Explore the benefits and use cases of Java workflows
Advantages and application scenarios of Java workflow
With the rapid development of information technology, workflow management systems are increasingly used in enterprises. As a mature, stable and flexible process engine, Java workflow has many advantages and can be applied to various scenarios. This article will introduce the advantages of Java workflow and illustrate its application scenarios through specific code examples.
- Advantages
1.1 Stable and reliable
The Java workflow engine is based on mature technology frameworks, such as Spring and Hibernate, and has good stability and reliability. It can handle high concurrency and large amounts of data scenarios, and can ensure the correct execution of the process.
1.2 Flexible and scalable
The Java workflow engine adopts a modular design and can be flexibly configured and expanded according to different business needs. It provides rich APIs and interfaces, and supports custom process models, task processing, event monitoring and other functions.
1.3 Visual Management
Java workflow engine usually provides a visual process designer and management interface, allowing business personnel to easily design, adjust and manage workflows. This greatly improves efficiency and reduces developer workload.
1.4 Multi-platform support
The Java workflow engine supports cross-platform features and can run on different operating systems and servers, such as Windows, Linux, Unix, etc. This allows enterprises to choose the appropriate platform for deployment based on their needs.
- Application Scenario
2.1 Approval Process
The approval process is one of the most common workflow scenarios in enterprises. Java workflow engine can help enterprises design and manage approval processes, such as leave, reimbursement, contract approval, etc. The following is a code example of a simple leave approval process:
public class LeaveProcess { public static void main(String[] args) { // 创建工作流引擎 WorkflowEngine engine = new WorkflowEngine(); // 注册流程节点 engine.registerNode("Manager", new ManagerNode()); engine.registerNode("HR", new HRNode()); engine.registerNode("CEO", new CEONode()); // 创建请假流程 Workflow workflow = new Workflow(); workflow.addNode("Manager"); workflow.addNode("HR"); workflow.addNode("CEO"); // 开始流程 engine.startWorkflow(workflow); } } public class ManagerNode implements Node { public void execute(Context context) { System.out.println("部门经理审批通过"); // 更新上下文状态 context.set("managerApproved", true); } } public class HRNode implements Node { public void execute(Context context) { // 获取上下文状态 boolean managerApproved = context.get("managerApproved"); if (managerApproved) { System.out.println("人事部审批通过"); // 更新上下文状态 context.set("hrApproved", true); } else { System.out.println("人事部审批不通过"); // 更新上下文状态 context.set("hrApproved", false); } } } public class CEONode implements Node { public void execute(Context context) { // 获取上下文状态 boolean hrApproved = context.get("hrApproved"); if (hrApproved) { System.out.println("CEO审批通过"); } else { System.out.println("CEO审批不通过"); } } }
2.2 Order processing
Order processing is a common workflow scenario in e-commerce systems. Java workflow engine can help enterprises design and manage order processing processes, such as order creation, payment, shipping, etc. The following is a code example of a simple order processing process:
public class OrderProcess { public static void main(String[] args) { // 创建工作流引擎 WorkflowEngine engine = new WorkflowEngine(); // 注册流程节点 engine.registerNode("CreateOrder", new CreateOrderNode()); engine.registerNode("PayOrder", new PayOrderNode()); engine.registerNode("DeliverOrder", new DeliverOrderNode()); // 创建订单处理流程 Workflow workflow = new Workflow(); workflow.addNode("CreateOrder"); workflow.addNode("PayOrder"); workflow.addNode("DeliverOrder"); // 开始流程 engine.startWorkflow(workflow); } } public class CreateOrderNode implements Node { public void execute(Context context) { System.out.println("订单创建成功"); // 更新上下文状态 context.set("orderCreated", true); } } public class PayOrderNode implements Node { public void execute(Context context) { // 获取上下文状态 boolean orderCreated = context.get("orderCreated"); if (orderCreated) { System.out.println("订单支付成功"); // 更新上下文状态 context.set("orderPaid", true); } else { System.out.println("订单支付失败"); // 更新上下文状态 context.set("orderPaid", false); } } } public class DeliverOrderNode implements Node { public void execute(Context context) { // 获取上下文状态 boolean orderPaid = context.get("orderPaid"); if (orderPaid) { System.out.println("订单发货成功"); } else { System.out.println("订单发货失败"); } } }
Summary:
The Java workflow engine has the advantages of stability, reliability, flexibility and scalability, visual management, and multi-platform support. It can be applied to various scenarios such as approval process and order processing. Through the above code examples, you can better understand and apply the Java workflow engine.
