


Essential for Golang programmers: summary of common libraries and practical applications
Jan 18, 2024 am 08:53 AMMust-read for Golang programmers: Summary of commonly used libraries and practical applications
Introduction:
Go language (Golang) is a powerful statically typed programming language , its concise syntax and efficient performance make it widely used in various fields. As a Golang programmer, it is very important to understand and master some commonly used libraries to improve development efficiency and code quality. This article will introduce some commonly used Golang libraries and give specific practical application examples.
1. Network development library
- net/http
net/http is one of the standard libraries of the Go language. It provides support for the HTTP protocol, allowing developers to Create and handle HTTP requests and responses very conveniently. The following is an example of using the net/http library to create a simple web service:
package main import ( "fmt" "net/http" ) func mainHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, World!") } func main() { http.HandleFunc("/", mainHandler) http.ListenAndServe(":8080", nil) }
- gin
gin is a lightweight web framework developed based on the net/http library and has High performance and flexible features. The following is an example of using the gin library to create a simple web service:
package main import "github.com/gin-gonic/gin" func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.String(http.StatusOK, "Hello, World!") }) r.Run(":8080") }
2. Database operation library
- database/sql
database/sql is in Go language One of the standard libraries, which provides an operation interface for the database. Use database/sql to connect to various types of databases, such as MySQL, PostgreSQL, SQLite, etc. The following is an example of using the database/sql library to connect to a MySQL database and perform query operations:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(host:port)/database") if err != nil { fmt.Println("Failed to connect to database:", err) return } defer db.Close() rows, err := db.Query("SELECT * FROM users") if err != nil { fmt.Println("Failed to query from database:", err) return } defer rows.Close() for rows.Next() { var id int var name string err := rows.Scan(&id, &name) if err != nil { fmt.Println("Failed to scan rows:", err) return } fmt.Println("ID:", id, "Name:", name) } }
- gorm
gorm is a powerful ORM library that provides simple and convenient database operations. methods, and supports multiple database types. The following is an example of using the gorm library to connect to a MySQL database and perform query operations:
package main import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" ) type User struct { ID uint Name string } func main() { dsn := "user:password@tcp(host:port)/database" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { fmt.Println("Failed to connect to database:", err) return } defer db.Close() var users []User result := db.Find(&users) if result.Error != nil { fmt.Println("Failed to query from database:", result.Error) return } for _, user := range users { fmt.Println("ID:", user.ID, "Name:", user.Name) } }
3. Log processing library
- log
log is the standard of the Go language One of the packages that provides simple logging functionality. The following is an example of using the log library to record logs:
package main import ( "log" "os" ) func main() { file, err := os.Create("app.log") if err != nil { log.Fatal("Failed to create log file:", err) } defer file.Close() log.SetOutput(file) log.Println("This is a log message") }
- zap
zap is a high-performance log library with rich features, such as structured logs and highly customizable wait. The following is an example of using the zap library to record logs:
package main import "go.uber.org/zap" func main() { logger, _ := zap.NewProduction() defer logger.Sync() logger.Info("This is a log message") }
Summary:
This article introduces common Golang libraries in network development, database operations and log processing, and gives specific instructions. Practical application examples. Mastering these common libraries will help improve development efficiency and code quality. I hope it will be helpful to Golang programmers!
The above is the detailed content of Essential for Golang programmers: summary of common libraries and practical applications. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
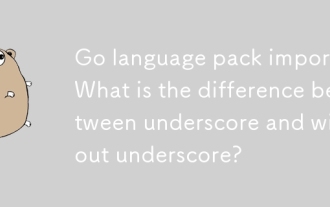
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
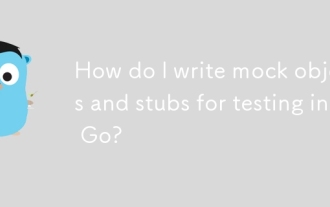
How do I write mock objects and stubs for testing in Go?
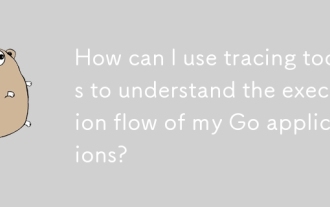
How can I use tracing tools to understand the execution flow of my Go applications?
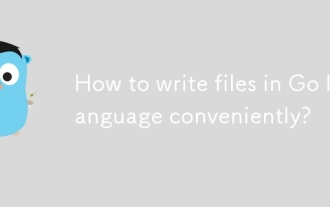
How to write files in Go language conveniently?
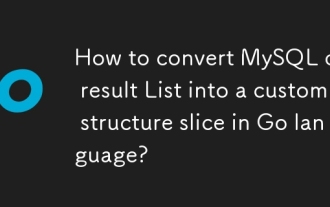
How to convert MySQL query result List into a custom structure slice in Go language?
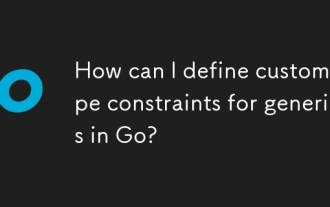
How can I define custom type constraints for generics in Go?
