Golang data conversion skills and examples sharing
Golang’s data conversion skills and examples are shared
Introduction:
Golang is a powerful programming language that handles various data types and format conversions It provides many convenient functions and techniques. This article will share some practical tips for data conversion in Golang and provide specific code examples.
1. Conversion between strings and integers
In Golang, conversion between strings and integers is a frequently encountered requirement. Golang provides the strconv package, whose Atoi() and Itoa() functions can easily convert strings and integers to and from each other.
- Convert string to integer
Sample code:
package main import ( "fmt" "strconv" ) func main() { str := "123" num, err := strconv.Atoi(str) if err != nil { fmt.Println("转换失败:", err) return } fmt.Println("转换后的整数:", num) }
Run result:
Converted integer: 123
- Integer to string
Sample code:
package main import ( "fmt" "strconv" ) func main() { num := 123 str := strconv.Itoa(num) fmt.Println("转换后的字符串:", str) }
Running result:
Converted string: 123
2. Conversion between strings and floating point numbers
In addition to conversion between strings and integers, Golang also provides conversion functions between strings and floating point numbers. This is a very useful function in price calculations or other scenarios that require precise calculations.
- Convert string to floating point number
Sample code:
package main import ( "fmt" "strconv" ) func main() { str := "3.14" num, err := strconv.ParseFloat(str, 64) if err != nil { fmt.Println("转换失败:", err) return } fmt.Println("转换后的浮点数:", num) }
Running result:
Converted floating point number: 3.14
- Floating point number to string
Sample code:
package main import ( "fmt" "strconv" ) func main() { num := 3.14 str := strconv.FormatFloat(num, 'f', -1, 64) fmt.Println("转换后的字符串:", str) }
Running result:
Converted string: 3.14
3. Between slices and strings Conversion
Conversion between slices and strings is also one of the common operations in Golang. We can convert a string into a slice and modify it; or convert a slice into a string as output or storage content.
- Convert string to slice
Sample code:
package main import ( "fmt" ) func main() { str := "hello" slice := []byte(str) slice[0] = 'H' fmt.Println("转换后的切片:", slice) }
Run result:
Converted slice: [72 101 108 108 111]
- Slice to string
Sample code:
package main import ( "fmt" ) func main() { slice := []byte{'H', 'e', 'l', 'l', 'o'} str := string(slice) fmt.Println("转换后的字符串:", str) }
Run result:
Converted string: Hello
IV. Other data types Conversion between
In addition to conversion between strings, integers and floating point numbers, Golang also provides conversion functions between some other data types, such as conversion between bool and string, time and string conversion, etc.
- Conversion between bool and string
Sample code:
package main import ( "fmt" "strconv" ) func main() { b := true str := strconv.FormatBool(b) fmt.Println("转换后的字符串:", str) str2 := "true" bool, err := strconv.ParseBool(str2) if err != nil { fmt.Println("转换失败:", err) return } fmt.Println("转换后的bool:", bool) }
Run result:
Converted string: true
Conversion The resulting bool: true
- Conversion between time and string
Sample code:
package main import ( "fmt" "time" ) func main() { now := time.Now() str := now.Format("2006-01-02 15:04:05") fmt.Println("转换后的字符串:", str) str2 := "2022-01-01 12:00:00" time, err := time.Parse("2006-01-02 15:04:05", str2) if err != nil { fmt.Println("转换失败:", err) return } fmt.Println("转换后的时间:", time) }
Run result:
Converted string: 2022-06-20 09:54:36
Time after conversion: 2022-01-01 12:00:00 0000 UTC
Summary:
This article introduces the method of data conversion in Golang Some practical tips and concrete code examples are provided. Through these techniques, we can easily convert between different data types and improve the flexibility and reusability of the code. I hope readers can use these techniques in actual development to improve work efficiency.
The above is the detailed content of Golang data conversion skills and examples sharing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


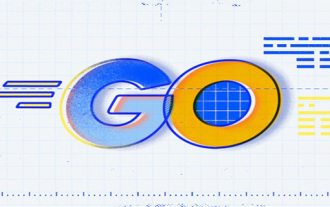
Conversion method: 1. Use the Atoi() function in the strconv package to convert the string type integer to the int type, with the syntax "strconv.Atoi(string)"; 2. Use the ParseInt() function in the strconv package to convert Returns an integer value represented by a string (accepts sign), syntax "strconv.ParseInt(string,10,64)".
