use JavaScript and WebSocket to implement a real-time online ordering system
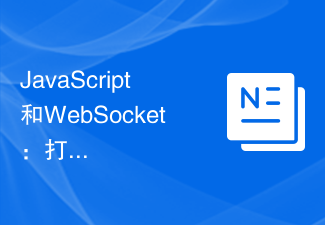
JavaScript and WebSocket: Building an efficient real-time weather forecasting system
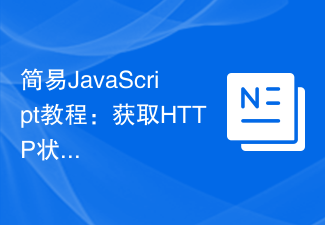
Simple JavaScript Tutorial: How to Get HTTP Status Code
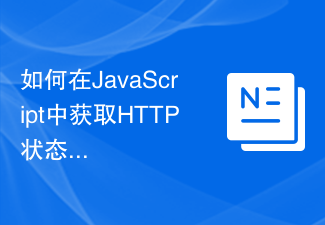
How to get HTTP status code in JavaScript the easy way

How to use insertBefore in javascript
