Analyze the uses and applications of closures in the Vue framework
Application scenario analysis of closures in the Vue framework
Introduction:
As a modern front-end framework, the Vue framework is very flexible and convenient to use. In the development process of Vue, we often use closures. Closure is an important concept in JavaScript. It not only helps us implement dynamic scope, but can also be used to manage the scope of variables, protect private variables, etc. This article will focus on the application scenarios of closures in the Vue framework, and analyze them with specific code examples.
- Implementation of dynamic scope
The directive in the Vue framework is one of the very important functions. Directives can dynamically change the behavior and style of DOM elements. In Vue, we often use the v-for directive to render lists. When we access external variables in the callback function of the v-for directive, we need to use closures to implement dynamic scope.
Code example:
<template> <div> <ul> <li v-for="(item, index) in itemList" :key="index"> <button @click="handleClick(index)">{{ item }}</button> </li> </ul> <p>当前点击按钮的索引:{{ currentIndex }}</p> </div> </template> <script> export default { data() { return { itemList: [1, 2, 3, 4, 5], currentIndex: -1 }; }, methods: { handleClick(index) { // 使用闭包,保存当前的索引值 this.currentIndex = index; } } }; </script>
In the above code, we use closure technology to save the current index value in the handleClick method so that it can be directly accessed in the template. This achieves dynamic scope, and the currentIndex value is automatically updated when different buttons are clicked.
- Protect private variables
In Vue component development, we often need to define some private variables to record the status within the component. These private variables are not expected to be directly accessed and modified by external components, and closures can be used to easily achieve this requirement.
Code example:
<template> <div> <button @click="handleClick">Click me</button> <p>{{ count }}</p> </div> </template> <script> export default { data() { return { count: 0 }; }, methods: { handleClick() { let self = this; // 使用闭包,保护count变量 (function() { self.count++; })(); } } }; </script>
In the above code, we use an immediate execution function to assign the this value of its own component to the variable self, and then execute the count variable through closure Revise. In this way, the protection of private variables is achieved, and external components cannot directly access and modify the value of count.
Conclusion:
In the Vue framework, closures have many application scenarios, and dynamic scope and protecting private variables are just two examples. Closures can help us manage the scope of variables more flexibly during the development process of Vue, and increase the maintainability and security of the code. I hope this article will help you understand the application of closures in the Vue framework.
The above is the detailed content of Analyze the uses and applications of closures in the Vue framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


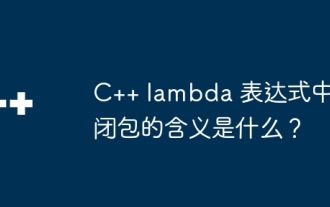
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
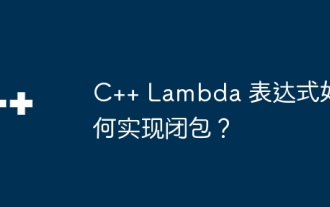
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
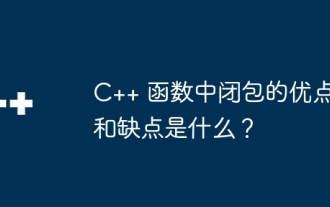
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.
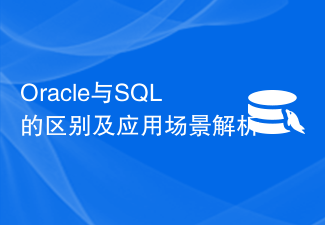
The difference between Oracle and SQL and analysis of application scenarios In the database field, Oracle and SQL are two frequently mentioned terms. Oracle is a relational database management system (RDBMS), and SQL (StructuredQueryLanguage) is a standardized language for managing relational databases. While they are somewhat related, there are also some significant differences. First of all, by definition, Oracle is a specific database management system, consisting of
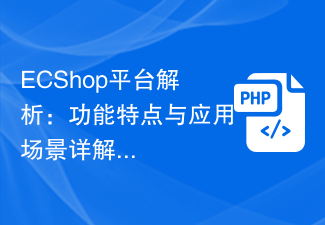
ECShop platform analysis: Detailed explanation of functional features and application scenarios ECShop is an open source e-commerce system developed based on PHP+MySQL. It has powerful functional features and a wide range of application scenarios. This article will analyze the functional features of the ECShop platform in detail, and combine it with specific code examples to explore its application in different scenarios. Features 1.1 Lightweight and high-performance ECShop adopts a lightweight architecture design, with streamlined and efficient code and fast running speed, making it suitable for small and medium-sized e-commerce websites. It adopts the MVC pattern

The Go language is suitable for a variety of scenarios, including back-end development, microservice architecture, cloud computing, big data processing, machine learning, and building RESTful APIs. Among them, the simple steps to build a RESTful API using Go include: setting up the router, defining the processing function, obtaining the data and encoding it into JSON, and writing the response.
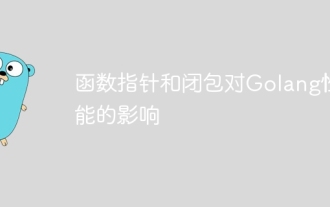
The impact of function pointers and closures on Go performance is as follows: Function pointers: Slightly slower than direct calls, but improves readability and reusability. Closures: Typically slower, but encapsulate data and behavior. Practical case: Function pointers can optimize sorting algorithms, and closures can create event handlers, but they will bring performance losses.
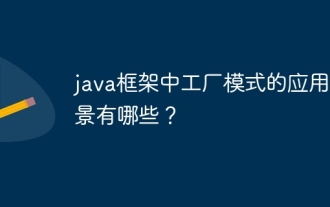
The factory pattern is used to decouple the creation process of objects and encapsulate them in factory classes to decouple them from concrete classes. In the Java framework, the factory pattern is used to: Create complex objects (such as beans in Spring) Provide object isolation, enhance testability and maintainability Support extensions, increase support for new object types by adding new factory classes
