


Analysis of application cases of front-end closure: What are its typical application scenarios?
Application case analysis of front-end closure: In what situations does it usually come into play?
In front-end development, closure (Closure) is a very powerful and commonly used concept. It is a special function that can access variables outside the function, and these variables will always be retained in memory. The application of closures can help us solve some common problems, such as data privatization, variable storage, etc. So, in what situations do closures come into play? The following will be analyzed through specific code examples.
- Data privatization
Closures can help us privatize data, avoid the abuse of global variables, and improve the maintainability and security of the code. Here is an example:
function createCounter() { let count = 0; // 私有变量 function increment() { count++; console.log(count); } function decrement() { count--; console.log(count); } return { increment: increment, decrement: decrement }; } let counter = createCounter(); counter.increment(); // 输出:1 counter.decrement(); // 输出:0
In this example, we create a counter using a closure. The counter logic is encapsulated inside a function and is accessed by returning an object containing increment and decrement functions. Since the count
variable is encapsulated inside the closure, it cannot be accessed directly from the outside, ensuring the privatization of the variable.
- Memory calculation
Closures can also help us implement memory calculation and improve the execution efficiency of the code. Memoization is a process of caching calculation results so that the next time the same input is used, the cached results can be returned directly to avoid repeated calculations. The following is an example of a Fibonacci sequence:
function memoize(f) { let cache = {}; return function (n) { if (cache[n] !== undefined) { return cache[n]; } let result = f(n); cache[n] = result; return result; }; } let fibonacci = memoize(function (n) { if (n === 0 || n === 1) { return n; } return fibonacci(n - 1) + fibonacci(n - 2); }); console.log(fibonacci(5)); // 输出:5
In this example, we use closures to create a memoize function memoize
. memoize
Accepts a function f
as a parameter and returns a new function. The new function first checks whether the calculation result has been cached. If so, it returns the cached result directly; if not, it calculates the result by calling function f
and caches the result. In this way, if the same input is encountered in subsequent calls, the cached results can be returned directly, avoiding repeated calculations.
- Modular development
Closures can also help us implement modular development, organize code by function, and improve the maintainability and reusability of code. Here is a simple modularization example:
var module = (function () { var privateVariable = 10; function privateFunction() { console.log('私有函数执行'); } return { publicVariable: 20, publicFunction: function () { console.log('公共函数执行'); privateVariable++; privateFunction(); } }; })(); console.log(module.publicVariable); // 输出:20 module.publicFunction(); // 输出:公共函数执行 私有函数执行
In this example, we create a module using closures. The variables and functions inside the module are encapsulated inside the closure and cannot be directly accessed from the outside, thereby achieving the purpose of information hiding and function encapsulation. At the same time, by returning an object containing public variables and public functions, we can access and use these public members externally.
Summary:
Closures are widely used in front-end development. In addition to data privatization, memorized computing and modular development in the above examples, closures can also be used in event processing, asynchronous programming and other scenarios. By properly applying closures, we can better organize and manage the code, improve the readability and maintainability of the code, and also improve the performance of the code. Therefore, understanding and mastering the application of closures is an essential skill for every front-end developer.
The above is the detailed content of Analysis of application cases of front-end closure: What are its typical application scenarios?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


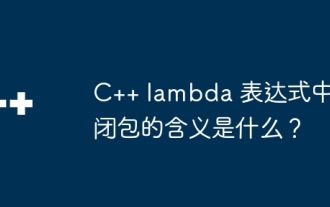
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
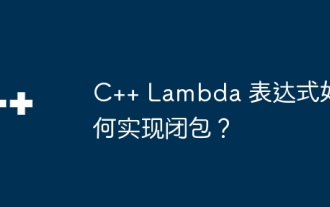
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
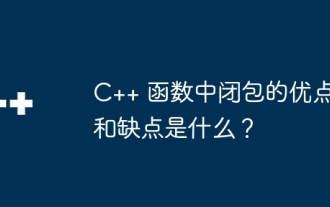
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.
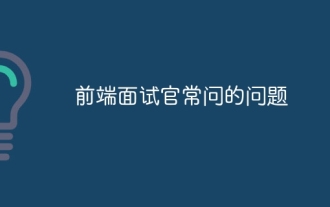
In front-end development interviews, common questions cover a wide range of topics, including HTML/CSS basics, JavaScript basics, frameworks and libraries, project experience, algorithms and data structures, performance optimization, cross-domain requests, front-end engineering, design patterns, and new technologies and trends. . Interviewer questions are designed to assess the candidate's technical skills, project experience, and understanding of industry trends. Therefore, candidates should be fully prepared in these areas to demonstrate their abilities and expertise.
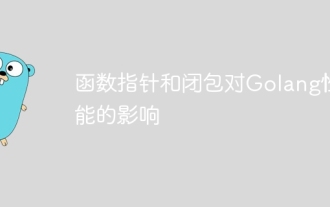
The impact of function pointers and closures on Go performance is as follows: Function pointers: Slightly slower than direct calls, but improves readability and reusability. Closures: Typically slower, but encapsulate data and behavior. Practical case: Function pointers can optimize sorting algorithms, and closures can create event handlers, but they will bring performance losses.
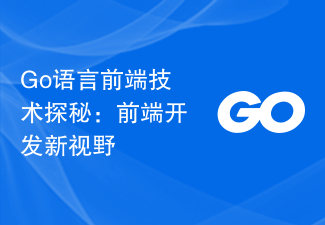
As a fast and efficient programming language, Go language is widely popular in the field of back-end development. However, few people associate Go language with front-end development. In fact, using Go language for front-end development can not only improve efficiency, but also bring new horizons to developers. This article will explore the possibility of using the Go language for front-end development and provide specific code examples to help readers better understand this area. In traditional front-end development, JavaScript, HTML, and CSS are often used to build user interfaces
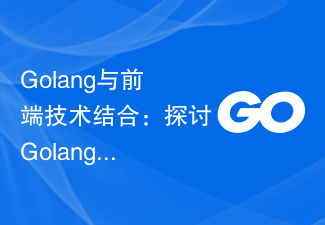
Combination of Golang and front-end technology: To explore how Golang plays a role in the front-end field, specific code examples are needed. With the rapid development of the Internet and mobile applications, front-end technology has become increasingly important. In this field, Golang, as a powerful back-end programming language, can also play an important role. This article will explore how Golang is combined with front-end technology and demonstrate its potential in the front-end field through specific code examples. The role of Golang in the front-end field is as an efficient, concise and easy-to-learn
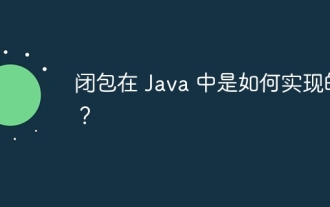
Closures in Java allow inner functions to access outer scope variables even if the outer function has exited. Implemented through anonymous inner classes, the inner class holds a reference to the outer class and keeps the outer variables active. Closures increase code flexibility, but you need to be aware of the risk of memory leaks because references to external variables by anonymous inner classes keep those variables alive.
