


Explore the power of PHP built-in objects: Learn what built-in objects PHP provides
In-depth analysis of PHP built-in objects: To explore the functions of built-in objects provided by PHP, specific code examples are required
As a commonly used server-side scripting language, PHP has built-in It contains many powerful objects that can help developers develop web applications more efficiently. In this article, we will take an in-depth analysis of PHP built-in objects, explore their functions and how to use them to improve programming efficiency.
- stdClass object
The stdClass object is a special object in PHP, which can be used as a general extension object. Through the stdClass object, we can dynamically create properties and methods at runtime. Here is a specific example:
$person = new stdClass(); $person->name = 'John Doe'; $person->age = 30; $person->getInfo = function () use ($person) { echo "Name: {$person->name}, Age: {$person->age}"; }; $person->getInfo(); // 输出:Name: John Doe, Age: 30
- DateTime Object
The DateTime object is a powerful tool for working with dates and times in PHP. Through the DateTime object, we can perform various date and time operations, such as calculating date differences, formatting dates, comparing dates, and so on. The following is a specific example:
$now = new DateTime(); echo $now->format('Y-m-d'); // 输出当前日期,例如:2022-01-01 $birthday = new DateTime('1990-01-01'); $age = $now->diff($birthday)->y; echo "Age: {$age}"; // 输出:Age: 32
- ArrayObject object
The ArrayObject object is a class used to encapsulate arrays in PHP. Through the ArrayObject object, we can operate objects like arrays. It provides a series of methods, such as adding elements, deleting elements, traversing elements, etc. The following is a specific example:
$fruits = new ArrayObject(['apple', 'banana', 'orange']); $fruits->append('kiwi'); $fruits->offsetUnset(1); foreach ($fruits as $fruit) { echo $fruit . ', '; // 输出:apple, orange, kiwi, }
- Exception object
The Exception object is the standard class for handling exceptions in PHP. Through the Exception object, we can throw and catch exceptions, and handle them accordingly when they occur. The following is a specific example:
function divide($dividend, $divisor) { if ($divisor == 0) { throw new Exception('Divisor cannot be zero'); } return $dividend / $divisor; } try { $result = divide(10, 0); echo "Result: {$result}"; } catch (Exception $e) { echo "Error: {$e->getMessage()}"; // 输出:Error: Divisor cannot be zero }
- PDO object
The PDO object is a tool class used for database operations in PHP. It provides developers with a series of methods to connect and operating databases. Through PDO objects, we can execute SQL statements, process transactions, prepare statements, etc. The following is a specific example:
$dsn = 'mysql:host=localhost;dbname=test'; $user = 'root'; $pass = 'password'; try { $pdo = new PDO($dsn, $user, $pass); $stmt = $pdo->query('SELECT * FROM users'); while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { echo $row['name'] . ', '; // 输出:John Doe, Jane Smith, ... } } catch (PDOException $e) { echo "Error: {$e->getMessage()}"; }
The above is just the tip of the iceberg of PHP's built-in objects. PHP also provides many other useful built-in objects, such as SplFileObject, SimpleXMLElement, DOMDocument, and so on. By in-depth understanding and flexible use of these built-in objects, we can develop PHP programs more efficiently. I hope this article can help readers better grasp the functions and usage techniques of PHP's built-in objects.
