


Methods to solve the problem of Chinese garbled characters in matplotlib
How to solve the problem of garbled Chinese display in Matplotlib, specific code examples are needed
Abstract:
Matplotlib is a tool for creating various forms of charts Python library. However, when using Matplotlib to draw Chinese characters, we often encounter the problem of garbled characters. This article will introduce how to solve the problem of garbled Chinese display in Matplotlib and provide specific code examples.
Introduction:
Matplotlib is one of the most popular data visualization libraries in Python and is widely used in scientific computing, data analysis and other fields. However, by default, Matplotlib is not friendly to Chinese support and often displays garbled characters, causing inconvenience to users. The following will introduce several methods to solve the problem of garbled Chinese display in Matplotlib, and attach corresponding code examples.
Solution 1: Modify the font
The default font of Matplotlib is generally 'Arial', and 'Arial' does not support Chinese characters. Therefore, we can modify the default font of Matplotlib to a font library that supports Chinese, such as 'Microsoft Yahei', 'SimHei', etc. The following is a code example for modifying the font:
import matplotlib.pyplot as plt plt.rcParams['font.family'] = 'SimHei'
Solution 2: Manually specify the font
In addition to modifying the default font, we can also manually specify a specific font when drawing to solve the problem of garbled characters . The following is a code example for manually specifying fonts:
import matplotlib.pyplot as plt import matplotlib.font_manager as fm # 手动指定字体 font = fm.FontProperties(fname='C:/Windows/Fonts/msyh.ttc') # 绘图 plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25]) plt.xlabel('横轴', fontproperties=font) plt.ylabel('纵轴', fontproperties=font) plt.title('示例图', fontproperties=font) plt.show()
Solution 3: Use the system’s own fonts
Some operating systems come with font libraries that can support Chinese characters, we can also use these Font library to solve the problem of garbled characters. The following is a code example for using the system's own fonts:
import matplotlib.pyplot as plt # 使用系统自带字体 plt.rcParams['font.sans-serif'] = ['Microsoft YaHei'] # 绘图 plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25]) plt.xlabel('横轴') plt.ylabel('纵轴') plt.title('示例图') plt.show()
Solution 4: Using font caching
Matplotlib provides a font caching mechanism that can cache the fonts that need to be used into the system. to improve performance and resolve garbled characters. The following is a code example using font caching:
import matplotlib.pyplot as plt from matplotlib.font_manager import FontManager, FontProperties # 缓存字体 fm = FontManager() fp = FontProperties(family='SimHei') fm.ttflist.extend(fp.get_familyfont()) # 使用缓存的字体绘图 plt.rcParams['font.family'] = fp.get_family() plt.plot([1, 2, 3, 4, 5], [1, 4, 9, 16, 25]) plt.xlabel('横轴') plt.ylabel('纵轴') plt.title('示例图') plt.show()
Summary:
This article introduces four methods to solve the problem of garbled Chinese display in Matplotlib, and provides corresponding code examples. By modifying the font, manually specifying the font, using the system's own fonts, and using the font cache, we can easily solve the problem of garbled Chinese display in Matplotlib, allowing us to better draw Chinese charts. Hope this article is helpful to readers.
The above is the detailed content of Methods to solve the problem of Chinese garbled characters in matplotlib. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
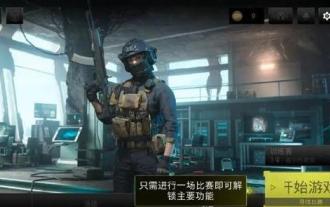
Call of Duty Warzone is a newly launched mobile game. Many players are very curious about how to set the language of this game to Chinese. In fact, it is very simple. Players only need to download the Chinese language pack, and then You can modify it after using it. The detailed content can be learned in this Chinese setting method introduction. Let us take a look together. How to set the Chinese language for the mobile game Call of Duty: Warzone 1. First enter the game and click the settings icon in the upper right corner of the interface. 2. In the menu bar that appears, find the [Download] option and click it. 3. Select [SIMPLIFIEDCHINESE] (Simplified Chinese) on this page to download the Simplified Chinese installation package. 4. Return to the settings

