Understand the key job tasks of Java testing
Understand the key tasks of Java testing, specific code examples are required
Java testing is a very important part of the software development process, it is designed to detect and verify the code correctness and reliability. Understanding the key job tasks of Java testing is essential for an experienced developer. This article will focus on the key tasks of Java testing and provide specific code examples.
1. Writing test cases
Writing test cases is the first step in Java testing. A test case is a sequence of inputs and expected outputs used to verify the correctness of the code. In Java, we can use JUnit framework to write test cases.
The following is a simple example that demonstrates how to write a test case:
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.assertEquals; public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); int result = calculator.add(2, 3); assertEquals(5, result); } @Test public void testSubtraction() { Calculator calculator = new Calculator(); int result = calculator.subtract(5, 3); assertEquals(2, result); } }
In the above code, we use JUnit’s @Test
annotation to mark the test Example. In each test case, we create a Calculator
instance and call its methods to perform the corresponding calculation operations. We then use the assertEquals
method to verify that the calculation results are as expected.
2. Execute tests
Executing tests is one of the key tasks of Java testing. Before executing tests, we need to ensure that our code is fully written and all dependencies are configured. Likewise, we can use JUnit framework to execute tests.
The following is a simple example showing how to execute the above test case:
import org.junit.jupiter.api.Test; import org.junit.jupiter.api.Assertions; import org.junit.jupiter.api.DisplayName; import org.junit.jupiter.api.Nested; @DisplayName("Calculator Test") public class CalculatorTest { @Nested @DisplayName("Addition Test") class AdditionTest { @Test @DisplayName("Test adding positive numbers") public void testAdditionWithPositiveNumbers() { Calculator calculator = new Calculator(); int result = calculator.add(2, 3); Assertions.assertEquals(5, result); } // 更多的测试用例... } @Nested @DisplayName("Subtraction Test") class SubtractionTest { @Test @DisplayName("Test subtracting positive numbers") public void testSubtractionWithPositiveNumbers() { Calculator calculator = new Calculator(); int result = calculator.subtract(5, 3); Assertions.assertEquals(2, result); } // 更多的测试用例... } }
In the above code, we used JUnit’s @Nested
annotations and @DisplayName
annotations organize and name tests. This can better organize our tests and improve the readability and maintainability of the code.
3. Handling exceptions
When conducting Java testing, you also need to consider possible exceptions in the code. We need to write test cases to verify the behavior of the code in the face of abnormal conditions.
The following is a simple example showing how to write a test case that handles exceptions:
import org.junit.jupiter.api.Test; import org.junit.jupiter.api.Assertions; public class CalculatorTest { @Test public void testDivisionByZero() { Calculator calculator = new Calculator(); Assertions.assertThrows(ArithmeticException.class, () -> calculator.divide(10, 0)); } }
In the above code, we have used JUnit’s assertThrows
method To verify whether the code will throw an ArithmeticException
exception. This ensures that our code correctly throws an exception when faced with a divide-by-zero exception rather than producing an incorrect result.
Summary:
Understanding the key tasks of Java testing is an essential skill for becoming an excellent Java developer. Writing test cases, executing tests, and handling exceptions are all important tasks in Java testing. Through the demonstration of sample code, I hope readers can better understand the key tasks of Java testing and be able to apply it to actual projects.
The above is the detailed content of Understand the key job tasks of Java testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


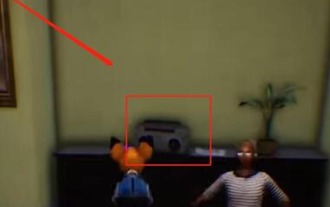
The Terror Corridor is a mission in Goat Simulator 3. How can you complete this mission? Master the detailed clearance methods and corresponding processes, and be able to complete the corresponding challenges of this mission. The following will bring you Goat Simulator. 3 Horror Corridor Guide to learn related information. Goat Simulator 3 Terror Corridor Guide 1. First, players need to go to Silent Hill in the upper left corner of the map. 2. Here you can see a house with RESTSTOP written on the roof. Players need to operate the goat to enter this house. 3. After entering the room, we first go straight forward, and then turn right. There is a door at the end here, and we go in directly from here. 4. After entering, we also need to walk forward first and then turn right. When we reach the door here, the door will be closed. We need to turn back and find it.
