


Django: a full-stack framework or backend development only?
Django is a popular Python web framework that provides many powerful features to make web application development easier and more efficient. However, some people think that Django is only suitable for back-end development and not for full-stack development. This article will dive into whether Django is limited to backend development and provide some concrete code examples.
As to whether Django is suitable for full-stack development, the answer is yes, it depends on the specific scope of full-stack development you understand. If you think full-stack development only involves front-end and back-end development, then Django has you covered. If you consider that full-stack development also includes working with servers, databases, APIs, and other technologies, then Django can do the job too.
Specifically, Django provides some powerful tools and libraries that make it ideal for developing websites and web applications. Here are some examples:
- Front-End Development
Django uses a template engine to render HTML. Template engines allow you to easily mix dynamic content with static HTML interfaces. Django also provides some basic CSS and JavaScript libraries to make your website more beautiful and dynamic.
Here is a simple example showing how to use the template engine to render HTML in Django:
# views.py from django.shortcuts import render def home(request): username = 'Alice' return render(request, 'home.html', {'username': username})
<!-- home.html --> <!DOCTYPE html> <html> <head> <title>Home Page</title> </head> <body> <h1 id="Welcome-username">Welcome, {{ username }}!</h1> </body> </html>
In this example, we define a home view that will render the template home. html. We also pass a variable username to the template, and the template uses {{ username }} to render the value of this variable.
- Backend development
Django is a complete backend framework that provides many excellent tools and libraries to handle databases, security, form validation, etc. End development issues. Here is a simple example showing how to define a model in Django and save it to the database:
# models.py from django.db import models class Person(models.Model): first_name = models.CharField(max_length=30) last_name = models.CharField(max_length=30) def __str__(self): return f'{self.first_name} {self.last_name}'
# views.py from django.shortcuts import render from .models import Person def home(request): person = Person(first_name='Alice', last_name='Smith') person.save() return render(request, 'home.html', {'person': person})
<!-- home.html --> <!DOCTYPE html> <html> <head> <title>Home Page</title> </head> <body> <h1 id="Hello-person">Hello, {{ person }}!</h1> </body> </html>
In this example, we define a model called Person and use it to create A character named Alice Smith. We pass the person object into the view that renders the home.html template and use {{ person }} in the template to render the string representation of this object.
- Server and API
Django not only provides the basic functionality required for web applications, but also provides the functionality to handle HTTP requests and responses. In Django, you can easily create REST API-based services and manage them using Django's admin interface.
Here is a simple REST API example:
# serializers.py from rest_framework import serializers from .models import Person class PersonSerializer(serializers.ModelSerializer): class Meta: model = Person fields = ['first_name', 'last_name']
# views.py from rest_framework import generics from .models import Person from .serializers import PersonSerializer class PersonList(generics.ListCreateAPIView): queryset = Person.objects.all() serializer_class = PersonSerializer
In this example, we use Django Rest Framework (DRF) to create a simple REST API. We define a serializer called PersonSerializer that converts the Person model into JSON format. We also define a view called PersonList that provides GET and POST requests and returns a JSON representation of the Person model.
- Database
Django comes with a built-in ORM, which makes it ideal for working with databases. Django ORM allows you to operate your database using Python code instead of the SQL query language. Here is a simple example that shows how to define a model in Django and query the data in the database:
# models.py from django.db import models class Person(models.Model): first_name = models.CharField(max_length=30) last_name = models.CharField(max_length=30) def __str__(self): return f'{self.first_name} {self.last_name}'
# views.py from django.shortcuts import render from .models import Person def home(request): people = Person.objects.all() return render(request, 'home.html', {'people': people})
<!-- home.html --> <!DOCTYPE html> <html> <head> <title>Home Page</title> </head> <body> <h1 id="People">People:</h1> <ul> {% for person in people %} <li>{{ person }}</li> {% endfor %} </ul> </body> </html>
In this example, we define a model called Person and use it to query the database All characters in . We list the person objects into the home.html template and use the template tags {% for person in people %} and {% endfor %} to loop through all the people.
To sum up, Django is a very powerful and comprehensive framework that can be applied to full-stack development. Whether you want to develop front-end, back-end, API, server or database, Django has powerful tools and libraries to meet your needs.
The above is the detailed content of Django: a full-stack framework or backend development only?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


