Analyze the time complexity and applicability of Java bubble sort
Time complexity analysis and application scenarios of Java bubble sort
[Introduction]
Bubble Sort (Bubble Sort) is a basic sorting algorithm . It works by repeatedly exchanging adjacent out-of-order elements until the sequence is sorted. The time complexity of bubble sort is high, but its implementation is simple and suitable for sorting small-scale data.
[Algorithm Principle]
The algorithm principle of bubble sort is very simple. First, two adjacent elements are compared from the sequence, and if the order is wrong, the positions are exchanged; then, each pair of adjacent elements in the sequence is compared and exchanged in turn, until the entire sequence is sorted.
[Pseudocode]
The following is a pseudocode example of bubble sorting:
function bubbleSort(arr): n = arr.length for i = 0 to (n-1): for j = 0 to (n-1-i): if arr[j] > arr[j+1]: swap(arr[j], arr[j+1]) return arr
[Time complexity analysis]
The time complexity of bubble sorting depends on the number of elements n. In the best case, the sequence is already in order, and only one round of comparison is needed to determine that the sorting is complete, and the time complexity is O(n). In the worst case, the sequence is completely in reverse order, requiring n bubble operations, and the time complexity is O(n^2). On average, the time complexity is also O(n^2). Therefore, the time complexity of bubble sort is O(n^2).
[Application Scenario]
Bubble sorting has a high time complexity, so it is not suitable for sorting large-scale data. However, due to its simple implementation and clear logic, it is a better choice for sorting small-scale data. Its application scenarios include:
- When you need to manually implement the sorting algorithm, bubble sort is a simple and easy-to-understand choice;
- When the array size is small and there is no need When considering performance requirements, bubble sort can meet the sorting needs;
- When the array to be sorted is already basically ordered, the advantages of bubble sort appear because it only requires a limited number of comparisons and exchanges.
[Java code example]
The following is a bubble sort example code implemented in Java:
public class BubbleSort { public static void main(String[] args) { int[] arr = {5, 2, 8, 9, 1}; bubbleSort(arr); System.out.println(Arrays.toString(arr)); } public static void bubbleSort(int[] arr) { int n = arr.length; for (int i = 0; i < n-1; i++) { for (int j = 0; j < n-1-i; j++) { if (arr[j] > arr[j+1]) { int temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } } } } }
The above code example demonstrates how to use bubble sort to sort an integer array Sort. The running result is [1, 2, 5, 8, 9].
[Summary]
Although bubble sort has high time complexity, the implementation is simple and easy to understand. It is suitable for sorting small-scale data, especially when you need to manually implement a sorting algorithm or sort a basically ordered array. However, bubble sort performs poorly when dealing with large-scale data, so its use in this scenario is not recommended.
The above is the detailed content of Analyze the time complexity and applicability of Java bubble sort. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
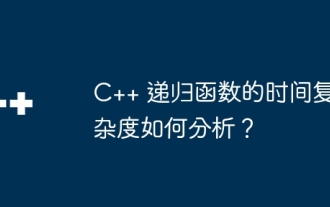
Time complexity analysis of recursive functions involves: identifying base cases and recursive calls. Calculate the time complexity of the base case and each recursive call. Sum the time complexity of all recursive calls. Consider the relationship between the number of function calls and the size of the problem. For example, the time complexity of the factorial function is O(n) because each recursive call increases the recursion depth by 1, giving a total depth of O(n).
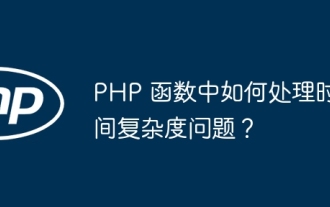
Time complexity is a measure of how long a function takes to execute. Common PHP function time complexity problems include nested loops, large array traversals, and recursive calls. Techniques for optimizing time complexity include: using caching to reduce the number of loops simplifying algorithms using parallel processing
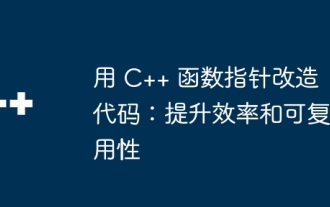
Function pointer technology can improve code efficiency and reusability, specifically as follows: Improved efficiency: Using function pointers can reduce repeated code and optimize the calling process. Improve reusability: Function pointers allow the use of general functions to process different data, improving program reusability.
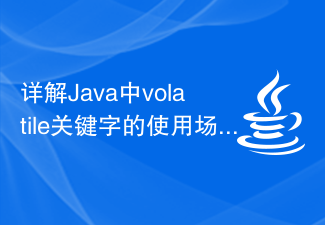
Detailed explanation of the role and application scenarios of the volatile keyword in Java 1. The role of the volatile keyword In Java, the volatile keyword is used to identify a variable that is visible between multiple threads, that is, to ensure visibility. Specifically, when a variable is declared volatile, any modifications to the variable are immediately known to other threads. 2. Application scenarios of the volatile keyword The status flag volatile keyword is suitable for some status flag scenarios, such as a
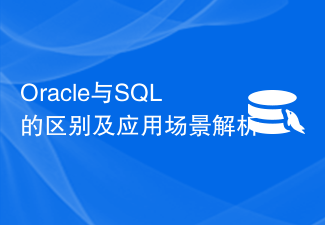
The difference between Oracle and SQL and analysis of application scenarios In the database field, Oracle and SQL are two frequently mentioned terms. Oracle is a relational database management system (RDBMS), and SQL (StructuredQueryLanguage) is a standardized language for managing relational databases. While they are somewhat related, there are also some significant differences. First of all, by definition, Oracle is a specific database management system, consisting of

The Go language is suitable for a variety of scenarios, including back-end development, microservice architecture, cloud computing, big data processing, machine learning, and building RESTful APIs. Among them, the simple steps to build a RESTful API using Go include: setting up the router, defining the processing function, obtaining the data and encoding it into JSON, and writing the response.
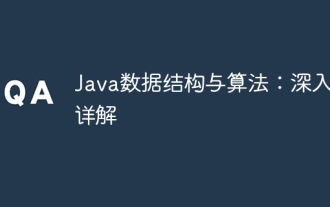
Data structures and algorithms are the basis of Java development. This article deeply explores the key data structures (such as arrays, linked lists, trees, etc.) and algorithms (such as sorting, search, graph algorithms, etc.) in Java. These structures are illustrated through practical examples, including using arrays to store scores, linked lists to manage shopping lists, stacks to implement recursion, queues to synchronize threads, and trees and hash tables for fast search and authentication. Understanding these concepts allows you to write efficient and maintainable Java code.
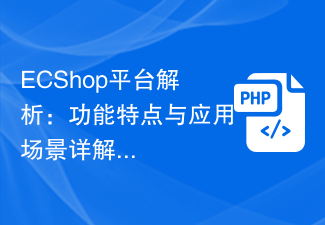
ECShop platform analysis: Detailed explanation of functional features and application scenarios ECShop is an open source e-commerce system developed based on PHP+MySQL. It has powerful functional features and a wide range of application scenarios. This article will analyze the functional features of the ECShop platform in detail, and combine it with specific code examples to explore its application in different scenarios. Features 1.1 Lightweight and high-performance ECShop adopts a lightweight architecture design, with streamlined and efficient code and fast running speed, making it suitable for small and medium-sized e-commerce websites. It adopts the MVC pattern
