


Learn Golang in depth: Flexible application of timestamp acquisition techniques
Golang Advanced: Master the acquisition and application of timestamps
Timestamps are a way of expressing time in computer systems, based on a specific Time is the starting point, the number of seconds or milliseconds from a time point in the past or future to the starting point. In development, timestamp is very important. It is often used to record the time when an event occurs and perform time comparison and calculation. This article will introduce how to obtain timestamps in Golang, and demonstrate various applications of timestamps through specific code examples.
1. Get the current timestamp
In Golang, you can easily get the current timestamp through the functions provided by the time package. The following is a sample code:
package main import ( "fmt" "time" ) func main() { timestamp := time.Now().Unix() fmt.Println("当前时间戳:", timestamp) }
Running the above code will output the current timestamp, and the result will be similar to: current timestamp: 1605721708.
2. Timestamp formatting
The default timestamp is an integer in seconds, which is not very intuitive. For better readability, we can format the timestamp to a specified datetime format. The time package in Golang provides the Format function to implement formatting operations. The following is a sample code:
package main import ( "fmt" "time" ) func main() { timestamp := time.Now().Unix() fmt.Println("当前时间戳:", timestamp) tm := time.Unix(timestamp, 0) fmt.Println("格式化时间:", tm.Format("2006-01-02 15:04:05")) }
Run the above code, in addition to outputting the current timestamp, the formatted time is also output, for example: formatted time: 2020-11-19 08:08:24.
It is worth noting that the formatting of time in Golang adopts a special format, such as "2006-01-02 15:04:05" in the above example, where the numbers are fixed, respectively. Represents year, month, day, hour, minute and second. This is to commemorate the time when Golang was born.
3. Convert timestamp to time type
Sometimes we need to convert timestamp to time type in Golang to facilitate time operations. The time package in Golang provides Unix and UnixNano functions to convert timestamps to time types. The following is a sample code:
package main import ( "fmt" "time" ) func main() { timestamp := time.Now().Unix() fmt.Println("当前时间戳:", timestamp) tm := time.Unix(timestamp, 0) fmt.Println("时间类型:", tm) }
Run the above code, in addition to outputting the current timestamp, it also outputs the result converted to a time type, for example: Time type: 2020-11-19 08:08:24 0800 CST.
4. Calculation of timestamps
In addition to obtaining and formatting timestamps, addition and subtraction operations can also be performed to achieve time calculation. The time type in Golang provides the Add method and Sub method to perform time addition and subtraction operations. The following is a sample code:
package main import ( "fmt" "time" ) func main() { tm := time.Now() fmt.Println("当前时间:", tm) oneHourLater := tm.Add(time.Hour) fmt.Println("一小时后的时间:", oneHourLater) threeDaysAgo := tm.AddDate(0, 0, -3) fmt.Println("三天前的时间:", threeDaysAgo) duration := oneHourLater.Sub(threeDaysAgo) fmt.Println("时间间隔:", duration) }
Run the above code, in addition to outputting the current time, it also outputs the results after addition and subtraction, for example: time one hour later: 2020-11-19 09:08: 24.0103487 0800 CST, time three days ago: 2020-11-16 08:08:24.0103487 0800 CST, time interval: 24h0m0s.
Through the above code example, we can see how to use the Add and Sub methods to add and subtract time, and get the result of the time interval.
Summary
Time stamps are very important in development and can be used to record the occurrence time of events, compare times, calculate time, etc. This article uses specific code examples to introduce how to obtain timestamps in Golang, as well as timestamp formatting, conversion and calculation applications. I hope it will be helpful to readers and improve their understanding of timestamps in Golang.
