How to effectively avoid memory leaks in closures?
How to prevent memory leaks in closures?
Closure is one of the most powerful features in JavaScript, which can realize function nesting and data encapsulation. However, closures are also prone to memory leaks, especially when dealing with asynchronous and timers. This article explains how to prevent memory leaks in closures and provides specific code examples.
Memory leaks usually occur when an object is no longer needed, but the memory it occupies cannot be released for some reason. In closures, when functions reference external variables that are no longer needed, memory leaks may occur.
The following are some common situations where closures cause memory leaks:
- The timer is not cleaned up: When using setTimeout or setInterval to create a timer, if the closure refers to an external Variables, even if the timer has completed execution, the referenced variables cannot be garbage collected.
- Event listener is not removed: If a closure is used as a callback function for an event, and the event listener is not removed correctly, the closure will still be retained in memory.
- Asynchronous requests are not canceled: If a closure is used to handle a callback function for an asynchronous request, and the request fails to be canceled or destroyed in time, the closure will continue to retain its reference.
In order to avoid the occurrence of memory leaks, we can take the following methods:
- Cancel the timer: After using the timer function to create a timer, make sure not to Clean the timer in time when necessary. You can use the clearTimeout or clearInterval function to cancel the timer.
The sample code is as follows:
function startTimer() { var count = 0; var timer = setInterval(function() { count++; console.log(count); if (count >= 10) { clearInterval(timer); } }, 1000); } startTimer();
In the above code, we added a conditional judgment in the callback function of the timer. When the count reaches 10, the timer is cleared.
- Remove event listeners: After adding an event listener using addEventListener or jQuery's on function, make sure to properly remove the event listener when it is no longer needed.
The sample code is as follows:
var button = document.getElementById('myButton'); function handleClick() { console.log('Button clicked!'); } button.addEventListener('click', handleClick); // do something... button.removeEventListener('click', handleClick);
In the above code, we passed in the same callback function when calling the removeEventListener function to ensure that the event listener is removed correctly.
- Cancel asynchronous requests: When using asynchronous requests, make sure to cancel or destroy the request in time to prevent the closure from continuing to retain its reference.
The sample code is as follows:
function fetchData() { var xhr = new XMLHttpRequest(); xhr.onreadystatechange = function() { if (xhr.readyState === 4) { console.log(xhr.responseText); } }; xhr.open('GET', 'https://example.com/data', true); xhr.send(); // do something... // cancel request xhr.abort(); } fetchData();
In the above code, we use the xhr.abort() function to cancel the asynchronous request.
To sum up, in order to prevent memory leaks in closures, we need to clean up resources that are no longer needed in time. These resources include timers, event listeners, asynchronous requests, etc. As long as these resources are canceled or destroyed correctly, memory leaks can be avoided.
I hope the code examples provided in this article will be helpful to you and allow you to better understand how to prevent memory leaks in closures.
The above is the detailed content of How to effectively avoid memory leaks in closures?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


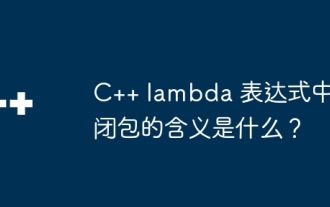
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
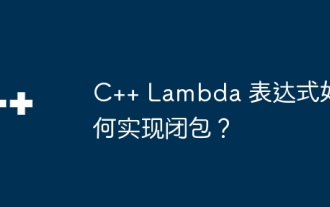
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
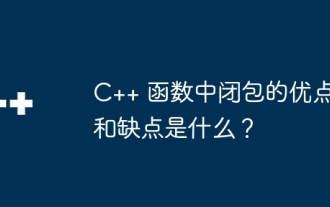
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.
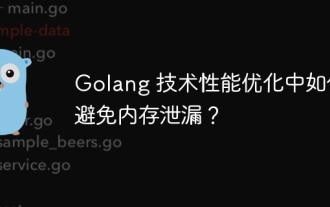
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
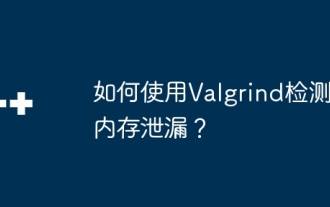
Valgrind detects memory leaks and errors by simulating memory allocation and deallocation. To use it, follow these steps: Install Valgrind: Download and install the version for your operating system from the official website. Compile the program: Compile the program using Valgrind flags (such as gcc-g-omyprogrammyprogram.c-lstdc++). Analyze the program: Use the valgrind--leak-check=fullmyprogram command to analyze the compiled program. Check the output: Valgrind will generate a report after the program execution, showing memory leaks and error messages.
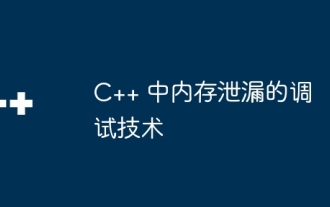
A memory leak in C++ means that the program allocates memory but forgets to release it, causing the memory to not be reused. Debugging techniques include using debuggers (such as Valgrind, GDB), inserting assertions, and using memory leak detector libraries (such as Boost.LeakDetector, MemorySanitizer). It demonstrates the use of Valgrind to detect memory leaks through practical cases, and proposes best practices to avoid memory leaks, including: always releasing allocated memory, using smart pointers, using memory management libraries, and performing regular memory checks.
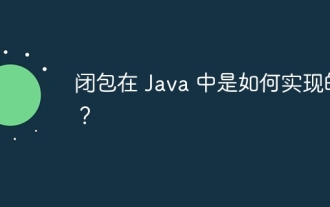
Closures in Java allow inner functions to access outer scope variables even if the outer function has exited. Implemented through anonymous inner classes, the inner class holds a reference to the outer class and keeps the outer variables active. Closures increase code flexibility, but you need to be aware of the risk of memory leaks because references to external variables by anonymous inner classes keep those variables alive.

Go language function closures play a vital role in unit testing: Capturing values: Closures can access variables in the outer scope, allowing test parameters to be captured and reused in nested functions. Simplify test code: By capturing values, closures simplify test code by eliminating the need to repeatedly set parameters for each loop. Improve readability: Use closures to organize test logic, making test code clearer and easier to read.
