Basic principles and calling methods of Java reflection
Basic principles and calling methods of Java reflection
Foreword:
Java reflection is an important feature in the Java language, which allows the program to dynamically Obtain class information and operate class members. Through reflection, we can dynamically create objects, call methods, get/set properties, etc. at runtime, which greatly improves the flexibility and scalability of the program. This article will introduce the basic principles of Java reflection and give specific code examples.
1. Basic principles of reflection
The implementation of Java reflection is based on the Class class, which is the core class of Java reflection. Each Java class will generate a corresponding Class object after compilation. Class information can be obtained through the Class object, and class operations can be performed through the class information.
Java reflection mainly has the following core classes and interfaces:
- Class class: represents the type of class, which is the entry point of Java reflection.
- Field class: represents the member variables of the class.
- Method class: represents the member method of the class.
- Constructor class: Represents the constructor method of the class.
The basic principle of Java reflection is as follows: first, obtain the corresponding Class object through the fully qualified name of the class or the getClass() method of the object; then, through some methods of the Class class, such as getFields() , getMethods(), etc., to obtain Field, Method, Constructor and other objects; finally, perform specific operations through these objects, such as getting/setting attribute values, calling methods, creating objects, etc.
2. Reflection calling method
2.1 Obtain Class object
The corresponding Class object can be obtained through the fully qualified name of the class or the getClass() method of the object.
The sample code is as follows:
// 通过类的全限定名获取Class对象 Class<?> clazz1 = Class.forName("com.example.User"); // 通过对象的getClass()方法获取Class对象 User user = new User(); Class<?> clazz2 = user.getClass();
2.2 Obtaining the attribute value
The attribute value of the object can be obtained through the get() method of the Field class.
The sample code is as follows:
// 获取public属性值 Field field = clazz.getDeclaredField("name"); String name = (String) field.get(user); // 获取private属性值 Field privateField = clazz.getDeclaredField("age"); privateField.setAccessible(true); // 设置private属性的访问权限 int age = (int) privateField.get(user);
2.3 Setting the attribute value
The attribute value of the object can be set through the set() method of the Field class.
The sample code is as follows:
// 设置public属性值 Field field = clazz.getDeclaredField("name"); field.set(user, "Tom"); // 设置private属性值 Field privateField = clazz.getDeclaredField("age"); privateField.setAccessible(true); // 设置private属性的访问权限 privateField.set(user, 20);
2.4 Calling the method
The method of the object can be called through the invoke() method of the Method class.
The sample code is as follows:
// 调用无参方法 Method method1 = clazz.getDeclaredMethod("sayHello"); method1.invoke(user); // 调用带参方法 Method method2 = clazz.getDeclaredMethod("sayHi", String.class); method2.invoke(user, "Jack");
2.5 Creating objects
Objects can be created through the newInstance() method of the Constructor class.
The sample code is as follows:
Constructor<?> constructor = clazz.getDeclaredConstructor(String.class, int.class); User newUser = (User) constructor.newInstance("Lucy", 25);
Summary:
Java reflection is a powerful feature in the Java language, which can dynamically obtain class information and operate class members at runtime . The basic principle of reflection is to obtain class information through the Class class and perform specific operations through Field, Method, Constructor and other objects. In actual applications, we can use the reflection mechanism to realize various dynamic requirements and improve the flexibility and scalability of the program.
The above is the detailed content of Basic principles and calling methods of Java reflection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


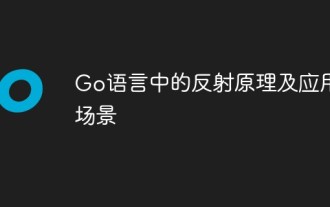
The Go language comes with a reflection mechanism, which is one of its biggest features. Reflection provides the Go language with a way to check variables and call methods at runtime, which allows us to understand and manipulate data in the program in a common, unified way without caring about the type of specific data. This is programming A common problem in language. In this article, we will delve into the reflection principles and application scenarios in the Go language. What is reflection? In the computer field, reflection refers to dynamically detecting the type of data or operating on data at runtime.
