


An in-depth analysis of Java reflection: exploring the internal implementation of the reflection mechanism
Exploring the principles of Java reflection: In-depth understanding of the underlying implementation of reflection requires specific code examples
In Java development, reflection is an important and powerful function. It allows the program to obtain and operate information such as classes, methods, properties, etc. at runtime, making the program more dynamic and flexible. However, the principles and underlying implementation of reflection are not well known. This article will delve into the principles of Java reflection and deepen your understanding through specific code examples.
First of all, we need to understand Java’s class loading mechanism. In Java, class loading is done through ClassLoader. ClassLoader loads bytecode files (.class files) into the JVM according to certain rules and converts them into usable Class objects. Java reflection is implemented through this Class object.
Code example 1: Load the class through the Class.forName() method
Class<?> clazz = Class.forName("com.example.TestClass");
Through the above code, we can get the Class object of the "com.example.TestClass" class. The Class object represents the information of this class in the JVM.
Next, we need to understand what information is saved in the Class object. The Class object stores a lot of important information, including the name, methods, attributes, etc. of the class. Among them, two commonly used methods are getDeclaredMethods() and getDeclaredFields(), which return detailed information about the methods and properties defined in the class respectively.
Code example 2: Get the method and attribute information in the class through the Class object
Class<?> clazz = Class.forName("com.example.TestClass"); // 获取类中定义的方法信息 Method[] methods = clazz.getDeclaredMethods(); for (Method method : methods) { System.out.println("Method name: " + method.getName()); } // 获取类中定义的属性信息 Field[] fields = clazz.getDeclaredFields(); for (Field field : fields) { System.out.println("Field name: " + field.getName()); }
Through the above code, we can get the detailed information of all methods and attributes defined in the TestClass class, and printed on the console.
Next, we need to understand how reflection creates objects, calls methods and accesses properties. In reflection, we use Constructor class, Method class and Field class.
Code example 3: Create objects, call methods and access properties through reflection
Class<?> clazz = Class.forName("com.example.TestClass"); // 创建对象 Constructor> constructor = clazz.getDeclaredConstructor(); constructor.setAccessible(true); Object obj = constructor.newInstance(); // 调用方法 Method method = clazz.getDeclaredMethod("testMethod", String.class); method.setAccessible(true); method.invoke(obj, "Hello, Reflection!"); // 访问属性 Field field = clazz.getDeclaredField("testField"); field.setAccessible(true); field.set(obj, "Reflection Test"); String value = (String) field.get(obj); System.out.println("Field value: " + value);
Through the above code, we can use reflection to create objects of the TestClass class, call the testMethod method and pass in parameters, Then access the testField property and get its value.
So far, we have an in-depth understanding of the principles and underlying implementation of Java reflection. Reflection allows us to obtain and manipulate class information at runtime, making the program more flexible and scalable. By understanding ClassLoader, Class object, Constructor class, Method class and Field class, we can better apply reflection to solve practical problems.
It should be noted that although reflection is powerful, overuse of reflection may cause performance degradation. Therefore, it should be used with caution in actual development and other more effective solutions should be given priority.
To summarize, this article hopes to help readers better understand and apply the reflection function by in-depth discussion of the principles and underlying implementation of Java reflection, combined with specific code examples.
The above is the detailed content of An in-depth analysis of Java reflection: exploring the internal implementation of the reflection mechanism. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


