Analysis and practice: Atomicity of variable assignment in Golang
Analysis and practice of atomicity of variable assignment in Golang
In concurrent programming, it is crucial to ensure the atomicity of data. In Golang, some mechanisms are provided to ensure the atomicity of variable assignment. This article will focus on the analysis and practice of this topic.
1. The concept of atomic operations
In concurrent programming, atomic operations refer to operations that will not be interrupted by other threads. They are either completed or not executed at all. In Golang, atomic operations can be implemented through functions in the sync/atomic package. These functions can ensure that operations on shared variables are atomic during concurrent execution.
2. How to implement atomic operations
The sync/atomic package in Golang provides a series of atomic operation functions, such as AddInt32, AddInt64, CompareAndSwapInt32, etc. The implementation of these functions is generally based on instructions provided by the underlying hardware, such as CAS (Compare and Swap) instructions, which ensure safe access to shared variables through atomic operations.
3. Atomic analysis of variable assignment
In Golang, variable assignment is generally divided into two steps: read operation and assignment operation. In a concurrent environment, if multiple coroutines assign values to the same variable at the same time, race conditions may occur, leading to data inconsistency.
In order to analyze the atomicity of variable assignment, when multiple coroutines are executed concurrently, we can use the atomic operation function in the sync/atomic package to ensure that the operation of shared variables is atomic. The following is a simple sample code:
package main import ( "fmt" "sync" "sync/atomic" ) var counter int64 func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() for i := 0; i < 1000; i++ { atomic.AddInt64(&counter, 1) } }() go func() { defer wg.Done() for i := 0; i < 1000; i++ { atomic.AddInt64(&counter, 1) } }() wg.Wait() fmt.Println("Counter:", counter) }
In this example, we use sync.WaitGroup to wait for the two coroutines to complete execution, and use the atomic.AddInt64 function to perform variable assignment operations. Through atomic operations, we can ensure that the increment operation of the counter variable is atomic and avoid the problem of race conditions.
4. Practice of atomicity of variable assignment
In actual development, in order to ensure the atomicity of variable assignment, we can use mechanisms such as mutex locks for protection. The following is a sample code for a mutex lock:
package main import ( "fmt" "sync" ) var counter int64 var mutex sync.Mutex func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() for i := 0; i < 1000; i++ { mutex.Lock() counter++ mutex.Unlock() } }() go func() { defer wg.Done() for i := 0; i < 1000; i++ { mutex.Lock() counter++ mutex.Unlock() } }() wg.Wait() fmt.Println("Counter:", counter) }
In this example, we use sync.Mutex to protect access to the counter variable. Through the Lock function and Unlock function, we can ensure that only one coroutine can access the variable at any time, thereby ensuring the atomicity of variable assignment.
Summary: In Golang, the atomicity of variable assignment is one of the issues that must be considered in concurrent programming. By using mechanisms such as atomic operation functions or mutex locks in the sync/atomic package, we can effectively ensure that operations on shared variables are atomic. Proper use of these mechanisms can improve the concurrency performance and stability of the program.
The above is the detailed content of Analysis and practice: Atomicity of variable assignment in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


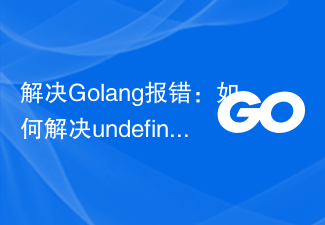
When developing with Golang, you often encounter undefinedidentifier errors. This error is caused by the presence of undefined identifiers in the code. In this article, we will cover common undefinedidentifier errors and their solutions. 1. Why does the undefinedidentifier error occur? Golang is a statically typed language, so

