


A closer look at Django: a web application framework in Python
Django is one of the most popular web application frameworks in the Python language. Its purpose is to help developers quickly build high-quality, easy-to-maintain web applications. If you're looking for a quick way to start web development, Django is a great choice.
In this article, we’ll explore some of Django’s core concepts and features, and provide some concrete code examples to help you better understand how it works.
Core concepts of the Django framework:
1. Views
In Django, views are responsible for processing user requests and returning corresponding responses. View functions usually take an HTTP request as a parameter and return an HTTP response object. They can handle the request directly and return a response, or they can hand the request to a template for rendering.
The following is a simple view function:
from django.http import HttpResponse def hello(request): return HttpResponse("Hello, World!")
This function accepts a parameter named request, which stores all the information of the HTTP request. It returns an HTTP response containing the string "Hello, World!".
To associate this view function with a URL pattern, you need to create a URL pattern in your Django application's urls.py file. Here is the sample code:
from django.urls import path from . import views urlpatterns = [ path('hello/', views.hello, name='hello'), ]
This URL pattern will match all URLs ending with /hello/ and send them to the hello view function.
2. Models
In Django, models are used to define the data structure of the application. They are responsible for storing application data in the database and provide APIs for reading and modifying the data. Django uses a relational database management system by default, such as MySQL or SQLite, but other database modules can also be used.
Here is a simple model definition:
from django.db import models class Book(models.Model): title = models.CharField(max_length=100) author = models.CharField(max_length=100) published_date = models.DateField() def __str__(self): return self.title
This model defines a model called Book and stores the title, author and publication date for each book. It also defines a __str__ method that returns the character representation of the model.
To use this model, you need to create a database table in your Django application. This can be done by running the following commands in the terminal:
$ python manage.py makemigrations $ python manage.py migrate
These commands will create the database tables required by the Book model.
3. Templates
In Django, templates are used to generate dynamic HTML pages. They are defined as HTML files, which contain some special tags and variables. Django replaces these tags and variables with actual data and returns the results to the user's browser.
Here is a simple template:
{% extends "base.html" %} {% block content %} <h1 id="book-title">{{ book.title }}</h1> <p>Author: {{ book.author }}</p> <p>Published on: {{ book.published_date }}</p> {% endblock %}
This template uses Django’s template language to display the title, author, and publication date of a book. It also inherits a base template called base.html and defines the actual content in a block called content.
To use templates, you need to pass data to them in the view function. This can be done in the following way:
from django.shortcuts import render from .models import Book def book_detail(request, book_id): book = Book.objects.get(id=book_id) return render(request, 'book_detail.html', {'book': book})
This view function reads the book with the specified id from the database and passes this object to the book_detail.html template.
To summarize, Django is a powerful and easy-to-use web application framework. It provides many useful features such as models, views, and templates to help build high-quality web applications. In this article, we introduce some of Django's core concepts and features, and provide some concrete code examples to help you better understand how it works.
The above is the detailed content of A closer look at Django: a web application framework in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


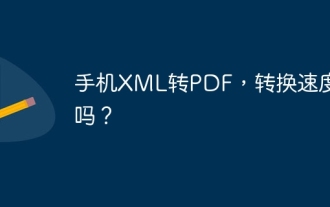
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
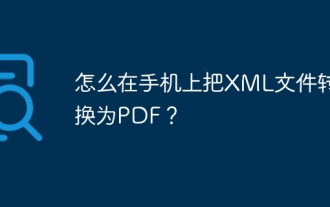
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
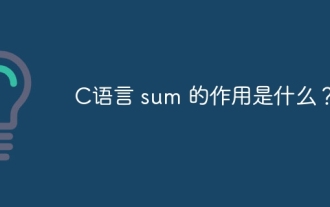
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
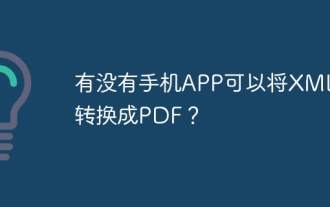
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
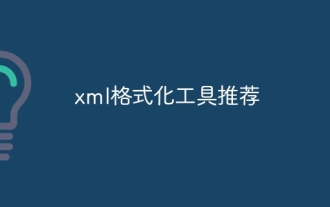
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
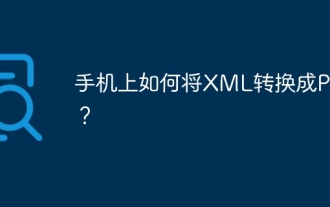
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
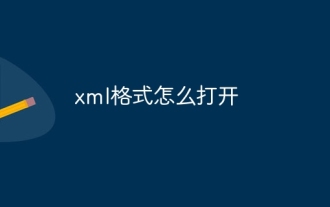
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
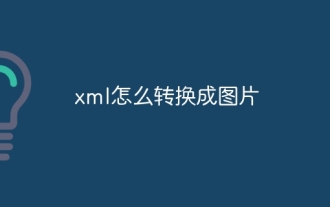
XML can be converted to images by using an XSLT converter or image library. XSLT Converter: Use an XSLT processor and stylesheet to convert XML to images. Image Library: Use libraries such as PIL or ImageMagick to create images from XML data, such as drawing shapes and text.
