How to use dynamic proxy in Spring
Steps to use dynamic proxy in Spring: 1. Define an interface; 2. Create a target class; 3. Create a proxy class; 4. Configure notifications; 5. Run the application. Detailed introduction: 1. To define an interface, you first need to define an interface, which will be implemented by the proxy object. This interface defines the behavior that you want to execute before, after, and when an exception is thrown; 2. Create a target class, create a target class MyServiceImpl that implements the MyService interface. This class contains what you want to do before the method is called, etc.
The operating system for this tutorial: Windows 10 system, DELL G3 computer.
In the Spring framework, dynamic proxy is a commonly used technology for dynamically creating proxy objects at runtime in order to implement AOP (aspect-oriented programming) functions. Dynamic proxies allow programmers to define cross-cutting concerns that can execute specific code before and after a method is called, when an exception is thrown, when executing within a transaction, etc.
The following are the steps to implement AOP in Spring using dynamic proxy:
1. Define an interface: First, you need to define an interface, which will be implemented by the proxy object . This interface defines the behavior you want to perform before and after method calls, when exceptions are thrown, etc. For example, you can define an interface named MyService that contains a doSomething() method.
public interface MyService { void doSomething(); }
2. Create a target class: Create a target class MyServiceImpl that implements the MyService interface. This class contains the code you want to execute before, after, etc. the method is called.
public class MyServiceImpl implements MyService { public void doSomething() { System.out.println("Doing something..."); } }
3. Create a proxy class: Use the AOP framework provided by Spring to create a proxy class. Spring provides two types of dynamic proxies: JDK dynamic proxies and CGLIB dynamic proxies. Here we take the JDK dynamic proxy as an example and use ProxyFactoryBean to create a proxy class MyServiceProxy.
import org.springframework.aop.framework.ProxyFactoryBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AppConfig { @Bean public MyService myService() { return new MyServiceImpl(); } @Bean public MyServiceProxy myServiceProxy() { ProxyFactoryBean factory = new ProxyFactoryBean(); factory.setTargetBeanName("myService"); // 指定目标对象的名字 factory.addAdvice(new SimpleTraceInterceptor()); // 添加通知,定义在方法调用之前、之后等场景中执行的代码 return (MyServiceProxy) factory.getObject(); // 获取代理对象 } }
4. Configuration notification: In the proxy class, you need to configure the notification (Advice) to define the code to be executed before and after the method is called. Here we use SimpleTraceInterceptor as an example, which will output logs before and after method calls. You can customize the notification implementation as needed.
5. Run the application: Start the application and test whether the dynamic proxy works as expected. You can verify that the notification executed correctly by calling various methods on the target object. In the above example, when myServiceProxy().doSomething() is called, the log "Doing something..." will be output, and the corresponding logs will be output before and after the method call.
In addition to the JDK dynamic proxy used in the above example, Spring also provides CGLIB dynamic proxy as another option. CGLIB dynamic proxy is suitable for classes that do not implement interfaces. The class file of the proxy object class is loaded through the bytecode processing framework asm, and subclasses are generated by modifying the bytecode. The configuration method of using CGLIB dynamic proxy is similar to that of JDK dynamic proxy, except that different classes or configuration methods need to be used when creating proxy objects.
The above is the detailed content of How to use dynamic proxy in Spring. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
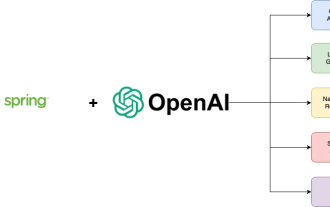
In 2023, AI technology has become a hot topic and has a huge impact on various industries, especially in the programming field. People are increasingly aware of the importance of AI technology, and the Spring community is no exception. With the continuous advancement of GenAI (General Artificial Intelligence) technology, it has become crucial and urgent to simplify the creation of applications with AI functions. Against this background, "SpringAI" emerged, aiming to simplify the process of developing AI functional applications, making it simple and intuitive and avoiding unnecessary complexity. Through "SpringAI", developers can more easily build applications with AI functions, making them easier to use and operate.

How to implement spring programmatic transactions: 1. Use TransactionTemplate; 2. Use TransactionCallback and TransactionCallbackWithoutResult; 3. Use Transactional annotations; 4. Use TransactionTemplate in combination with @Transactional; 5. Customize the transaction manager.
