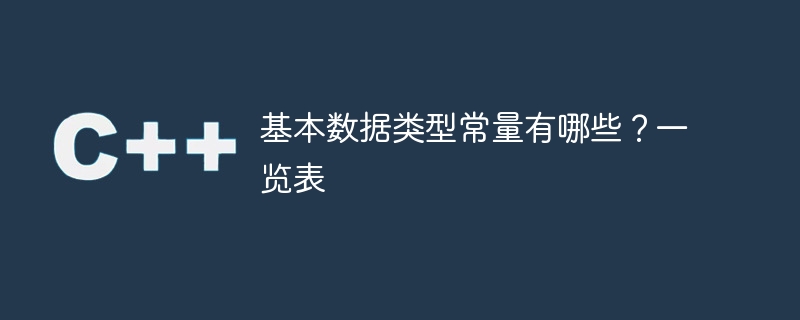
What are the basic data type constants? List, specific code examples required
In computer programming, a constant is a quantity that does not change its value while the program is running. In various programming languages, constants are divided into different types, which include basic data type constants. Basic data type constants refer to constants with fixed types and fixed values, which occupy a fixed storage space in memory.
The following is a list of basic data type constants, as well as corresponding specific code examples:
- Integer constants:
- Decimal constants: Decimal constants are composed of the number 0~ A value consisting of 9 without decimal point or exponent. For example, integer constants 10, 20, 30, etc.
- Octal constant: Octal constant is a value starting with the number 0 and consisting of the numbers 0~7. For example, octal constants 012, 034, etc.
- Hexadecimal constant: Hexadecimal constant is a value starting with 0x or 0X and consisting of numbers 0~9 and letters A~F (both upper and lower case). For example, hexadecimal constants 0xFF, 0x1A, etc.
Sample code:
int decimalConstant = 10;
int octalConstant = 012;
int hexadecimalConstant = 0xA;
Copy after login
- Floating point number constant:
- Decimal floating point number constant: Decimal floating point number constant is composed of integer part, decimal point and decimal part composed value. For example, floating point constants 3.14, 0.1, etc.
- Scientific notation floating point number constant: The floating point number constant expressed in scientific notation consists of the real part, the exponent and the letter E (or e) representing the exponent. For example, the floating-point constant 3.14E2 represents 3.14 times 10 raised to the power 2.
Sample code:
double decimalFloatingPointConstant = 3.14;
double scientificNotationFloatingPointConstant = 3.14E2;
Copy after login
- Character constants:
Character constants are values consisting of a single character, enclosed in single quotes. For example, character constants 'a', 'A', etc.
Sample code:
char characterConstant = 'a';
Copy after login
- String constant:
A string constant is a value composed of multiple characters, using double quotes to enclose the character sequence. For example, string constant "Hello World" etc.
Sample code:
String stringConstant = "Hello World";
Copy after login
- Boolean constants:
Boolean constants have only two values, true and false, used to represent logical true and logical false.
Sample code:
boolean booleanConstant = true;
Copy after login
- Empty constant:
Empty constant represents a special value that does not refer to any object. In Java, empty constants are represented by null.
Sample code:
String nullConstant = null;
Copy after login
The above is a list of common basic data type constants and corresponding code examples. Mastering the concepts and usage of these basic data type constants is very important basic knowledge for computer programming. Whether you are learning programming or in the actual development process, you need to be familiar with and flexibly use these constants to complete various tasks. Hope this article can be helpful to readers.
The above is the detailed content of What are the common basic data type constants? Summary Table. For more information, please follow other related articles on the PHP Chinese website!