Why can't localstorage successfully save data?
Why does storing data to localstorage always fail? Need specific code examples
In front-end development, we often need to store data on the browser side to improve user experience and facilitate subsequent data access. Localstorage is a technology provided by HTML5 for client-side data storage. It provides a simple way to store data and maintain data persistence after the page is refreshed or closed.
However, when we use localstorage for data storage, we sometimes encounter storage failures. So, why does storing data to localstorage fail? Below we will explore some possible reasons for localstorage storage failure and give specific code examples.
- Storage space limit: Each browser has a limit on the storage space of localstorage. According to the specification, the maximum storage space of localstorage is 5MB. If we try to store more data than the browser limits, the storage will fail. We can avoid this problem by checking the storage space size of localstorage.
The following is a sample code to check the storage space size:
function checkStorageSpace() { var storageSpace = 0; for (var i = 0; i < localStorage.length; i++) { var key = localStorage.key(i); var value = localStorage.getItem(key); storageSpace += key.length + value.length; } return storageSpace; } var data = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; if (checkStorageSpace() + data.length <= 5 * 1024 * 1024) { localStorage.setItem("data", data); }
In the above code, we define a function checkStorageSpace()
to traverse localstorage of all data and calculate its size. Then, we define a data to be stored data
, and decide whether to store the data in localstorage by judging whether the current storage space is sufficient.
- Data conversion error: localstorage can only store string type data. If we try to store other types of data, such as objects or numbers, the storage will fail. In order to solve this problem, we need to convert the data to string type and then store it.
The following is a sample code to convert data to string type:
var data = { name: "John", age: 25, email: "john@example.com" }; localStorage.setItem("data", JSON.stringify(data));
In the above code, we define an object data
and then use JSON.stringify()
method converts it to string type and stores it in localstorage.
- Storage event triggering failure: The storage operation of localstorage is synchronous, which means that when we call the
setItem()
method to store data, it will directly trigger the storage event. If the browser's current storage space is full or the user has disabled localstorage, storage failure will occur.
In order to solve this problem, we can check whether localstorage is available before storing and give a prompt if necessary.
The following is a sample code to check whether localstorage is available:
function checkLocalStorageAvailability() { try { var testKey = "__test__"; localStorage.setItem(testKey, testKey); localStorage.removeItem(testKey); return true; } catch (e) { return false; } } if (checkLocalStorageAvailability()) { localStorage.setItem("data", "Lorem ipsum dolor sit amet, consectetur adipiscing elit."); } else { alert("Localstorage is not available!"); }
In the above code, we define a function checkLocalStorageAvailability()
to try to store a localstorage Test the key and delete it immediately. If this operation is successful, localstorage is available.
Through the above sample code, we can solve some common problems of localstorage storage failure. In actual development, we can also use try-catch statements to handle exceptions in storage operations to improve the robustness of the program.
To summarize, when storing data to localstorage fails, we need to consider issues such as storage space limitations, data type conversion errors, and storage event triggering failures. By properly handling these issues, we can effectively use localstorage to achieve persistent storage of data.
The above is the detailed content of Why can't localstorage successfully save data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Users usually upgrade the computer system version to fix some problems. If the user fails to update to the latest version of 23H2 using win11 system, there are three methods to solve your problem. What to do if Win11 update 23H2 fails. Method 1: Bypass TPM1, click "File Explorer - View", and check the "Hidden Items" option in the drop-down menu. 2. Go to and delete "C:\$WINDOWS.~BT\Sources\Panther-Appraiser_Data.ini". 3. Then re-create a folder with the same name in this location, and then click to cancel the "Hide Items" option. 4. Re-update the system and finally click on “Wind
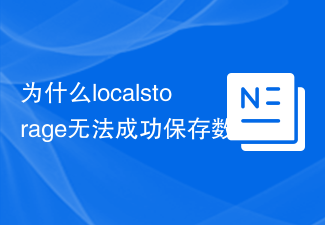
Why does storing data to localstorage always fail? Need specific code examples In front-end development, we often need to store data on the browser side to improve user experience and facilitate subsequent data access. Localstorage is a technology provided by HTML5 for client-side data storage. It provides a simple way to store data and maintain data persistence after the page is refreshed or closed. However, when we use localstorage for data storage, sometimes
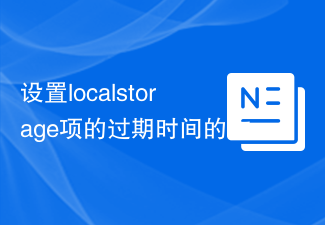
How to set the expiration time of localstorage requires specific code examples. With the rapid development of the Internet, front-end development often requires saving data in the browser. Localstorage is a commonly used WebAPI that aims to provide a way to store data locally in the browser. However, localstorage does not provide a direct way to set the expiration time. This article will introduce how to set the expiration time of localstorage through code examples.