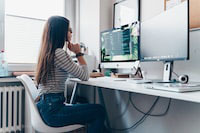
This article will explain in detail how PHP formats rows into CSV and writes file pointers. I think it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Format rows to CSV and write to file pointer Step 1: Open file pointer $file=fopen("path/to/file.csv","w"); Step 2: Convert rows to CSV string using fputcsv( ) function converts rows to CSV strings. The function accepts the following parameters: $file: file pointer $fields: CSV fields as an array $delimiter: field delimiter (optional) $enclosure: field quotes (
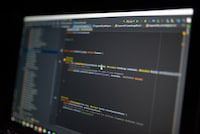
This article will explain in detail about changing the current umask in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Overview of PHP changing current umask umask is a php function used to set the default file permissions for newly created files and directories. It accepts one argument, which is an octal number representing the permission to block. For example, to prevent write permission on newly created files, you would use 002. Methods of changing umask There are two ways to change the current umask in PHP: Using the umask() function: The umask() function directly changes the current umask. Its syntax is: intumas
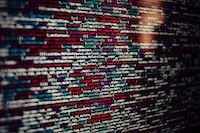
This article will explain in detail how to create a file with a unique file name in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Creating files with unique file names in PHP Introduction Creating files with unique file names in PHP is essential for organizing and managing your file system. Unique file names ensure that existing files are not overwritten and make it easier to find and retrieve specific files. This guide will cover several ways to generate unique filenames in PHP. Method 1: Use the uniqid() function The uniqid() function generates a unique string based on the current time and microseconds. This string can be used as the basis for the file name.
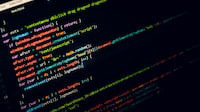
This article will explain in detail about PHP calculating the MD5 hash of files. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP calculates the MD5 hash of a file MD5 (MessageDigest5) is a one-way encryption algorithm that converts messages of arbitrary length into a fixed-length 128-bit hash value. It is widely used to ensure file integrity, verify data authenticity and create digital signatures. Calculating the MD5 hash of a file in PHP PHP provides multiple methods to calculate the MD5 hash of a file: Use the md5_file() function. The md5_file() function directly calculates the MD5 hash value of the file and returns a 32-character
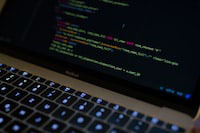
This article will explain in detail how PHP returns an array after key value flipping. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Key Value Flip Array Key value flip is an operation on an array that swaps the keys and values in the array to generate a new array with the original key as the value and the original value as the key. Implementation method In PHP, you can perform key-value flipping of an array through the following methods: array_flip() function: The array_flip() function is specially used for key-value flipping operations. It receives an array as argument and returns a new array with the keys and values swapped. $original_array=[
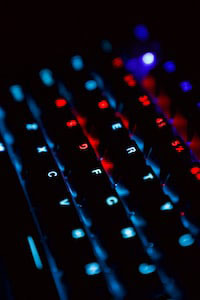
This article will explain in detail how PHP truncates files to a given length. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Introduction to PHP file truncation The file_put_contents() function in PHP can be used to truncate files to a specified length. Truncation means removing part of the end of a file, thereby shortening the file length. Syntax file_put_contents($filename,$data,SEEK_SET,$offset);$filename: the file path to be truncated. $data: Empty string to be written to the file. SEEK_SET: designated as the beginning of the file
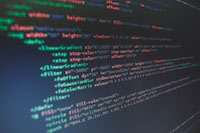
This article will explain in detail how PHP determines whether a specified key exists in an array. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP determines whether a specified key exists in an array: In PHP, there are many ways to determine whether a specified key exists in an array: 1. Use the isset() function: isset($array["key"]) This function returns a Boolean value, true if the specified key exists, false otherwise. 2. Use array_key_exists() function: array_key_exists("key",$arr

This article will explain in detail the numerical encoding of the error message returned by PHP in the previous Mysql operation. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. . Using PHP to return MySQL error information Numeric Encoding Introduction When processing mysql queries, you may encounter errors. In order to handle these errors effectively, it is crucial to understand the numerical encoding of error messages. This article will guide you to use php to obtain the numerical encoding of Mysql error messages. Method of obtaining the numerical encoding of error information 1. mysqli_errno() The mysqli_errno() function returns the most recent error number of the current MySQL connection. The syntax is as follows: $erro