The above is the detailed content of Explore the power of PHP built-in objects: Learn what built-in objects PHP provides. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
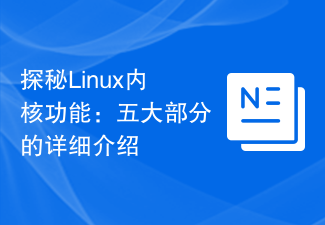
As the core part of the operating system, the Linux kernel is responsible for important functions such as managing hardware resources and providing system calls. This article will delve into the five major parts of the Linux kernel, including process management, file system, network communication, device driver and memory management, and provide a detailed introduction and code examples. 1. Process Management Process Creation In the Linux kernel, process creation is implemented through the fork() system call. Here is a simple example code: #include
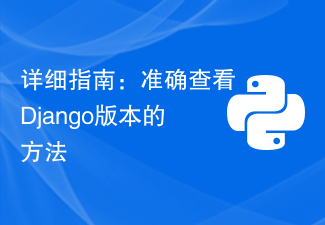
For an in-depth analysis of how to accurately view the Django version, specific code examples are required. Introduction: As a popular Python Web framework, Django often requires version management and upgrades. However, sometimes it may be difficult to check the Django version number in the project, especially when the project has entered the production environment, or uses a large number of custom extensions and partial modules. This article will introduce in detail how to accurately check the version of the Django framework, and provide some code examples to help developers better manage
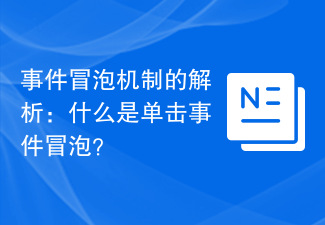
What is click event bubbling? In-depth analysis of the event bubbling mechanism requires specific code examples. Event bubbling (Event Bubbling) means that in the DOM tree structure, when an element triggers an event, the event will be passed along the DOM tree from the child elements to the root element. This process is like bubbles bubbling, so it is called event bubbling. Event bubbling is a mechanism of the DOM event model, included in documents such as HTML, XML, and SVG. This mechanism allows event handlers registered on the parent element to receive
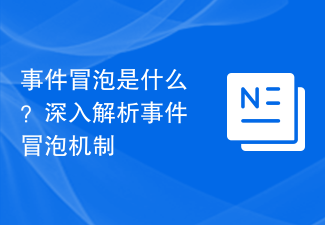
What is event bubbling? In-depth analysis of the event bubbling mechanism Event bubbling is an important concept in web development, which defines the way events are delivered on the page. When an event on an element is triggered, the event will be transmitted starting from the innermost element and passed outwards until it is passed to the outermost element. This delivery method is like bubbles bubbling in water, so it is called event bubbling. In this article, we will analyze the event bubbling mechanism in depth. The principle of event bubbling can be understood through a simple example. Suppose we have an H
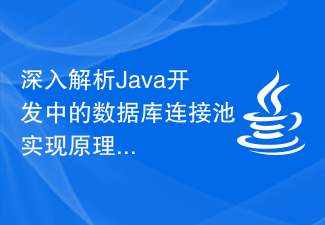
In-depth analysis of the implementation principle of database connection pool in Java development. In Java development, database connection is a very common requirement. Whenever we need to interact with the database, we need to create a database connection and then close it after performing the operation. However, frequently creating and closing database connections has a significant impact on performance and resources. In order to solve this problem, the concept of database connection pool was introduced. The database connection pool is a caching mechanism for database connections. It creates a certain number of database connections in advance and
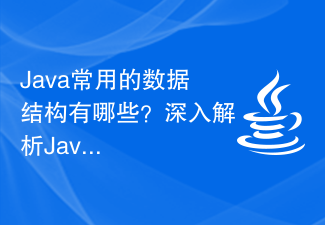
Java is a widely used programming language, and data structures are an integral part of the development process. Data structures help organize and manage data and improve program execution efficiency. In Java, commonly used data structures include arrays, linked lists, stacks, queues, trees, graphs, etc. This article will provide an in-depth analysis of these commonly used Java data structures and provide specific code examples. 1. Array Array is a linear data structure that can store elements of the same type. In Java, you can declare using
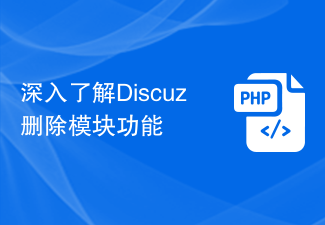
Since Discuz is an open source forum system, users can customize functions according to their own needs. Among them, the delete module function is one of the more common and important functions. Through the delete module function, administrators can delete content in the forum to keep the forum orderly and clean. This article will deeply explore how to implement the delete module function in Discuz, provide specific code examples, and guide readers to understand its implementation principles. 1. The role of the delete module function The delete module function plays an important role in Discuz
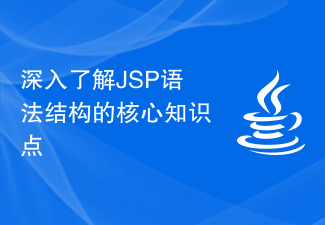
JSP syntax structure: Core knowledge point analysis JSP (JavaServerPages) is a server-side scripting language used to create dynamic web pages. The JSP syntax structure is simple and easy to learn, but it is powerful and can meet various complex web development needs. 1. JSP page structure A JSP page usually consists of the following parts: Directives: Directives are used to tell the JSP container how to process the page. Common instructions are: used to set