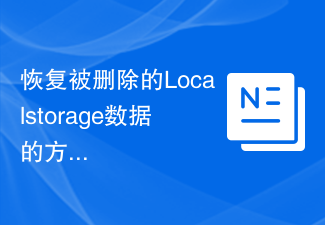
How to recover deleted Localstorage data? Localstorage is a technology used to store data in web pages. It is widely used in various web applications to share data between multiple pages. However, sometimes we may accidentally delete data in Localstorage, which causes us trouble. So, how to recover deleted Localstorage data? Below are specific steps and code examples. Step 1: Stop writing to Loca
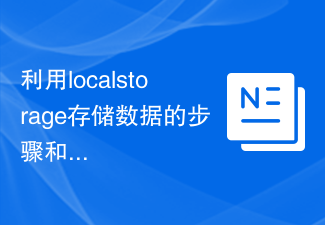
Steps and precautions for using localStorage to store data This article mainly introduces how to use localStorage to store data and provides relevant code examples. LocalStorage is a way of storing data in the browser that keeps the data local to the user's computer without going through a server. The following are the steps and things to pay attention to when using localStorage to store data. Step 1: Check whether the browser supports LocalStorage
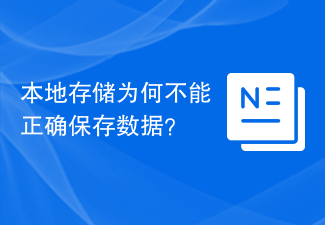
Why can't localstorage save my data normally? In web development, we often need to save the user's data locally so that the data can be quickly loaded or restored the next time the user visits the website. In the browser, we can use localStorage to achieve this function. However, sometimes we find that data saved using localStorage does not work properly. So why does this happen? In understanding why localStorage
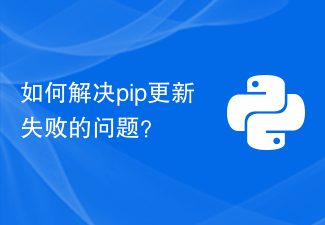
What should I do if pip update fails? Recently, while developing in Python, I encountered some problems with pip update failure. When developing, we often need to use pip to install, upgrade and remove Python third-party libraries. The failure of pip update will seriously affect our development work. This article will discuss some common pip update failures and provide solutions, hoping to help developers who encounter similar problems. First, when we execute pipinstall-
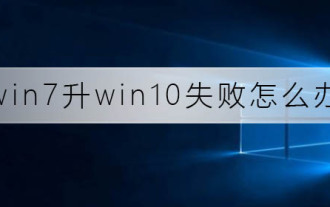
If the operating system we use is win7, some friends may fail to upgrade from win7 to win10 when upgrading. The editor thinks we can try upgrading again to see if it can solve the problem. Let’s take a look at what the editor did for details~ What to do if win7 fails to upgrade to win10. Method 1: 1. It is recommended to download a driver first to evaluate whether your computer can be upgraded to Win10. 2. Then use the driver test after upgrading. Check if there are any driver abnormalities, and then fix them with one click. Method 2: 1. Delete all files under C:\Windows\SoftwareDistribution\Download. 2.win+R run "wuauclt.e
