


A practical guide to optimizing Python multi-threaded applications
Practical Guide: How to optimize Python multi-threaded applications, specific code examples are required
Introduction:
As computer performance continues to improve, multi-threaded applications It has become one of the important means for developers to improve the efficiency of program operation. As a high-level programming language that is easy to learn and use, Python also provides support for multi-threaded programming. However, in practice, we often encounter the problem of inefficient multi-threaded applications. This article will start from the perspective of optimizing Python multi-threaded applications and provide you with some practical tips and specific code examples.
1. Reasonable design of the number of threads
In multi-threaded applications, the reasonable design of the number of threads has a decisive impact on the performance of the program. Too many threads will increase the overhead of thread switching, while too few threads will not make full use of system resources. Therefore, we need to determine the number of threads reasonably.
Sample code:
import threading def worker(): # 线程执行的任务 print("执行任务") def main(): thread_num = 5 # 线程数量 threads = [] for i in range(thread_num): t = threading.Thread(target=worker) t.start() threads.append(t) for t in threads: t.join() if __name__ == "__main__": main()
In the above code example, we have created 5 threads to perform the task. By adjusting the value of thread_num
, you can flexibly control the number of threads.
2. Reasonable division of tasks
In multi-threaded applications, task division is also the key to optimization. Reasonable division of tasks can balance the load among various threads and give full play to the advantages of multi-threaded parallel computing.
Sample code:
import threading def worker(start, end): # 线程执行的任务 for i in range(start, end): print("任务{}".format(i)) def main(): total_tasks = 50 # 总任务数 thread_num = 5 # 线程数量 threads = [] tasks_per_thread = total_tasks // thread_num # 每个线程处理的任务数 for i in range(thread_num): start = i * tasks_per_thread end = (i + 1) * tasks_per_thread if i == thread_num - 1: # 最后一个线程处理剩余的任务 end = total_tasks t = threading.Thread(target=worker, args=(start, end)) t.start() threads.append(t) for t in threads: t.join() if __name__ == "__main__": main()
In the above code example, we divide the total number of tasks into 5 parts and assign them to 5 threads for processing. This ensures that each thread is responsible for a relatively balanced task.
3. Avoid shared resource competition
In multi-threaded applications, shared resource competition is a common problem. When multiple threads perform read and write operations on shared resources at the same time, data inconsistency and performance degradation may result. Therefore, we need to take measures to avoid contention for shared resources.
Sample code:
import threading shared_counter = 0 # 共享计数器 lock = threading.Lock() # 锁对象 def worker(): global shared_counter for _ in range(10000): with lock: # 使用锁来保证对共享资源的互斥访问 shared_counter += 1 def main(): thread_num = 5 # 线程数量 threads = [] for _ in range(thread_num): t = threading.Thread(target=worker) t.start() threads.append(t) for t in threads: t.join() print("共享计数器的值为:", shared_counter) if __name__ == "__main__": main()
In the above code example, we used threading.Lock()
to create a lock object and access the shared resource in the code block with lock:
is used to achieve mutually exclusive access to shared resources and ensure data consistency.
Conclusion:
Optimizing Python multi-threaded applications not only requires good design and reasonable division of tasks, but also requires a reasonable setting of the number of threads to avoid competition for shared resources. This article provides practical tips and methods through specific code examples, hoping to help everyone optimize Python multi-threaded applications in practice. At the same time, it is worth noting that optimizing multi-threaded applications is not a one-time thing and needs to be adjusted and optimized according to specific circumstances.
The above is the detailed content of A practical guide to optimizing Python multi-threaded applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
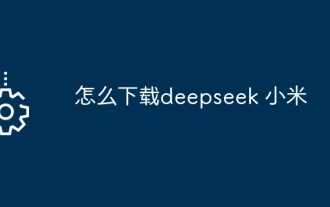
How to download DeepSeek Xiaomi? Search for "DeepSeek" in the Xiaomi App Store. If it is not found, continue to step 2. Identify your needs (search files, data analysis), and find the corresponding tools (such as file managers, data analysis software) that include DeepSeek functions.
