Reveal the main responsibilities and tasks of Java testing
Explore the core functions and work content of Java testing
Overview:
With the rapid development of software development, how to provide high-quality software products has become an important issue for enterprises A big challenge. As an important part of ensuring software quality, software testing plays a key role. This article will explore the core functions and work content of Java testing, and give specific code examples so that readers can better understand the actual application of Java testing.
1. The core functions of Java testing
- Predict software defects:
One of the core functions of Java testing is to start from the early stages of software design and development, that is, in the code Anticipate possible defects in software before writing it. To achieve this goal, testers need to participate in processes such as requirements analysis, design discussions, and code reviews, identify potential defect points, and make suggestions for improvements and optimizations. - Discover software defects:
Another core function of Java testing is to discover actual defects in the software. Testers conduct comprehensive and systematic testing of software by writing test cases, executing test scripts, simulating user operations, etc. They use various testing techniques and tools to improve test coverage and work to find and resolve defects in software as early as possible. - Ensuring software quality:
The ultimate goal of Java testing is to ensure software quality. Testers not only find defects, they also need to track and modify defects, and ensure that the software can work properly under different scenarios and pressures. To achieve this, they also need continuous integration and automated testing to increase testing efficiency and feedback speed.
2. The work content of Java testing
- Unit testing:
Unit testing is the basis of Java testing. It tests the smallest testable unit in the software. , that is, a method or function. By writing various test cases and assertion statements, testers can verify that the unit operates as expected and ensure its functional and logical correctness.
Sample code:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class MathUtilsTest { @Test public void testAdd() { MathUtils mathUtils = new MathUtils(); int sum = mathUtils.add(2, 3); assertEquals(5, sum); } @Test public void testMultiply() { MathUtils mathUtils = new MathUtils(); int product = mathUtils.multiply(2, 3); assertEquals(6, product); } } public class MathUtils { public int add(int a, int b) { return a + b; } public int multiply(int a, int b) { return a * b; } }
- Integration testing:
Integration testing is testing multiple units together to verify the interaction and collaboration between them is it right or not. Testers need to write integration test cases and simulate various real-life scenarios to ensure that the interfaces and dependencies between various modules, components, or services work properly.
Sample code:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); int sum = calculator.add(2, 3); assertEquals(5, sum); } } public class Calculator { public int add(int a, int b) { MathUtils mathUtils = new MathUtils(); return mathUtils.add(a, b); } public int subtract(int a, int b){ MathUtils mathUtils = new MathUtils(); return mathUtils.subtract(a, b); } } public class MathUtils { public int add(int a, int b) { return a + b; } public int subtract(int a, int b) { return a - b; } }
- GUI testing:
GUI testing is the testing of the graphical user interface of the software to verify that the user interaction and interface layout are as expected . Testers need to simulate user operations, input and click events, check the response and display of the interface, and ensure the stability and ease of use of the interface in various scenarios.
Sample code:
import org.junit.jupiter.api.Test; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class LoginPageTest { @Test public void testLogin() { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("http://example.com/login"); WebElement usernameInput = driver.findElement(By.id("username")); WebElement passwordInput = driver.findElement(By.id("password")); WebElement submitButton = driver.findElement(By.id("submit")); usernameInput.sendKeys("user1"); passwordInput.sendKeys("123456"); submitButton.click(); WebElement welcomeMessage = driver.findElement(By.id("welcome-message")); assertEqual("Welcome, user1!", welcomeMessage.getText()); driver.quit(); } }
Summary:
As an important part of ensuring software quality, Java testing requires testers to have a solid Java programming foundation and testing technology knowledge. In practice, Java testers need to predict software defects, discover software defects and ensure software quality. By writing different types of test cases such as unit tests, integration tests, and GUI tests, combined with appropriate tools and frameworks, Java testers can provide strong support for software development and ensure the improvement of software quality.
The above is the detailed content of Reveal the main responsibilities and tasks of Java testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


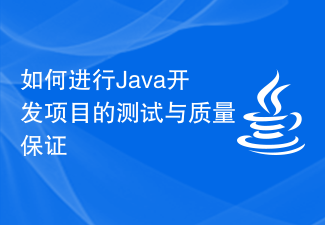
How to conduct testing and quality assurance of Java development projects In comprehensive software development projects, testing and quality assurance are important links to ensure the stability, reliability and effectiveness of the final software product. For Java development projects, comprehensive testing and quality assurance are also required to ensure the successful implementation of the project. This article will discuss how to conduct testing and quality assurance of Java development projects. Define a test plan Before conducting testing, you first need to develop a test plan. The test plan should include the scope of the test, the purpose and objectives of the test, the environment of the test
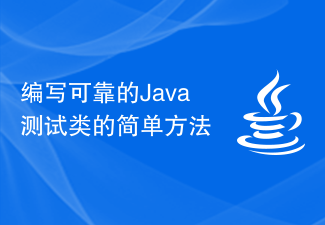
Simple and easy-to-understand method of writing Java test classes: Make your code more reliable Introduction: In the software development process, writing test code is a very important link. By testing the code, we can verify whether our code can run normally, and can quickly locate and fix problems in subsequent development. This article will introduce a simple and easy-to-understand method of writing Java test classes to help readers better conduct code testing. What is test code? Test code is a piece of code used to verify the function of the program. Its purpose is to determine whether the code is as expected.
