


In-depth discussion of the properties and solution process of matrix inverse in Numpy
Numpy Special Topic: Analysis of the Properties and Solution Process of Matrix Inverse
Introduction:
Matrix inverse is one of the important concepts in linear algebra. In scientific computing, matrix inversion can be used to solve many problems, such as solving linear equations, least squares method, etc. Numpy is a powerful scientific computing library in Python that provides a wealth of matrix operation tools, including related functions for matrix inverses. This article will introduce the properties and solution process of matrix inversion, and give specific code examples combined with functions in the Numpy library.
1. Definition and properties of matrix inverse:
- Definition: Given an n-order matrix A, if there exists an n-order matrix B, such that AB=BA=I (where I is the identity matrix), then matrix B is called the inverse matrix of matrix A, denoted as A^-1.
- Properties:
a. If the inverse of matrix A exists, then the inverse is unique.
b. If the inverse of matrix A exists, then A is a non-singular matrix (the determinant is not 0), and vice versa.
c. If matrices A and B are both non-singular matrices, then (AB)^-1 = B^-1 A^-1.
d. If matrix A is a symmetric matrix, its inverse matrix is also a symmetric matrix.
2. The solution process of matrix inverse:
Matrix inverse can be solved by a variety of methods, including Gaussian elimination method, LU decomposition method, eigenvalue decomposition method, etc. In Numpy, our common method is to use the inv function in the linear algebra module (linalg).
The following takes a 2x2 matrix as an example to show the calculation process of the matrix inverse:
Suppose we have a matrix A:
A = [[1, 2],
[3, 4]]
First, we use the inv function provided by Numpy to solve the inverse matrix:
import numpy as np
A = np.array([[1, 2], [3, 4]])
A_inv = np.linalg.inv(A)
Next, we verify whether the inverse matrix meets the defined requirements, that is, AA^-1 = A^-1A = I:
identity_matrix = np.dot(A, A_inv)
identity_matrix_inv = np.dot(A_inv, A)
print(identity_matrix)
print(identity_matrix_inv)
Run the above code, we will find that both outputs are identity matrices:
[[1. 0.]
[0. 1.]]
This proves that we are seeking The obtained matrix A_inv is indeed the inverse matrix of matrix A.
3. Application examples of matrix inverse:
Matrix inversion has a wide range of uses in practical applications. Let’s further illustrate this with an example.
Suppose we have a linear system of equations:
2x 3y = 8
4x 5y = 10
We can express this system of equations in matrix form as AX = B, where A is the coefficient matrix, X is the unknown vector (variable), and B is the constant vector. We can solve this system of equations by inverting the matrix.
import numpy as np
A = np.array([[2, 3], [4, 5]])
B = np.array([8, 10] )
A_inv = np.linalg.inv(A)
X = np.dot(A_inv, B)
print(X)
Run the above code, We will get the solution of the unknown vector X:
[1. 2.]
This means that the solution of the system of equations is x=1, y=2.
Through the above examples, we can see that the process of solving the matrix inverse is relatively simple, and the functions provided in the Numpy library allow us to easily solve the inverse matrix and apply it to practical problems.
Conclusion:
This article introduces the definition and properties of matrix inverse, analyzes the solution process of matrix inverse in detail, and gives specific code examples combined with functions in the Numpy library. By using the Numpy library, problems involving matrix inversions in scientific computing can be simplified and solved. I hope this article will be helpful to readers in learning and applying matrix inversion.
The above is the detailed content of In-depth discussion of the properties and solution process of matrix inverse in Numpy. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