Advantages and Disadvantages of Using Golang to Develop Mobile Games With the popularity of mobile devices and the continuous improvement of their performance, the mobile game market is becoming more and more popular, attracting more and more developers to join it. When choosing a development language, Golang, as a fast, efficient and easy-to-learn language, attracts the attention of many developers. This article will discuss the advantages and disadvantages of using Golang to develop mobile games, and illustrate it through specific code examples. Advantages: Strong cross-platform: Golang can be compiled into binaries for different platforms

Analysis and practice of atomicity of variable assignment in Golang In concurrent programming, it is crucial to ensure the atomicity of data. In Golang, some mechanisms are provided to ensure the atomicity of variable assignment. This article will focus on the analysis and practice of this topic. 1. The concept of atomic operations In concurrent programming, atomic operations refer to operations that will not be interrupted by other threads. They are either completed or not executed at all. In Golang, atomic operations can be implemented through functions in the sync/atomic package. These functions
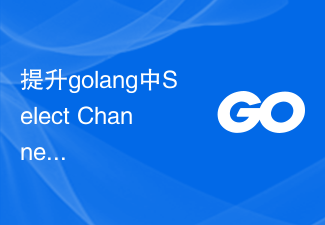
Methods to improve the efficiency of SelectChannelsGo concurrent programming in golang Introduction: With the continuous development of computer technology, multi-core and concurrent programming have gradually become an important direction in application development. In Go language, concurrent programming can be easily achieved by using goroutines and channels. The Select statement is a key tool for managing and controlling multiple channels. In this article, we will explore how to improve the use of Sele in golang
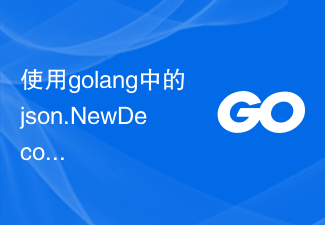
Streaming encoding and decoding of JSON using json.NewDecoder and json.NewEncoder functions in golang JSON is a lightweight data exchange format that is widely used in web applications and modern APIs due to its ease of reading and writing. In golang, we can use the json package to encode and decode JSON data. The json.NewDecoder and json.NewEncoder functions provide a stream
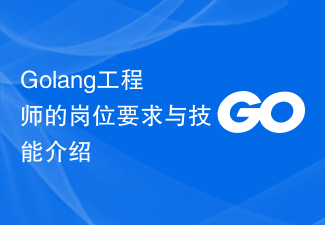
Job requirements and skill introduction for Golang engineers. With the rapid development of the Internet industry, Golang, as an efficient, concise and high-concurrency programming language, has gradually become favored by more and more companies. Therefore, the market demand for engineers with Golang skills is becoming increasingly strong. So, what job requirements and skills should a good Golang engineer have? Next, we'll introduce it, with specific code examples. 1. Job requirements: 1. Proficient in Golang programming
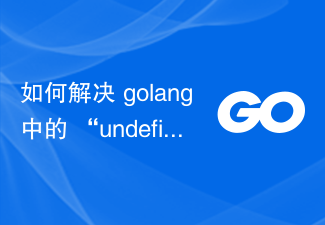
Go is a fast, efficient, compiled programming language. Due to its excellent performance and readability, it has gradually been favored by more and more developers in recent years. Database/sql is an important package in Go, which provides an interface for developers to interact with the database. However, in the process of using database/sql.Open, developers may encounter a classic error: "undefined: database/sql.Open". This article
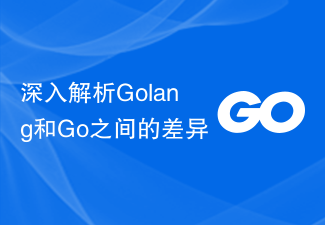
An in-depth analysis of the differences between Golang and Go Overview Golang and Go are two names for the same programming language. They refer to a simple, efficient, and concurrency-safe programming language developed by Google. Golang is the full name of the language, while Go is its more commonly used abbreviation. In this article, we will delve into the differences between Golang and Go and understand their development history, features, and usage scenarios. Development History The development of Golang can be traced back to 2007, by RobPike