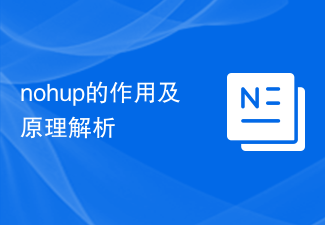
Analysis of the role and principle of nohup In Unix and Unix-like operating systems, nohup is a commonly used command that is used to run commands in the background. Even if the user exits the current session or closes the terminal window, the command can still continue to be executed. In this article, we will analyze the function and principle of the nohup command in detail. 1. The role of nohup: Running commands in the background: Through the nohup command, we can let long-running commands continue to execute in the background without being affected by the user exiting the terminal session. This needs to be run
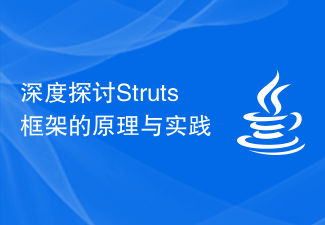
Principle analysis and practical exploration of the Struts framework. As a commonly used MVC framework in JavaWeb development, the Struts framework has good design patterns and scalability and is widely used in enterprise-level application development. This article will analyze the principles of the Struts framework and explore it with actual code examples to help readers better understand and apply the framework. 1. Analysis of the principles of the Struts framework 1. MVC architecture The Struts framework is based on MVC (Model-View-Con
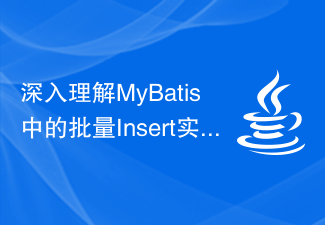
MyBatis is a popular Java persistence layer framework that is widely used in various Java projects. Among them, batch insertion is a common operation that can effectively improve the performance of database operations. This article will deeply explore the implementation principle of batch Insert in MyBatis, and analyze it in detail with specific code examples. Batch Insert in MyBatis In MyBatis, batch Insert operations are usually implemented using dynamic SQL. By constructing a line S containing multiple inserted values

The RPM (RedHatPackageManager) tool in Linux systems is a powerful tool for installing, upgrading, uninstalling and managing system software packages. It is a commonly used software package management tool in RedHatLinux systems and is also used by many other Linux distributions. The role of the RPM tool is very important. It allows system administrators and users to easily manage software packages on the system. Through RPM, users can easily install new software packages and upgrade existing software
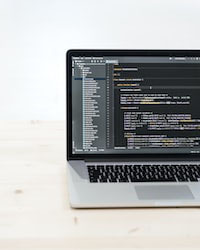
Java reflection is a powerful tool that allows you to access the private fields and methods of a class, thereby revealing the inner workings of the software. This is useful in areas such as reverse engineering, software analysis and debugging. To use Java reflection, you first need to import the java.lang.reflect package. Then, you can use the Class.forName() method to obtain the Class object of a class. Once you have a Class object, you can use various methods to access the fields and methods of the class. For example, you can use the getDeclaredFields() method to get all fields of a class, including private fields. You can also use the getDeclaredMethods() method
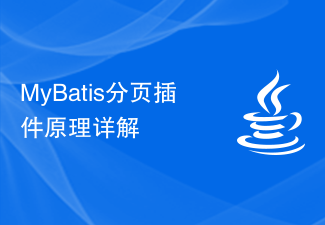
MyBatis is an excellent persistence layer framework. It supports database operations based on XML and annotations. It is simple and easy to use. It also provides a rich plug-in mechanism. Among them, the paging plug-in is one of the more frequently used plug-ins. This article will delve into the principles of the MyBatis paging plug-in and illustrate it with specific code examples. 1. Paging plug-in principle MyBatis itself does not provide native paging function, but you can use plug-ins to implement paging queries. The principle of paging plug-in is mainly to intercept MyBatis
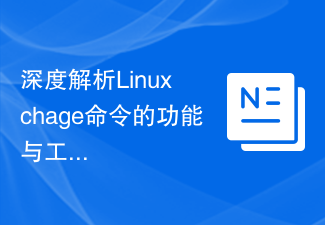
The chage command in the Linux system is a command used to modify the password expiration date of a user account. It can also be used to modify the longest and shortest usable date of the account. This command plays a very important role in managing user account security. It can effectively control the usage period of user passwords and enhance system security. How to use the chage command: The basic syntax of the chage command is: chage [option] user name. For example, to modify the password expiration date of user "testuser", you can use the following command
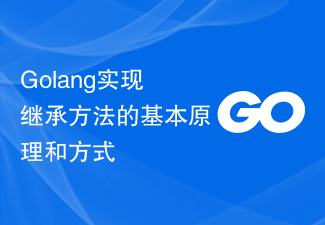
The basic principles and implementation methods of Golang inheritance methods In Golang, inheritance is one of the important features of object-oriented programming. Through inheritance, we can use the properties and methods of the parent class to achieve code reuse and extensibility. This article will introduce the basic principles and implementation methods of Golang inheritance methods, and provide specific code examples. The basic principle of inheritance methods In Golang, inheritance is implemented by embedding structures. When a structure is embedded in another structure, the embedded structure has embedded