Excel spreadsheet is one of the office software that many people are using now. Some users, because their computer is Win11 system, so the English interface is displayed. They want to switch to the Chinese interface, but they don’t know how to operate it. To solve this problem, this issue The editor is here to answer the questions for all users. Let’s take a look at the content shared in today’s software tutorial. Tutorial for switching Excel to Chinese: 1. Enter the software and click the "File" option on the left side of the toolbar at the top of the page. 2. Select "options" from the options given below. 3. After entering the new interface, click the “language” option on the left
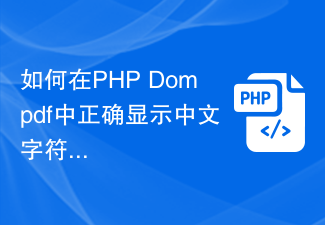
How to display Chinese characters correctly in PHPDompdf When using PHPDompdf to generate PDF files, it is a common challenge to encounter the problem of garbled Chinese characters. This is because the font library used by Dompdf by default does not contain Chinese character sets. In order to display Chinese characters correctly, we need to manually set the font of Dompdf and make sure to select a font that supports Chinese characters. Here are some specific steps and code examples to solve this problem: Step 1: Download the Chinese font file First, we need
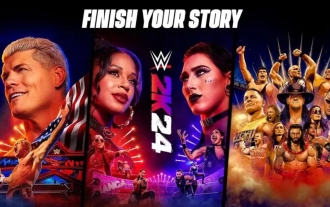
"WWE2K24" is a racing sports game created by Visual Concepts and was officially released on March 9, 2024. This game has been highly praised, and many players are eagerly interested in whether it will have a Chinese version. Unfortunately, so far, "WWE2K24" has not yet launched a Chinese language version. Will wwe2k24 be in Chinese? Answer: Chinese is not currently supported. The standard version of WWE2K24 in the Steam Chinese region is priced at 199 yuan, the deluxe version is 329 yuan, and the commemorative edition is 395 yuan. The game has relatively high configuration requirements, and there are certain standards in terms of processor, graphics card, or running memory. Official recommended configuration and minimum configuration introduction:
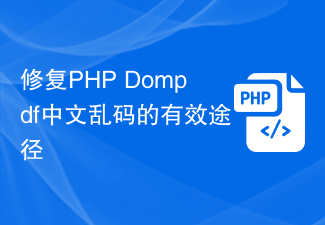
Title: An effective way to repair Chinese garbled characters in PHPDompdf. When using PHPDompdf to generate PDF documents, garbled Chinese characters are a common problem. This problem usually stems from the fact that Dompdf does not support Chinese character sets by default, resulting in Chinese content not being displayed correctly. In order to solve this problem, we need to take some effective ways to fix the Chinese garbled problem of PHPDompdf. 1. Use custom font files. An effective way to solve the problem of Chinese garbled characters in Dompdf is to use
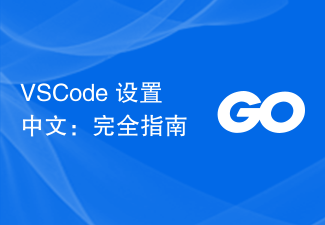
VSCode Setup in Chinese: A Complete Guide In software development, Visual Studio Code (VSCode for short) is a commonly used integrated development environment. For developers who use Chinese, setting VSCode to the Chinese interface can improve work efficiency. This article will provide you with a complete guide, detailing how to set VSCode to a Chinese interface and providing specific code examples. Step 1: Download and install the language pack. After opening VSCode, click on the left
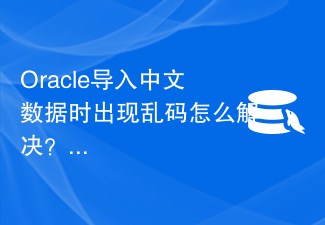
Title: Methods and code examples to solve the problem of garbled characters when importing Chinese data into Oracle. When importing Chinese data into Oracle database, garbled characters often appear. This may be due to incorrect database character set settings or encoding conversion problems during the import process. . In order to solve this problem, we can take some methods to ensure that the imported Chinese data can be displayed correctly. The following are some solutions and specific code examples: 1. Check the database character set settings In the Oracle database, the character set settings are

Tips for solving Chinese garbled characters written by PHP into txt files. With the rapid development of the Internet, PHP, as a widely used programming language, is used by more and more developers. In PHP development, it is often necessary to read and write text files, including txt files that write Chinese content. However, due to encoding format problems, sometimes the written Chinese will appear garbled. This article will introduce some techniques to solve the problem of Chinese garbled characters written into txt files by PHP, and provide specific code examples. Problem analysis in PHP, text
