In-depth understanding of different data types in Go language
To understand the different data types in Go language, you need specific code examples
As a statically typed programming language, Go language has rich data types, including basic Data types and composite data types. Mastering the characteristics and usage of different data types is crucial to writing efficient and accurate programs. The following will introduce several common data types in Go language with specific code examples.
- Basic data types:
Go language provides some basic data types, including integer, floating point, Boolean and string types.
Integer type:
package main import "fmt" func main() { var a int = 10 fmt.Printf("整型变量a的值为:%d ", a) // 其他整型类型 var b int8 = 127 var c int16 = 32767 var d int32 = 2147483647 var e int64 = 9223372036854775807 fmt.Printf("整型变量b的值为:%d ", b) fmt.Printf("整型变量c的值为:%d ", c) fmt.Printf("整型变量d的值为:%d ", d) fmt.Printf("整型变量e的值为:%d ", e) }
Floating point type:
package main import "fmt" func main() { var f1 float32 = 3.14159 var f2 float64 = 3.1415926535897932384626433 fmt.Printf("浮点型变量f1的值为:%f ", f1) fmt.Printf("浮点型变量f2的值为:%f ", f2) }
Boolean type:
package main import "fmt" func main() { var b1 bool = true var b2 bool = false fmt.Printf("布尔型变量b1的值为:%t ", b1) fmt.Printf("布尔型变量b2的值为:%t ", b2) }
String type:
package main import "fmt" func main() { var str1 string = "Hello, Go" str2 := "Hello, 世界" fmt.Printf("字符串str1的值为:%s ", str1) fmt.Printf("字符串str2的值为:%s ", str2) }
- Composite data types
In addition to basic data types, the Go language also provides some composite data types, including arrays, slices, dictionaries, and structures.
Array:
package main import "fmt" func main() { var arr [5]int arr[0] = 10 arr[1] = 20 arr[2] = 30 arr[3] = 40 arr[4] = 50 fmt.Println("数组arr的值为:", arr) }
Slice:
package main import "fmt" func main() { var slice []int slice = make([]int, 5) slice[0] = 10 slice[1] = 20 slice[2] = 30 slice[3] = 40 slice[4] = 50 fmt.Println("切片slice的值为:", slice) }
Dictionary:
package main import "fmt" func main() { var dict map[string]int dict = make(map[string]int) dict["a"] = 10 dict["b"] = 20 dict["c"] = 30 fmt.Println("字典dict的值为:", dict) }
Structure:
package main import "fmt" type Person struct { Name string Age int } func main() { var p1 Person p1.Name = "Tom" p1.Age = 18 fmt.Printf("结构体p1的值为:%v ", p1) }
These code examples cover Learn about several common data types in Go language and how to use them. By understanding and mastering these data types, I believe readers can write Go programs more effectively. Of course, the Go language also provides other rich data types, and readers can consult relevant materials for in-depth study.
The above is the detailed content of In-depth understanding of different data types in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


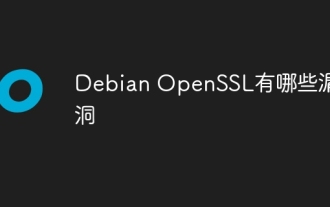
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
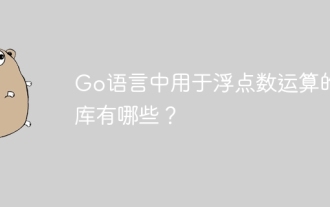
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
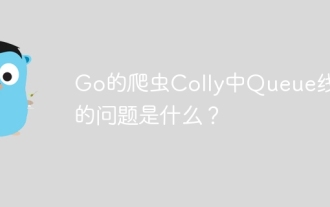
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
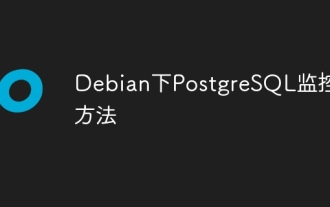
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
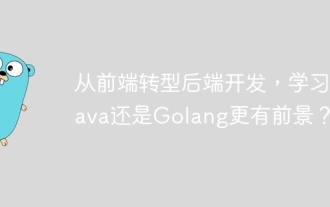
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
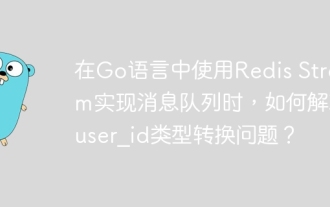
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
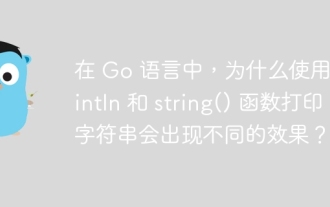
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
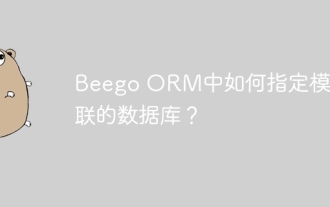
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
