


Use Python to write game programs and create your own game works
Use Python to write game programs and create your own game works, you need specific code examples
Python is a high-level, general-purpose, interpreted programming language. It has concise and clear syntax, is easy to learn, and has a wide range of applications. It can be used in various fields such as network applications, graphical interface programming, and game development.
This article will introduce how to use Python language to write a simple game program and provide specific code examples. Readers can follow the sample code to learn basic game development skills, and then create their own game works according to their own needs.
- Pygame library introduction
When using Python to write game programs, we can use the Pygame library to implement game development. Pygame is a Python module specifically used for game development. It provides many simple and easy-to-use game functions and tools, which can greatly simplify the game development process.
In order to use the Pygame library, we need to install it first. You can download the corresponding installation package from the Python official website and install it according to the installation tutorial. After the installation is complete, we can introduce the Pygame library in the Python code in the following ways:
import pygame
- Writing the first game program
Next, we will introduce How to use the Pygame library to write the first game program - "Snake". This is a relatively simple game, suitable for beginners to practice.
2.1 First, we need to import the Pygame library and initialize Pygame:
import pygame pygame.init()
2.2 Then, we need to create a window to display the game screen:
window = pygame.display.set_mode((500, 500)) pygame.display.set_caption("Snake Game")
The above code creates Create a window with a size of 500*500 and set the title of the window to "Snake Game".
2.3 Next, define the snake class:
class Snake: def __init__(self, x, y): self.x = x self.y = y self.width = 10 self.height = 10 self.dx = 0 self.dy = 0 self.body = []
The above code defines a greedy snake class that includes attributes such as position, size, direction, and body.
2.4 Next, we need to define a function to draw the greedy snake:
def draw_snake(snake): for block in snake.body: pygame.draw.rect(window, (0, 255, 0), [block[0], block[1], snake.width, snake.height])
The above code loops through the body of the greedy snake and uses the drawing function pygame.draw.rect in Pygame to draw it Each body block.
2.5 Next we need to define a main loop to process game events and update the game screen:
snake = Snake(250, 250) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: snake.dx = -10 snake.dy = 0 elif keys[pygame.K_RIGHT]: snake.dx = 10 snake.dy = 0 elif keys[pygame.K_UP]: snake.dx = 0 snake.dy = -10 elif keys[pygame.K_DOWN]: snake.dx = 0 snake.dy = 10 snake.x += snake.dx snake.y += snake.dy window.fill((0, 0, 0)) draw_snake(snake) pygame.display.update()
The above code creates an instance of Snake and uses a while loop to continuously update the game screen. In the loop, we first handle the QUIT event to exit the game, and then monitor the keyboard input through pygame.key.get_pressed in Pygame to change the moving direction of the snake. Next, we update the snake's coordinates, clear the window, and call the draw_snake function to draw the snake's body. Finally, update the game screen through the pygame.display.update function.
- Summary
The above are the basic steps and code examples for writing a simple game using Python and the Pygame library. By studying the content of this article, readers can understand how to use Python to implement game development processes and techniques, laying the foundation for creating their own game works. Of course, in practice, it is necessary to continuously accumulate experience and innovation in order to write richer, more complex and interesting game works.
The above is the detailed content of Use Python to write game programs and create your own game works. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


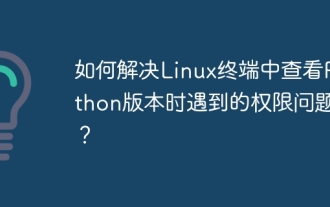
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
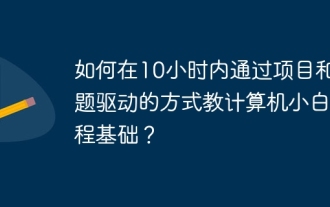
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
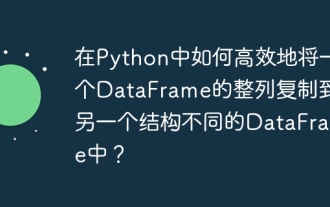
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
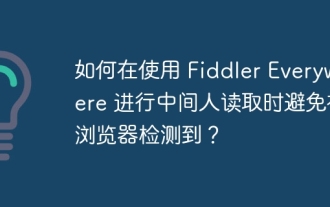
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
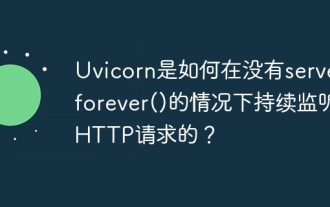
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
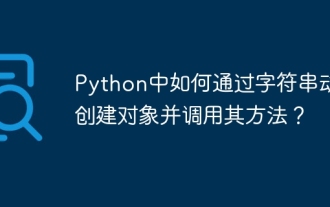
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
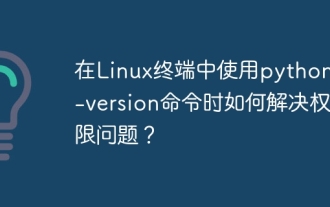
Using python in Linux terminal...
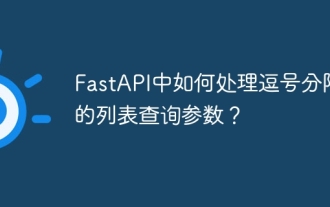
Fastapi ...