The above is the detailed content of Explore the benefits and use cases of Java workflows. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
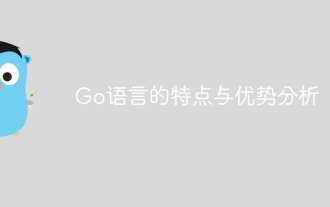
Features of Go language: High concurrency (goroutine) Automatic garbage collection Cross-platform simplicity Modularity Advantages of Go language: High performance Security Scalability Community support
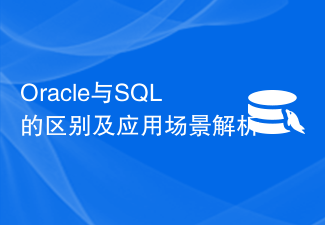
The difference between Oracle and SQL and analysis of application scenarios In the database field, Oracle and SQL are two frequently mentioned terms. Oracle is a relational database management system (RDBMS), and SQL (StructuredQueryLanguage) is a standardized language for managing relational databases. While they are somewhat related, there are also some significant differences. First of all, by definition, Oracle is a specific database management system, consisting of

Golang's single-threaded features and advantages With the booming development of the Internet and mobile applications, the demand for high-performance, high-concurrency programming languages is increasing. Against this background, the Go language (Golang for short) was developed by Google and first released in 2009, and quickly became popular among developers. Golang is an open source programming language that uses static typing and concurrent design. One of its biggest advantages is its single-threaded feature. Golang adopts Goroutine’s concurrency model.
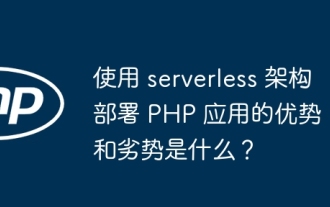
Deploying PHP applications using Serverless architecture has the following advantages: maintenance-free, pay-as-you-go, highly scalable, simplified development and support for multiple services. Disadvantages include: cold start time, debugging difficulties, vendor lock-in, feature limitations, and cost optimization challenges.
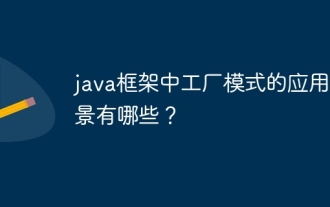
The factory pattern is used to decouple the creation process of objects and encapsulate them in factory classes to decouple them from concrete classes. In the Java framework, the factory pattern is used to: Create complex objects (such as beans in Spring) Provide object isolation, enhance testability and maintainability Support extensions, increase support for new object types by adding new factory classes
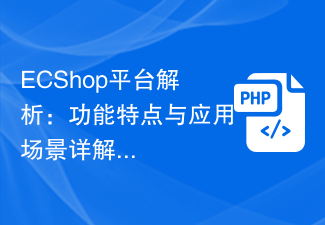
ECShop platform analysis: Detailed explanation of functional features and application scenarios ECShop is an open source e-commerce system developed based on PHP+MySQL. It has powerful functional features and a wide range of application scenarios. This article will analyze the functional features of the ECShop platform in detail, and combine it with specific code examples to explore its application in different scenarios. Features 1.1 Lightweight and high-performance ECShop adopts a lightweight architecture design, with streamlined and efficient code and fast running speed, making it suitable for small and medium-sized e-commerce websites. It adopts the MVC pattern

The Go language is suitable for a variety of scenarios, including back-end development, microservice architecture, cloud computing, big data processing, machine learning, and building RESTful APIs. Among them, the simple steps to build a RESTful API using Go include: setting up the router, defining the processing function, obtaining the data and encoding it into JSON, and writing the response.
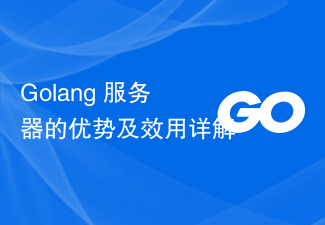
Golang is an open source programming language developed by Google. It is efficient, fast and powerful and is widely used in cloud computing, network programming, big data processing and other fields. As a strongly typed, static language, Golang has many advantages when building server-side applications. This article will analyze the advantages and utility of Golang server in detail, and illustrate its power through specific code examples. 1. The high-performance Golang compiler can compile the code into local code
