


Learn to use five different data submission methods to implement Ajax
Mastering the five data submission methods of Ajax requires specific code examples
Ajax (Asynchronous JavaScript and XML) is a technology used for front-end and back-end interaction. Data interaction with the server can be carried out through asynchronous requests without refreshing the entire page. In actual application development, we often need to use Ajax to submit form data or other types of data. The following will introduce five common Ajax data submission methods and provide specific code examples.
- Submit data by GET method:
Code example:
$.ajax({ url: "example.com/data", type: "GET", data: {name: "John", age: 25}, success: function(response) { console.log(response); } });
- Submit data by POST method:
Code example:
$.ajax({ url: "example.com/data", type: "POST", data: {name: "John", age: 25}, success: function(response) { console.log(response); } });
- Submit data in JSON mode:
Code example:
$.ajax({ url: "example.com/data", type: "POST", data: JSON.stringify({name: "John", age: 25}), contentType: "application/json", success: function(response) { console.log(response); } });
- Multimedia file upload:
Code example:
var formData = new FormData(); formData.append("file", fileInput.files[0]); $.ajax({ url: "example.com/upload", type: "POST", data: formData, contentType: false, processData: false, success: function(response) { console.log(response); } });
- Submit data using XML:
Code example:
var xmlData = '<user><name>John</name><age>25</age></user>'; $.ajax({ url: "example.com/data", type: "POST", data: xmlData, contentType: "application/xml", success: function(response) { console.log(response); } });
The above are five common Ajax data submissions code example. In actual development, the appropriate method can be selected to submit data according to specific needs. At the same time, we also need to pay attention to the issue of cross-domain requests to ensure the security and stability of front-end and back-end interactions.
Summary:
By mastering the five data submission methods of Ajax, we can handle front-end and back-end data interaction more flexibly. Not only can it improve user experience, but it can also reduce page refresh and resource consumption. Flexible application of these techniques in project development can improve development efficiency and code quality.
The above is the detailed content of Learn to use five different data submission methods to implement Ajax. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


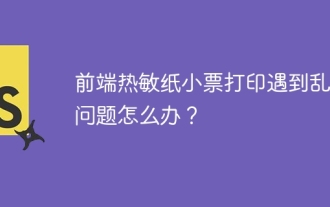
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
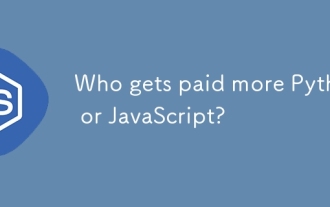
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
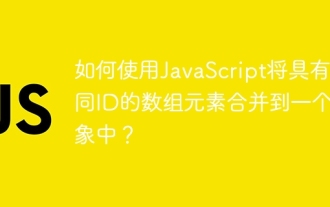
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
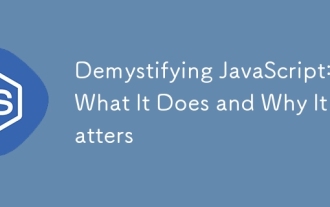
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
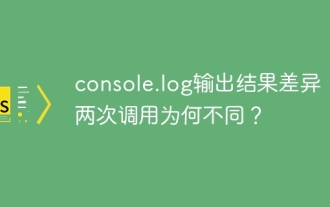
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
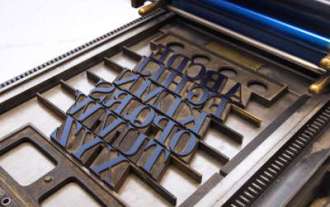
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
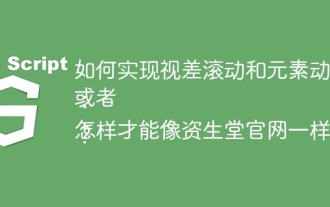
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
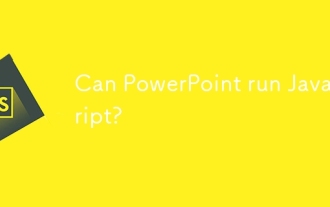
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