The above is the detailed content of Learn Golang in depth: Flexible application of timestamp acquisition techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


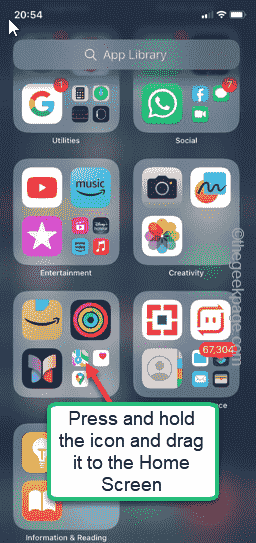
Deleted something important from your home screen and trying to get it back? You can put app icons back on the screen in a variety of ways. We have discussed all the methods you can follow and put the app icon back on the home screen. How to Undo Remove from Home Screen in iPhone As we mentioned before, there are several ways to restore this change on iPhone. Method 1 – Replace App Icon in App Library You can place an app icon on your home screen directly from the App Library. Step 1 – Swipe sideways to find all apps in the app library. Step 2 – Find the app icon you deleted earlier. Step 3 – Simply drag the app icon from the main library to the correct location on the home screen. This is the application diagram
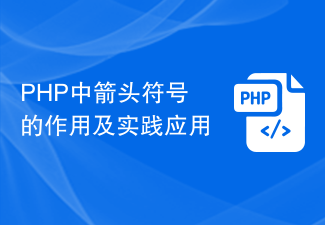
The role and practical application of arrow symbols in PHP In PHP, the arrow symbol (->) is usually used to access the properties and methods of objects. Objects are one of the basic concepts of object-oriented programming (OOP) in PHP. In actual development, arrow symbols play an important role in operating objects. This article will introduce the role and practical application of arrow symbols, and provide specific code examples to help readers better understand. 1. The role of the arrow symbol to access the properties of an object. The arrow symbol can be used to access the properties of an object. When we instantiate a pair
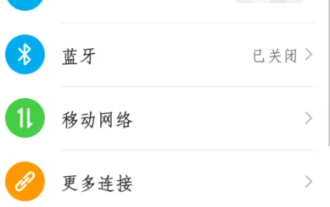
Google Authenticator is a tool used to protect the security of user accounts, and its key is important information used to generate dynamic verification codes. If you forget the key of Google Authenticator and can only verify it through the security code, then the editor of this website will bring you a detailed introduction on where to get the Google security code. I hope it can help you. If you want to know more Users please continue reading below! First open the phone settings and enter the settings page. Scroll down the page and find Google. Go to the Google page and click on Google Account. Enter the account page and click View under the verification code. Enter your password or use your fingerprint to verify your identity. Obtain a Google security code and use the security code to verify your Google identity.
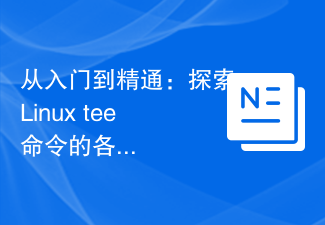
The Linuxtee command is a very useful command line tool that can write output to a file or send output to another command without affecting existing output. In this article, we will explore in depth the various application scenarios of the Linuxtee command, from entry to proficiency. 1. Basic usage First, let’s take a look at the basic usage of the tee command. The syntax of tee command is as follows: tee[OPTION]...[FILE]...This command will read data from standard input and save the data to
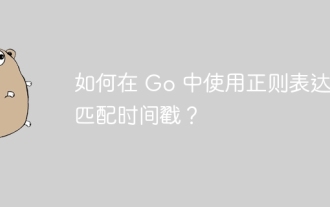
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
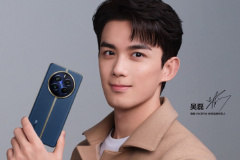
Although the general operations of domestic mobile phones are very similar, there are still some differences in some details. For example, different mobile phone models and manufacturers may have different dual-SIM installation methods. Erzhenwo 12Pro, a new mobile phone, also supports dual-SIM dual standby, but how should dual-SIM be installed on this phone? How to install dual SIM on Realme 12Pro? Remember to turn off your phone before installation. Step 1: Find the SIM card tray: Find the SIM card tray of the phone. Usually, in the Realme 12 Pro, the SIM card tray is located on the side or top of the phone. Step 2: Insert the first SIM card. Use a dedicated SIM card pin or a small object to insert it into the slot in the SIM card tray. Then, carefully insert the first SIM card.
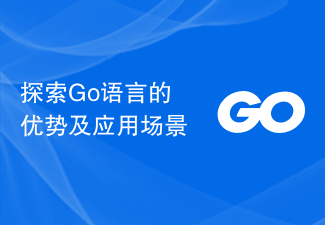
The Go language is an open source programming language developed by Google and first released in 2007. It is designed to be a simple, easy-to-learn, efficient, and highly concurrency language, and is favored by more and more developers. This article will explore the advantages of Go language, introduce some application scenarios suitable for Go language, and give specific code examples. Advantages: Strong concurrency: Go language has built-in support for lightweight threads-goroutine, which can easily implement concurrent programming. Goroutin can be started by using the go keyword
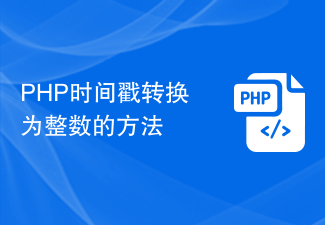
Timestamp in PHP is an integer form representing time, usually the number of seconds that have passed since the first year of Unix (January 1, 1970 00:00:00 GMT). In programming, we often need to convert timestamps into other forms of integers. Here we will introduce how to convert PHP timestamps into integers, as well as specific code examples. In PHP, we can use the strtotime() function to convert the time string to a timestamp and then use date
