Master the skills of using Golang packages
In-depth understanding of how to use Golang packages requires specific code examples
In the Go language, a package is the basic unit for organizing and managing code. A package can contain multiple Go source files (with .go extension), which together form a functional unit. The use of packages allows us to better organize code, improve code reusability, and facilitate team collaboration. This article will introduce how to deeply understand the use of Golang packages and provide specific code examples.
- Import of package
In Go language, if you want to use code in another package, you must import this package first. Packages can be imported through the import keyword. You can import standard library packages or custom packages. For example:
import ( "fmt" "log" "github.com/example/mypackage" )
When importing a package, you can also give the imported package an alias. For example:
import ( "fmt" mylog "log" mypkg "github.com/example/mypackage" )
Using aliases can avoid package name conflicts and better express the intent of the code.
- Visibility of packages
In the Go language, identifiers (variables, functions, types, etc.) in a package can be exported (visible) or not Exported. If the identifier starts with an uppercase letter, then it is exported and can be used by other packages; if it starts with a lowercase letter, then it is unexported and can only be used inside the current package.
For example, suppose we have a package named mypackage, which defines a function and a variable:
package mypackage func Add(a, b int) int { return a + b } var Name string = "mypackage"
Use this package in another package:
package main import ( "fmt" "github.com/example/mypackage" ) func main() { sum := mypackage.Add(2, 3) fmt.Println(sum) // 输出:5 fmt.Println(mypackage.Name) // 编译错误,Name是未导出的 }
- Package initialization
In the Go language, the initialization of the package will be performed automatically when the program is executed. The initialization operation of a package will only be performed once, no matter how many times the package is imported. Packages are initialized in dependency order. For example, if package A depends on package B, then package B will be initialized before package A is initialized.
The initialization operation of a package mainly includes:
- Initialize package-level variables
- Execute package-level init function
Call The order is as follows:
- First initialize the package-level variables and initialize them in the order of declaration.
- Then execute the package-level init function in the order of declaration.
package mypackage import "fmt" var Var1 = 10 var Var2 = calculateVar2() func calculateVar2() int { return Var1 * 2 } func init() { fmt.Println("mypackage initialization") fmt.Println(Var1, Var2) } func Add(a, b int) int { return a + b }
Use this package in the main program:
package main import ( "github.com/example/mypackage" ) func main() { sum := mypackage.Add(2, 3) println(sum) // 输出:5 }
Run this program and you will get the following output:
mypackage initialization 10 20 5
- Test of the package
In the Go language, we can write test code for each package, and the test code can be placed in the same package as the normal code file. The file name of the test code must end with _test.go so that the Go language testing tool can recognize it.
The name of the test function must start with Test and receive a *testing.T type parameter. For example:
package mypackage import ( "testing" ) func TestAdd(t *testing.T) { sum := Add(2, 3) if sum != 5 { t.Errorf("Add(2, 3) = %d; want 5", sum) } }
Execute the go test command in the root directory of the package to run all tests of the package:
$ go test
If the test passes, it will output:
PASS ok mypackage 0.003s
If If a test fails, failure information will be output.
In summary, to deeply understand the use of Golang packages, you need to pay attention to the following points:
- You can give the package an alias when importing it to avoid package name conflicts.
- The visibility rules of the package should be clear, and exported identifiers can be used by other packages.
- The initialization of packages is performed in dependency order.
- You can write test code for each package and run the test through the go test command.
I hope that through the introduction of this article, readers will have a deeper understanding of how to use Golang packages and be able to use them flexibly in actual development.
The above is the detailed content of Master the skills of using Golang packages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


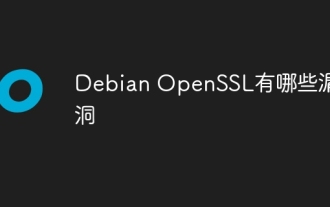
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
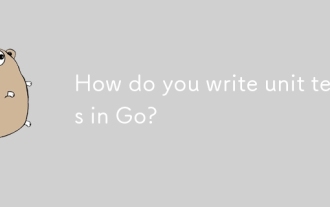
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
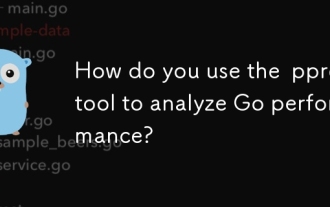
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
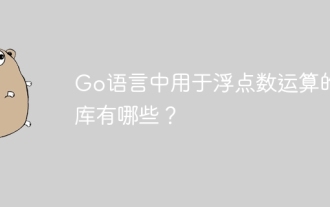
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
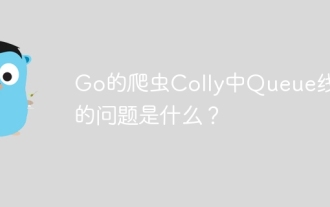
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
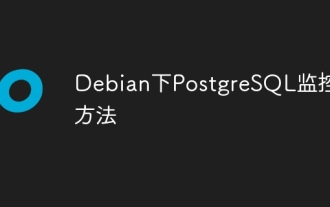
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
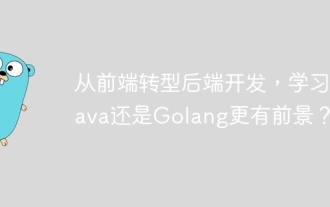
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
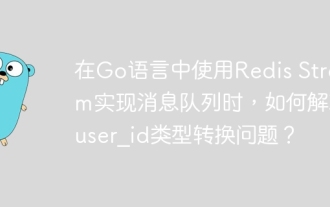
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