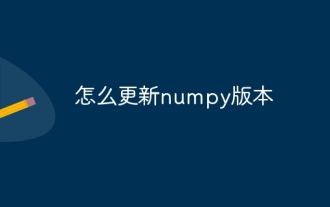
How to update the numpy version: 1. Use the "pip install --upgrade numpy" command; 2. If you are using the Python 3.x version, use the "pip3 install --upgrade numpy" command, which will download and install it, overwriting the current NumPy Version; 3. If you are using conda to manage the Python environment, use the "conda install --update numpy" command to update.
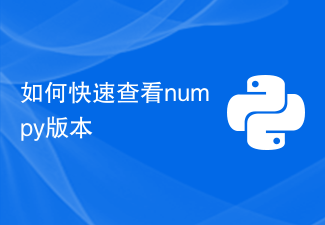
Numpy is an important mathematics library in Python. It provides efficient array operations and scientific calculation functions and is widely used in data analysis, machine learning, deep learning and other fields. When using numpy, we often need to check the version number of numpy to determine the functions supported by the current environment. This article will introduce how to quickly check the numpy version and provide specific code examples. Method 1: Use the __version__ attribute that comes with numpy. The numpy module comes with a __
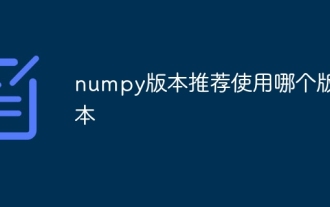
It is recommended to use the latest version of NumPy1.21.2. The reason is: Currently, the latest stable version of NumPy is 1.21.2. Generally, it is recommended to use the latest version of NumPy, as it contains the latest features and performance optimizations, and fixes some issues and bugs in previous versions.
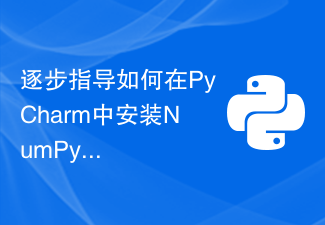
Teach you step by step to install NumPy in PyCharm and make full use of its powerful functions. Preface: NumPy is one of the basic libraries for scientific computing in Python. It provides high-performance multi-dimensional array objects and various functions required to perform basic operations on arrays. function. It is an important part of most data science and machine learning projects. This article will introduce you to how to install NumPy in PyCharm, and demonstrate its powerful features through specific code examples. Step 1: Install PyCharm First, we
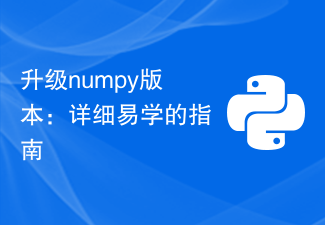
How to upgrade numpy version: Easy-to-follow tutorial, requires concrete code examples Introduction: NumPy is an important Python library used for scientific computing. It provides a powerful multidimensional array object and a series of related functions that can be used to perform efficient numerical operations. As new versions are released, newer features and bug fixes are constantly available to us. This article will describe how to upgrade your installed NumPy library to get the latest features and resolve known issues. Step 1: Check the current NumPy version at the beginning

How to add dimensions in numpy: 1. Use "np.newaxis" to add dimensions. "np.newaxis" is a special index value used to insert a new dimension at a specified position. You can use np.newaxis at the corresponding position. To increase the dimension; 2. Use "np.expand_dims()" to increase the dimension. The "np.expand_dims()" function can insert a new dimension at the specified position to increase the dimension of the array.
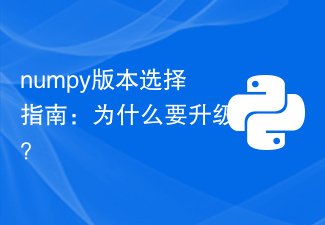
With the rapid development of fields such as data science, machine learning, and deep learning, Python has become a mainstream language for data analysis and modeling. In Python, NumPy (short for NumericalPython) is a very important library because it provides a set of efficient multi-dimensional array objects and is the basis for many other libraries such as pandas, SciPy and scikit-learn. In the process of using NumPy, you are likely to encounter compatibility issues between different versions, then
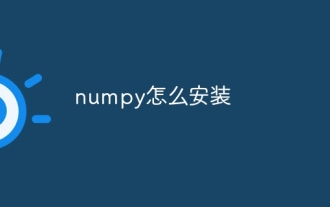
Numpy can be installed using pip, conda, source code and Anaconda. Detailed introduction: 1. pip, enter pip install numpy in the command line; 2. conda, enter conda install numpy in the command line; 3. Source code, unzip the source code package or enter the source code directory, enter in the command line python setup.py build python setup.py install.
