


Analyze the way Golang implements inheritance and its applicable scenarios
Golang inheritance implementation method and application scenario analysis
Inheritance is one of the important concepts of object-oriented programming. It provides the ability to reuse code and extend functions. Although the Go language does not have an inheritance mechanism in the traditional sense, through structure embedding and interface implementation, we can achieve effects similar to inheritance. This article will introduce the implementation of inheritance in Golang and explore its application scenarios. The following will be divided into two parts to explain.
1. Structure nesting to achieve inheritance
In the Go language, we can achieve an effect similar to inheritance through structure nesting. That is, embedding other structures in a structure as its fields, and realizing the reuse of fields and methods in this way.
The following is a simple example:
type Animal struct { name string } func (a *Animal) SayHello() { fmt.Println("Hello, I am an animal.") } type Dog struct { Animal breed string } func main() { dog := Dog{ Animal: Animal{ name: "Bobby", }, breed: "Beagle", } dog.SayHello() // 输出:Hello, I am an animal. fmt.Println(dog.name) // 输出:Bobby fmt.Println(dog.breed) // 输出:Beagle }
In the above example, we defined an Animal structure and added a SayHello method to it. Then we defined a Dog structure, and by embedding the Animal structure in the Dog structure, we realized the reuse of the Animal structure fields and methods. In this way, the Dog structure has the properties and methods of Animal.
There are many application scenarios for nested structures to implement inheritance. For example, when we need to define multiple structures with common characteristics, we can abstract these characteristics into a basic structure, and then use nested method is used in other structures. This reduces code duplication and allows for easy expansion and modification.
2. Implement polymorphism through interfaces
Another way to implement inheritance is to implement polymorphism through interfaces. In the Go language, an interface is a collection of methods. As long as these methods are implemented, they are considered to be the implementation type of the interface. Through the implementation of interfaces, we can achieve the purpose of uniformly calling methods of different structure objects and achieve polymorphic effects.
The following is a simple example:
type Animal interface { SayHello() } type Dog struct { name string } func (d *Dog) SayHello() { fmt.Println("Hello, I am a dog.") } type Cat struct { name string } func (c *Cat) SayHello() { fmt.Println("Hello, I am a cat.") } func main() { var animal Animal animal = &Dog{ name: "Bobby", } animal.SayHello() // 输出:Hello, I am a dog. animal = &Cat{ name: "Tom", } animal.SayHello() // 输出:Hello, I am a cat. }
In the above example, we define an Animal interface and define a SayHello method in the interface. Then we defined the Dog and Cat structures respectively and implemented the SayHello method for them. In the main function, we can see that through the implementation of the interface, we can assign Dog and Cat objects to animal variables and call their SayHello methods.
The application scenarios of interface polymorphism are very wide. For example, when dealing with some abstract business logic, we can use interfaces to define unified methods, and then implement the business logic in specific implementations. This enhances the scalability and maintainability of your code.
Summary:
Although there is no traditional inheritance mechanism in the Go language, through structure nesting and interface implementation, we can achieve effects similar to inheritance. Structure nesting can achieve the reuse of fields and methods, while interfaces can achieve polymorphic effects. In actual development, we can choose the appropriate way to implement inheritance according to different needs to improve code reusability and maintainability.
The above is the detailed content of Analyze the way Golang implements inheritance and its applicable scenarios. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


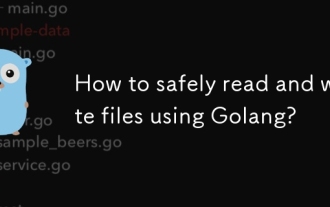
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
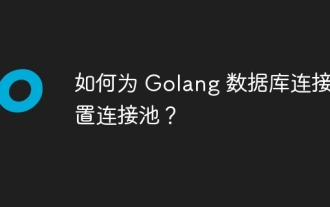
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
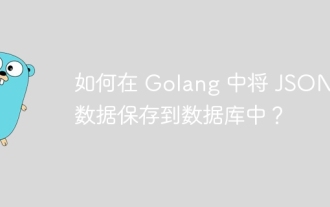
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
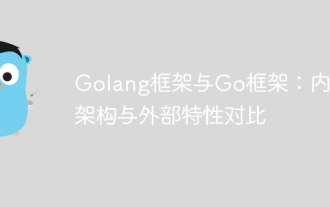
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
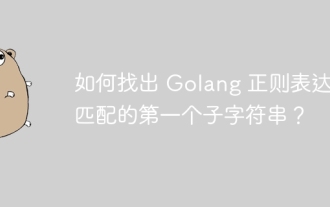
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
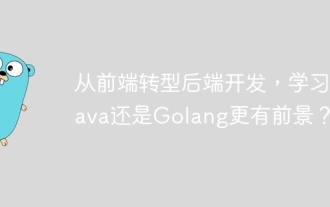
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
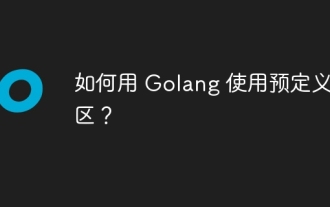
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
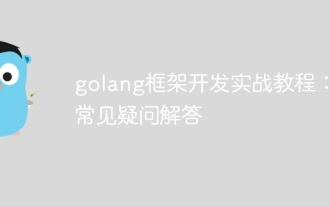
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