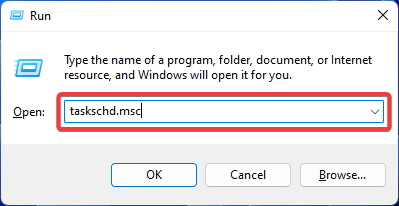
To automate tasks and manage multiple systems, mission planning software is a valuable tool in your arsenal, especially as a system administrator. Windows Task Scheduler does the job perfectly, but lately many people have reported operator rejected request errors. This problem exists in all iterations of the operating system, and even though it has been widely reported and covered, there is no effective solution. Keep reading to find out what might actually work for other people! What is the request in Task Scheduler 0x800710e0 that was denied by the operator or administrator? Task Scheduler allows automating various tasks and applications without user input. You can use it to schedule and organize specific applications, configure automatic notifications, help deliver messages, and more. it
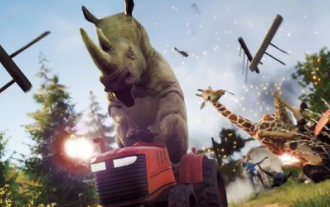
Goat Simulator 3 is a game with classic simulation gameplay, allowing players to fully experience the fun of casual action simulation. The game also has many exciting special tasks. Among them, the Goat Simulator 3 Imperial Tomb task requires players to find the bell tower. Some players are not sure how to operate the three clocks at the same time. Here is the guide to the Tomb of the Tomb mission in Goat Simulator 3! The guide to the Tomb of the Tomb mission in Goat Simulator 3 is to ring the bells in order. Detailed step expansion 1. First, players need to open the map and go to Wuqiu Cemetery. 2. Then go up to the bell tower. There will be three bells inside. 3. Then, in order from largest to smallest, follow the familiarity of 222312312. 4. After completing the knocking, you can complete the mission and open the door to get the lightsaber.

Rescue Steve is a unique task in Goat Simulator 3. What exactly needs to be done to complete it? This task is relatively simple, but we need to be careful not to misunderstand the meaning. Here we will bring you the rescue of Steve in Goat Simulator 3 Task strategies can help you better complete related tasks. Goat Simulator 3 Rescue Steve Mission Strategy 1. First come to the hot spring in the lower right corner of the map. 2. After arriving at the hot spring, you can trigger the task of rescuing Steve. 3. Note that there is a man in the hot spring. Although his name is Steve, he is not the target of this mission. 4. Find a fish named Steve in this hot spring and bring it ashore to complete this task.
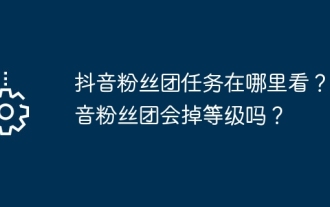
TikTok, as one of the most popular social media platforms at the moment, has attracted a large number of users to participate. On Douyin, there are many fan group tasks that users can complete to obtain certain rewards and benefits. So where can I find Douyin fan club tasks? 1. Where can I view Douyin fan club tasks? In order to find Douyin fan group tasks, you need to visit Douyin's personal homepage. On the homepage, you will see an option called "Fan Club." Click this option and you can browse the fan groups you have joined and related tasks. In the fan club task column, you will see various types of tasks, such as likes, comments, sharing, forwarding, etc. Each task has corresponding rewards and requirements. Generally speaking, after completing the task, you will receive a certain amount of gold coins or experience points.
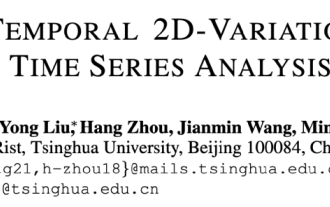
Achieving task universality is a core issue in the research of basic deep learning models, and is also one of the main focuses in the recent direction of large models. However, in the field of time series, various types of analysis tasks vary greatly. There are prediction tasks that require fine-grained modeling and classification tasks that require extracting high-level semantic information. How to build a unified deep basic model to efficiently complete various timing analysis tasks has not yet been established. To this end, a team from the School of Software of Tsinghua University conducted research on the basic issue of timing change modeling and proposed TimesNet, a task-universal timing basic model. The paper was accepted by ICLR 2023. Author list: Wu Haixu*, Hu Tengge*, Liu Yong*, Zhou Hang, Wang Jianmin, Long Mingsheng Link: https://ope
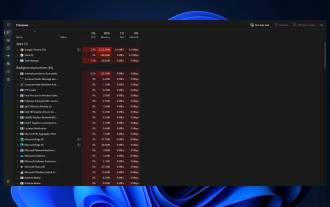
How to Pause Task Manager Process Updates in Windows 11 and Windows 10 Press CTRL+Window Key+Delete to open Task Manager. By default, Task Manager will open the Processes window. As you can see here, all the apps are endlessly moving around and it can be hard to point them down when you want to select them. So, press CTRL and hold it, this will pause the task manager. You can still select apps and even scroll down, but you must hold down the CTRL button at all times.
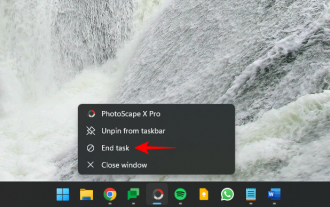
Frozen or unresponsive programs are easy to kill from Task Manager. But Microsoft has recently provided users with the facility to terminate these tasks directly from the taskbar. While the option isn't rolled out to everyone, it's easily available if you have the Windows Insider build. Here's everything you need to enable the End Task button and close tasks from the taskbar. How to Get the End Task Button from the Taskbar to Kill Apps Currently, the option to enable the End Task button for taskbar apps is only available as a developer option for users with Windows Insider builds. However, this may change in an upcoming feature update as it will be rolled out to users globally on the stable version. If you still
