


Reveal the core technologies of Java development and create outstanding developers
To deeply explore the core technology of Java development and become an outstanding developer, you need specific code examples
In recent years, Java has been widely used in enterprise-level development Programming language has become one of the first choices for developers. Java's powerful cross-platform nature, object-oriented design ideas, rich class libraries, and mature and stable development environment make it play an important role in the development of large-scale projects. To become an excellent Java developer, you not only need to master its syntax and basic knowledge, but also need to have an in-depth understanding of its core technology. This article will deeply explore the core technologies of Java development and help readers better understand through specific code examples.
1. Multi-threading technology
Multi-threading is one of the very important core technologies in Java development. In concurrent programming, multi-threading can significantly improve program performance and response speed. Below is a simple multithreading example showing how to create and start threads.
public class ThreadExample extends Thread { public void run() { System.out.println("Thread is running"); } public static void main(String[] args) { ThreadExample thread = new ThreadExample(); thread.start(); } }
The above code creates a custom thread class ThreadExample that inherits from the Thread class and overrides the run() method. In the main() method, we create an instance of ThreadExample and start the thread through the start() method. When running, the program will output "Thread is running".
2. Exception handling
Exception handling is another important core technology in Java development. Proper handling of exceptions can ensure the stability and robustness of the program. Below is a simple exception handling example showing how to catch and handle exceptions.
public class ExceptionExample { public static void main(String[] args) { try { int result = divide(10, 0); System.out.println(result); } catch (ArithmeticException e) { System.out.println("Divide by zero error"); } } public static int divide(int a, int b) { return a / b; } }
In the above code, we define a divide() method for division operations. In the main() method, we try to divide 10 by 0 and print the result. Since division cannot be divided by 0, an ArithmeticException will be thrown. By catching this exception in a try-catch block and outputting a custom prompt message, we can avoid program crashes.
3. Collection Framework
The collection framework is a data structure commonly used in Java development. It provides various collection classes to store and manipulate a set of objects. Below is a simple collection framework example that shows how to use a List collection to store and traverse data.
import java.util.ArrayList; import java.util.List; public class CollectionExample { public static void main(String[] args) { List<String> list = new ArrayList<>(); list.add("Java"); list.add("Python"); list.add("C++"); for (String item : list) { System.out.println(item); } } }
In the above code, we create an ArrayList instance and use the add() method to add elements to the list. With a for-each loop, we can iterate through each element in the list and print it out.
Summary:
This article deeply explores the core technology of Java development through specific code examples. Multi-threading technology can improve the performance and response speed of the program, exception handling can ensure the stability and robustness of the program, and the collection framework can conveniently store and operate a group of objects. By learning and in-depth understanding of these core technologies, we can become an excellent Java developer. In the future, Java, as a powerful and stable programming language, will continue to play an important role in the development field.
The above is the detailed content of Reveal the core technologies of Java development and create outstanding developers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
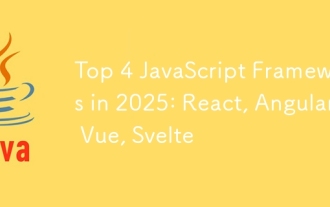
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
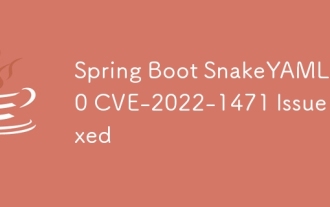
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
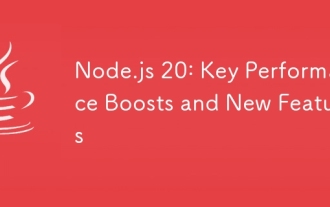
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
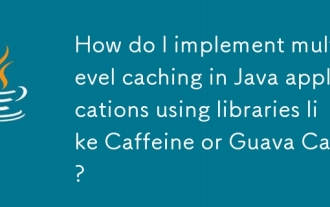
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
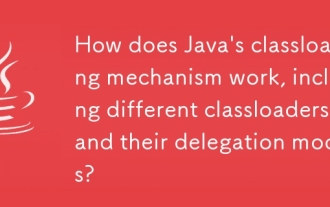
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
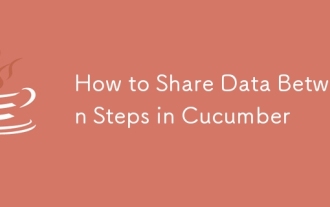
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
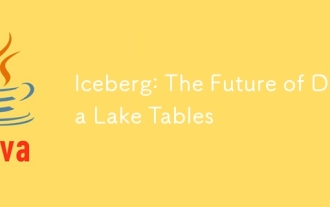
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
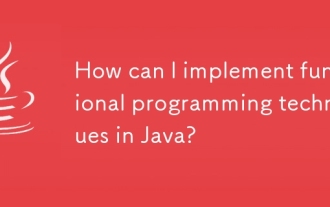
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
