


Analyze the characteristics of modules using closures in the Vue framework
Module analysis using closures in the Vue framework
In the Vue framework, closures are a very common programming technology that can help us modularize Organize and encapsulate code. This article will use specific code examples to analyze how to use closures for modular development in the Vue framework.
First let's look at a simple example. Suppose we have a Vue component that needs to display a counter in the template, and the function of increasing the count can be implemented after clicking the button. We can use closures to achieve this function, the code is as follows:
<template> <div> <p>{{ count }}</p> <button @click="increment">增加</button> </div> </template> <script> export default { data() { return { count: 0 }; }, methods: { increment: (function() { let count = 0; return function() { this.count++; }; })() } }; </script>
In the above code, we use an immediate execution function to create a closure. This closure contains a local variable count
, which is used to save the value of the counter. By assigning the function in the closure to the increment
method, we implement the function of incrementing the count when the button is clicked.
By using closures, we can effectively encapsulate variables and bind them to a specific function. This flexibility allows us to implement a more modular approach to development.
In addition to using closures in methods, we can also use closures in computed properties of Vue components. The following is a sample code for a computed property:
<template> <div> <p>{{ upperCaseText }}</p> </div> </template> <script> export default { data() { return { text: 'hello world' }; }, computed: { upperCaseText: function() { return (function() { let text = this.text; return text.toUpperCase(); })(); } } }; </script>
In the above code, we use a closure to encapsulate the computed property function. The local variable text
in the closure stores the text we need to calculate. By immediately executing the result returned by the function, we implement the functionality of converting text to uppercase.
In summary, the modular development method using closures in the Vue framework can help us encapsulate and hide some local variables and improve the maintainability and reusability of the code. Through closures, we can better organize code, achieve modular development, and reduce the risk of variable pollution.
Of course, you also need to pay attention to avoid memory leaks when using closures. External variables referenced in closures may cause memory leaks if they are saved for a long time without being released. Therefore, we need to manage variables in closures reasonably to ensure that resources are released correctly when they are not needed.
The above is an analysis of modules using closures in the Vue framework. Through specific code examples, we have seen the application scenarios of closures in Vue development. Using closures can help us better implement modular development and improve the readability and maintainability of the code. In actual development, we can use closures reasonably according to needs to better write high-quality Vue applications.
The above is the detailed content of Analyze the characteristics of modules using closures in the Vue framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


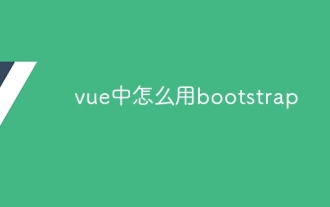
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
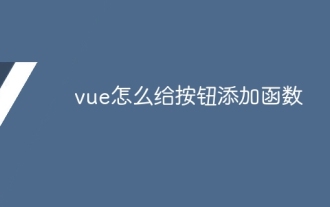
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
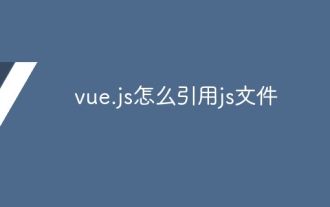
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
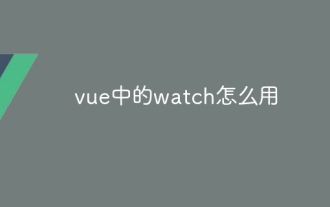
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
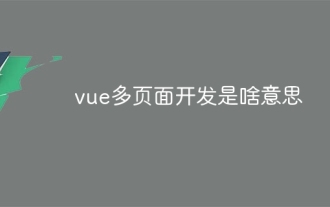
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
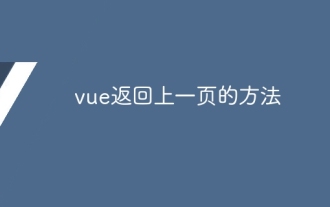
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
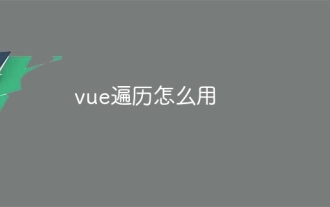
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