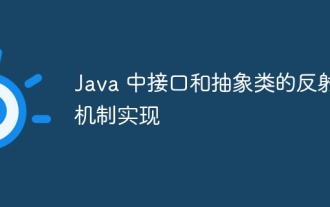
The reflection mechanism allows programs to obtain and modify class information at runtime. It can be used to implement reflection of interfaces and abstract classes: Interface reflection: obtain the interface reflection object through Class.forName() and access its metadata (name, method and field) . Reflection of abstract classes: Similar to interfaces, you can obtain the reflection object of an abstract class and access its metadata and non-abstract methods. Practical case: The reflection mechanism can be used to implement dynamic proxies, intercepting calls to interface methods at runtime by dynamically creating proxy classes.
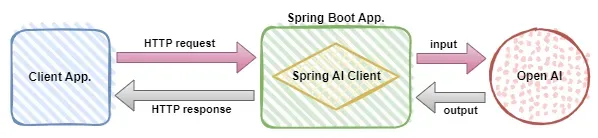
As an industry leader, Spring+AI provides leading solutions for various industries through its powerful, flexible API and advanced functions. In this topic, we will delve into the application examples of Spring+AI in various fields. Each case will show how Spring+AI meets specific needs, achieves goals, and extends these LESSONSLEARNED to a wider range of applications. I hope this topic can inspire you to understand and utilize the infinite possibilities of Spring+AI more deeply. The Spring framework has a history of more than 20 years in the field of software development, and it has been 10 years since the Spring Boot 1.0 version was released. Now, no one can dispute that Spring
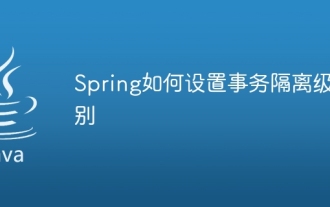
How to set the transaction isolation level in Spring: 1. Use the @Transactional annotation; 2. Set it in the Spring configuration file; 3. Use PlatformTransactionManager; 4. Set it in the Java configuration class. Detailed introduction: 1. Use the @Transactional annotation, add the @Transactional annotation to the class or method that requires transaction management, and set the isolation level in the attribute; 2. In the Spring configuration file, etc.
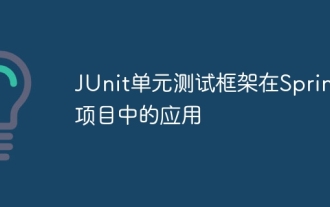
JUnit is a widely used Java unit testing framework in Spring projects and can be applied by following steps: Add JUnit dependency: org.junit.jupiterjunit-jupiter5.8.1test Write test cases: Use @ExtendWith(SpringExtension.class) to enable extension, use @Autowired inject beans, use @BeforeEach and @AfterEach to prepare and clean, and mark test methods with @Test.
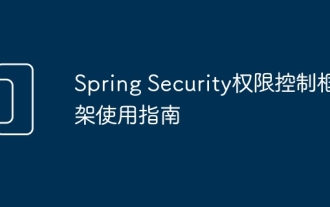
In back-end management systems, access permission control is usually required to limit different users' ability to access interfaces. If a user lacks specific permissions, he or she cannot access certain interfaces. This article will use the waynboot-mall project as an example to introduce how common back-end management systems introduce the permission control framework SpringSecurity. The outline is as follows: waynboot-mall project address: https://github.com/wayn111/waynboot-mall 1. What is SpringSecurity? SpringSecurity is an open source project based on the Spring framework, aiming to provide powerful and flexible security for Java applications.
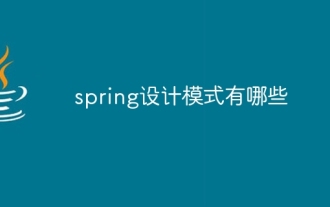
Spring design patterns include: 1. Dependency injection and control inversion; 2. Factory pattern; 3. Template pattern; 4. Observer pattern; 5. Decorator pattern; 6. Singleton pattern; 7. Strategy pattern and adapter pattern, etc. . Detailed introduction: 1. Dependency injection and inversion of control: These two design patterns are the core of the Spring framework. Through dependency injection, Spring is responsible for managing and injecting dependencies between components, reducing the coupling between components. Inversion of control refers to handing over object creation and dependency management to the Spring container, etc.