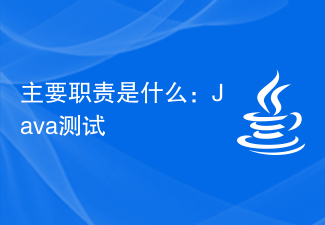
What is the main job of Java testing? In the entire life cycle of software development, testing is a crucial link. The goal of testing is to ensure the quality of the software and to verify that the software meets requirements and expectations. Java is a widely used programming language that is widely used in enterprise-level applications, mobile applications, cloud services and other fields. In Java development, testing also plays a vital role. The main work of Java testing includes the following aspects: Unit Testing (UnitTesting): Unit testing is
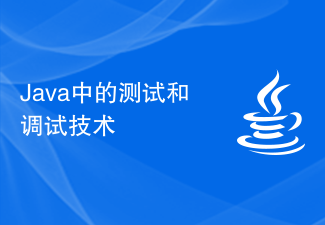
Java is a very popular programming language because it is portable, easy to learn and use, and has a strong community support. Testing and debugging are inevitable steps in writing quality software. In this article, we will explore testing and debugging techniques in Java to help you better understand how to write reliable Java applications. 1. Testing technology Testing refers to evaluating and verifying the correctness, integrity, validity, reliability, security and other quality attributes of the software through various means at different stages of software development.
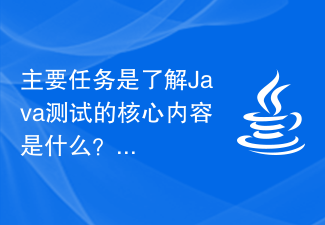
Explore what are the core tasks of Java testing? With the rapid development of software development, testing has become one of the key links to ensure software quality. In Java development, testing is an indispensable part. Java testing mainly includes different levels of testing such as unit testing, integration testing and system testing. So, what is the core task of Java testing? This article explores this. First of all, one of the core tasks of Java testing is to ensure the correctness of the code. Whether it is unit testing or integration testing, their
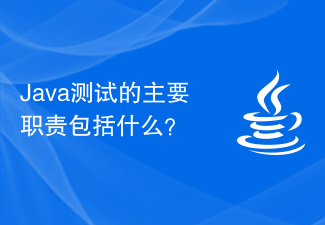
Understand what are the main job functions of Java testing? Abstract: With the increasing development of software development technology, testing work is becoming more and more important in the software life cycle. In Java development, testing is not only to ensure the quality of the code, but also to confirm whether the software meets requirements and expectations. This article will introduce the main work functions of Java testing, including test plan formulation, test case design, test execution and result analysis, etc. Text: Test Plan Developing a test plan is the basis of testing work. It describes the scope, goals, and resources of the test.
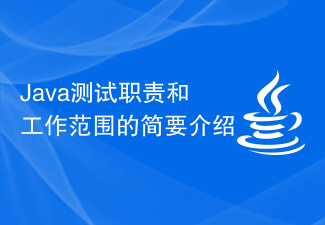
Java testing is an indispensable part of software development. It is mainly responsible for ensuring the quality and stability of software. This article will introduce the responsibilities and scope of work of Java testing, and provide some specific code examples. 1. Java testing responsibilities: Write test plans and test cases: Write detailed test plans and test cases based on software requirements and design documents, including functional testing, performance testing, security testing, etc. Execute testing: According to the test plan and test cases, perform various testing tasks, including unit testing and integration testing
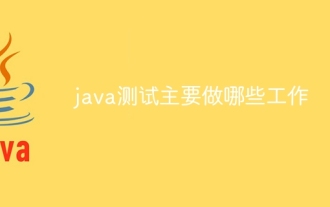
Java testing mainly involves: 1. Unit testing; 2. Integration testing; 3. Functional testing; 4. Performance testing; 5. Security testing; 6. Continuous integration and automated testing; 7. User acceptance testing; 8. Regression Testing etc. Detailed introduction: In Java development, testing is a key step to ensure software quality. These test levels and types are often combined in different stages to form a comprehensive testing strategy to ensure the stability, performance and security of the software.