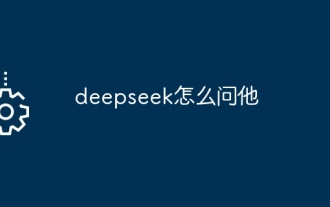
The key to using DeepSeek effectively is to ask questions clearly: express the questions directly and specifically. Provide specific details and background information. For complex inquiries, multiple angles and refute opinions are included. Focus on specific aspects, such as performance bottlenecks in code. Keep a critical thinking about the answers you get and make judgments based on your expertise.
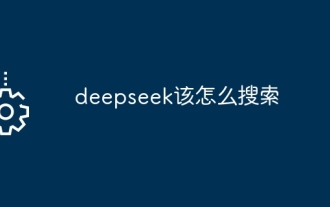
Just use the search function that comes with DeepSeek. Its powerful semantic analysis algorithm can accurately understand the search intention and provide relevant information. However, for searches that are unpopular, latest information or problems that need to be considered, it is necessary to adjust keywords or use more specific descriptions, combine them with other real-time information sources, and understand that DeepSeek is just a tool that requires active, clear and refined search strategies.
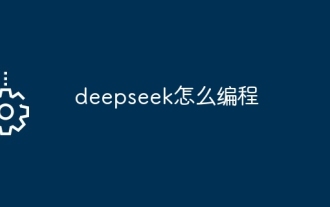
DeepSeek is not a programming language, but a deep search concept. Implementing DeepSeek requires selection based on existing languages. For different application scenarios, it is necessary to choose the appropriate language and algorithms, and combine machine learning technology. Code quality, maintainability, and testing are crucial. Only by choosing the right programming language, algorithms and tools according to your needs and writing high-quality code can DeepSeek be successfully implemented.
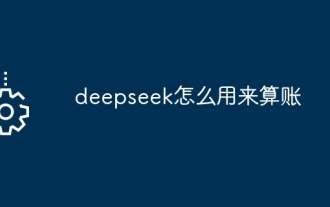
Question: Is DeepSeek available for accounting? Answer: No, it is a data mining and analysis tool that can be used to analyze financial data, but it does not have the accounting record and report generation functions of accounting software. Using DeepSeek to analyze financial data requires writing code to process data with knowledge of data structures, algorithms, and DeepSeek APIs to consider potential problems (e.g. programming knowledge, learning curves, data quality)
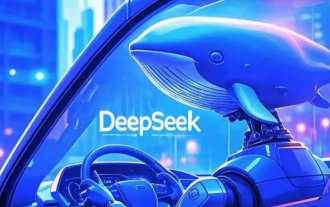
Detailed explanation of DeepSeekAPI access and call: Quick Start Guide This article will guide you in detail how to access and call DeepSeekAPI, helping you easily use powerful AI models. Step 1: Get the API key to access the DeepSeek official website and click on the "Open Platform" in the upper right corner. You will get a certain number of free tokens (used to measure API usage). In the menu on the left, click "APIKeys" and then click "Create APIkey". Name your APIkey (for example, "test") and copy the generated key right away. Be sure to save this key properly, as it will only be displayed once
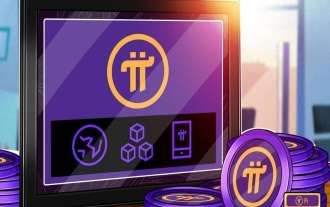
PiNetwork is about to launch PiBank, a revolutionary mobile banking platform! PiNetwork today released a major update on Elmahrosa (Face) PIMISRBank, referred to as PiBank, which perfectly integrates traditional banking services with PiNetwork cryptocurrency functions to realize the atomic exchange of fiat currencies and cryptocurrencies (supports the swap between fiat currencies such as the US dollar, euro, and Indonesian rupiah with cryptocurrencies such as PiCoin, USDT, and USDC). What is the charm of PiBank? Let's find out! PiBank's main functions: One-stop management of bank accounts and cryptocurrency assets. Support real-time transactions and adopt biospecies

Here are some popular AI slicing tools: TensorFlow DataSetPyTorch DataLoaderDaskCuPyscikit-imageOpenCVKeras ImageDataGenerator
