What are the programming methods for delayed execution?
What are the programming methods of delay function
1. Call the API SetTimer() function of the system timer to implement delay, and you need to include the header file
2. To achieve delay by cyclically calling the clock() function to read the program running time, the header file needs to be included
3. By calling the Sleep() function, you need to include the header file
The delay accuracy that the above functions can achieve is about 10ms
void delay(double second)
{
LARGE_INTEGER litmp;
LONGLONG QPart1, QPart2;
double dfMinus, dfFreq, dfTim;
QueryPerformanceFrequency(&litmp);
dfFreq = (double)litmp.QuadPart; // Get the clock frequency of the counter
QueryPerformanceCounter(&litmp);
QPart1 = litmp.QuadPart; // Get the initial value
do
{
QueryPerformanceCounter(&litmp);
QPart2 = litmp.QuadPart; //Get the abort value
dfMinus = (double)(QPart2 - QPart1);
dfTim = dfMinus / dfFreq; // Get the corresponding time value in seconds
}
while(dfTim
}
How to postpone the execution of a function in HTML5
1.[self performSelector:@selector (function name) withObject:nil afterDelay:5.0f]
Condition: In the main thread of uiviewController
2.[NSTimerscheduledTimerWithTimeInterval:5.0f target:self selector:@selector (function name) userInfo:nil repeats:NO];
In the specific function, execute the code, and after the execution is completed, call the NSTimer invalidate method to destroy the timer
3.[NSThread sleepForTimeInterval:5.0f];
[Method to delay execution];
Conditions: Main thread or sub-thread can be used
How to delay the execution of specified commands in bat
First of all, bat delay refers to executing a command and delaying it for a period of time before proceeding with the next command. Under cmd or in batch processing, the following four methods are often used for time delay:
1. Use the ping command to delay.
Example 1:
@echo off
echo before delay: %time%
ping /n 3 127.0.0.1 >nul
echo after delay: %time%
pause
2. Use the for command to delay.
Example 2:
@echo off
echo before delay: %time%
for /l %%i in (1,1,5000) do echo %%i>nul
echo after delay: %time%
pause
3. Use vbs delay function, with millisecond accuracy and error within 1000 milliseconds.
Example 3:
@echo off
echo %time%
call :delay 5000
echo %time%
pause
exit
:delay
echo WScript.Sleep %1>delay.vbs
CScript //B delay.vbs
del delay.vbs
goto :eof
4. Only use batch processing commands to achieve any time delay, with an accuracy of 10 milliseconds and an error of 50 milliseconds. Only batch processing commands can be used to achieve delay operations.
Example 4:
@echo off
set /p delay=Please enter the number of milliseconds to be delayed:
set TotalTime=0
set NowTime=%time%
:: Reading starting time, the time format is: 13:01:05.95
echo program start time: %NowTime%
:delay_continue
set /a minute1=1%NowTime:~3,2%-100
:: Read the starting time in minutes
set /a second1=1%NowTime:~-5,2%%NowTime:~-2%0-100000
:: Convert the starting time seconds to milliseconds
set NowTime=%time%
set /a minute2=1%NowTime:~3,2%-100
:: Read the minutes of the current time
set /a second2=1%NowTime:~-5,2%%NowTime:~-2%0-100000
:: Convert the seconds of the current time to milliseconds
set /a TotalTime =(%minute2%-%minute1% 60)%`*60000 %second2%-%second1%
if %TotalTime% lss �lay% goto delay_continue
echo program end time: %time%
echo set delay time: �lay% milliseconds
echo actual delay time: %TotalTime% milliseconds
pause
I hope the above methods can help you solve the problem satisfactorily!
The above is the detailed content of What are the programming methods for delayed execution?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
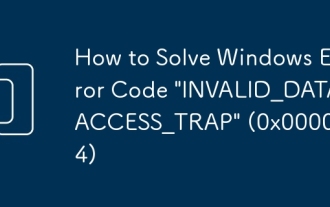
This article addresses the Windows "INVALID_DATA_ACCESS_TRAP" (0x00000004) error, a critical BSOD. It explores common causes like faulty drivers, hardware malfunctions (RAM, hard drive), software conflicts, overclocking, and malware. Trou

This article provides practical tips for maintaining ENE SYS systems. It addresses common issues like overheating and data corruption, offering preventative measures such as regular cleaning, backups, and software updates. A tailored maintenance s
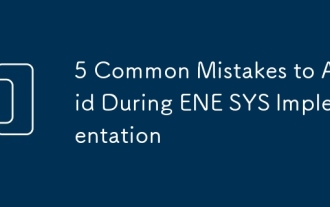
This article identifies five common pitfalls in ENE SYS implementation: insufficient planning, inadequate user training, improper data migration, neglecting security, and insufficient testing. These errors can lead to project delays, system failures
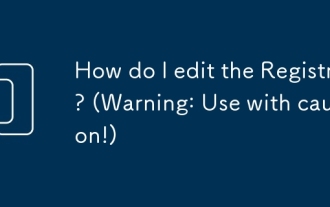
Article discusses editing Windows Registry, precautions, backup methods, and potential issues from incorrect edits. Main issue: risks of system instability and data loss from improper changes.
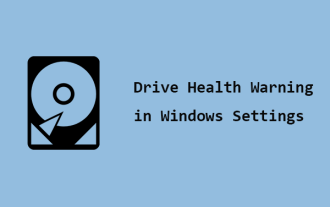
What does the drive health warning in Windows Settings mean and what should you do when you receive the disk warning? Read this php.cn tutorial to get step-by-step instructions to cope with this situation.
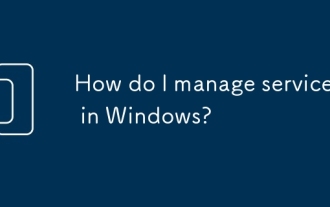
Article discusses managing Windows services for system health, including starting, stopping, restarting services, and best practices for stability.

This article identifies ene.sys as a Realtek High Definition Audio driver component. It details its function in managing audio hardware, emphasizing its crucial role in audio functionality. The article also guides users on verifying its legitimacy
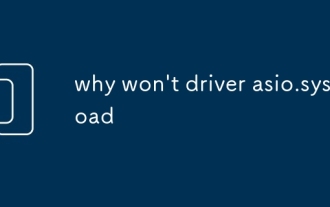
This article addresses the failure of the Windows asio.sys audio driver. Common causes include corrupted system files, hardware/driver incompatibility, software conflicts, registry issues, and malware. Troubleshooting involves SFC scans, driver upda