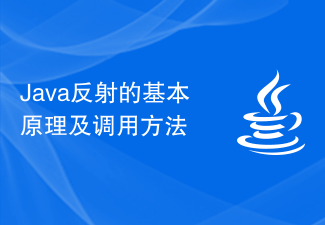
Basic principles of Java reflection and calling methods Preface: Java reflection is an important feature of the Java language, which allows programs to dynamically obtain class information and operate class members at runtime. Through reflection, we can dynamically create objects, call methods, get/set properties, etc. at runtime, which greatly improves the flexibility and scalability of the program. This article will introduce the basic principles of Java reflection and give specific code examples. 1. The basic principles of reflection. The implementation of Java reflection is based on the Class class. Class
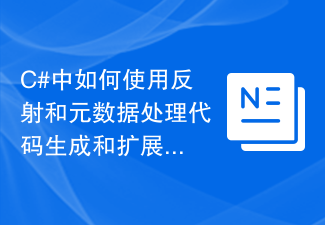
How to use reflection and metadata to handle code generation and extension and solutions in C#, specific code examples are required Title: Methods and solutions for using reflection and metadata to generate and extend code in C# Introduction: Reflection and metadata in C# development It is a very powerful tool that can help us achieve the function of dynamically generating and expanding code. This article describes how to use reflection and metadata to handle code generation and extension, and provides specific code examples. 1. Use reflection to generate code. Through reflection, we can dynamically load and check at runtime.
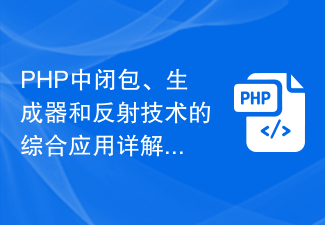
Detailed explanation of the comprehensive application of closures, generators and reflection technologies in PHP Introduction: As the complexity of web applications continues to increase, developers need more advanced and flexible technologies to deal with these challenges. PHP is a popular server-side scripting language that offers many powerful features, including closures, generators, and reflection. This article will introduce the comprehensive application of these technologies in detail and provide specific code examples. 1. Closure: Closure refers to a function defined inside a function and can access its outside
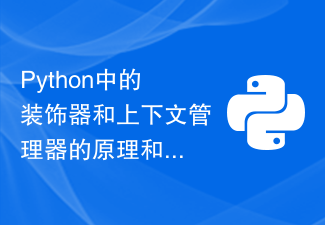
Decorators and context managers in Python are two very useful features that can help us better organize and manage code and improve code reusability. This article will introduce the principles and usage scenarios of decorators and context managers respectively, and give specific code examples. 1. Principles and usage scenarios of decorators: Decorators are a way to add additional functions to a function without changing the definition of the original function. It is actually a function that accepts the decorated function as input and returns the wrapped function. decorate
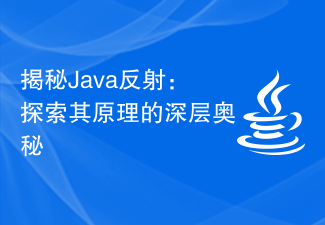
Decrypting Java Reflection: To explore the principles behind it, specific code examples are required. Introduction: In Java programming, reflection (Reflection) is a powerful and flexible mechanism that allows us to dynamically inspect classes, interfaces, fields and methods at runtime. , they can be called and manipulated even without knowing the concrete class. This article will delve into the principles behind Java reflection and provide specific code examples to help readers better understand and apply reflection. What is reflection? In short, reflection is a method of obtaining and manipulating
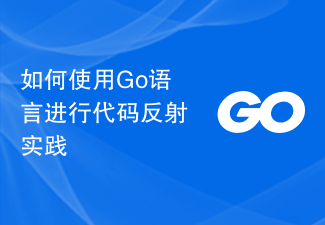
How to use Go language for code reflection practice introduction: In Go language, reflection is a powerful mechanism that allows us to dynamically check type information and manipulate objects while the program is running. Through reflection, we can call methods, access fields, create instances, etc. without knowing the specific type. This article will introduce how to use the reflection mechanism of Go language for code practice and give corresponding code examples. Reflection Basics Reflection refers to the ability to inspect variables, categories, methods, and interfaces when a program is running. In Go language, reflection is
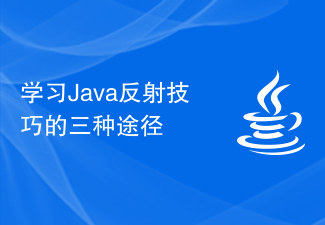
Learn more about the three techniques of Java reflection Introduction: Java reflection is a powerful programming technique that can dynamically obtain class-related information and operate class members at runtime. This article will introduce three advanced techniques of Java reflection and give specific code examples. Obtaining complete information of a class Through Java reflection, we can obtain complete information of a class, including class name, parent class, implemented interfaces, methods, fields, etc. Here is a sample code to get complete information about a class: importjava.lang