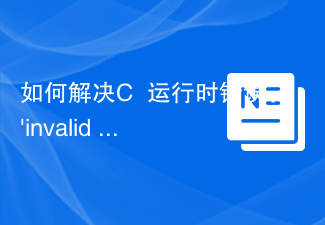
How to solve C++ runtime error: 'invalidtypeconversion'? During the C++ programming process, we often encounter various compile-time and run-time errors. One of the common runtime errors is the 'invalidtypeconversion' error. This error is triggered when we convert one data type to another incompatible data type. This article will introduce some common causes of this error and how to solve it.
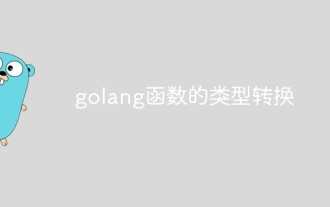
In-function type conversion allows data of one type to be converted to another type, thereby extending the functionality of the function. Use syntax: type_name:=variable.(type). For example, you can use the strconv.Atoi function to convert a string to a number and handle errors if the conversion fails.
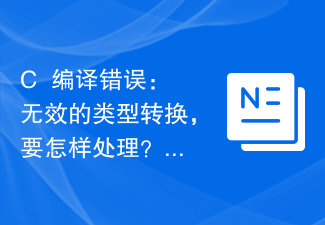
As a strongly typed language, C++ requires special attention when converting data types, otherwise the compiler will report an error. One of the more common errors is "invalid type conversion". This article will explain why this error occurs, how to perform type conversion, and how to avoid this error. 1. Cause of the error: Data type mismatch. There are some data types in C++ that cannot be converted directly. For example, you cannot convert a character variable directly to an integer variable, or a floating-point variable directly to a Boolean variable.
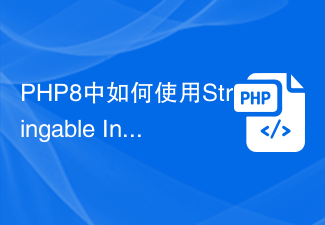
How to use StringableInterface to handle string operations more conveniently in PHP8? PHP8 is the latest version of the PHP language and brings many new features and improvements. One of the improvements that developers are excited about is the addition of StringableInterface. StringableInterface is an interface for handling string operations, which provides a more convenient way to handle and operate strings. This article will detail how to use
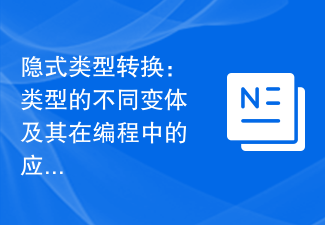
Explore the different types of implicit type conversions and their role in programming Introduction: In programming, we often need to deal with different types of data. Sometimes, we need to convert one data type to another type in order to perform a specific operation or meet specific requirements. In this process, implicit type conversion is a very important concept. Implicit type conversion refers to the process in which the programming language automatically performs data type conversion without explicitly specifying the conversion type. This article will explore the different types of implicit type conversions and their role in programming,
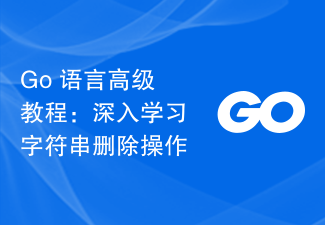
Go language is a very popular programming language, and its powerful features make it favored by many developers. String operations are one of the most common operations in programming, and in the Go language, string deletion operations are also very common. This article will delve into the string deletion operation in the Go language and use specific code examples to help you better understand and master this knowledge point. String deletion operation In the Go language, we usually use the strings package to perform string operations, including deletion operations.
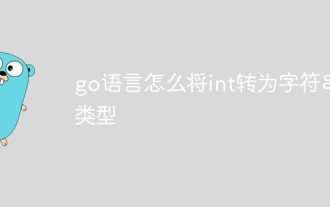
Conversion method: 1. Use the Itoa() function, the syntax "strconv.Itoa(num)"; 2. Use the FormatInt() function to convert int type data into the specified base and return it in the form of a string, the syntax "strconv .FormatInt(num,10)".
