


Using jwt-go library to implement JWT Token verification and authentication in Go language
Use jwt-go library to implement JWT Token authentication in Go language
JWT (JSON Web Token) is a lightweight authentication and authorization method This method can help us transfer safe and trustworthy information between users and systems based on JSON format. In the process of constructing JWT Token, we need to sign the Payload, which also means that when we parse the Token on the server side, we can verify its legitimacy.
We can use the jwt-go library in the Go language to implement the authentication function of JWT Token. The jwt-go library provides a simple way to generate, verify and parse JWT Token. JWT Token consists of two parts: header and payload, separated by dots.
The header contains two attributes alg (algorithm) and typ (type). The algorithm can use HMAC, RSA or some other encryption algorithms, and the type refers to the type of JWT Token, which is specified in the standard The value in is a JWT.
The payload also has some attributes, but these attributes are not standard attributes, but custom attributes, used for communication between the server and the client.
Now, let us take a look at the specific implementation process.
Install dependent libraries
Before starting to use the jwt-go library, we need to install it first. You can use the following command to install:
go get github.com/dgrijalva/jwt-go
Import dependent libraries
Import jwt-go library in the code:
import ( "github.com/dgrijalva/jwt-go" )
Generate JWT Token
To generate JWT Token in Go language, we need to sign the Payload. The signing process requires the use of a pair Public key and private key, the public key is used to verify the legitimacy of the Token, and the private key is used to generate the Token. We can save the private key in the configuration file or environment variable to ensure its security.
The following is an example of generating a JWT Token:
// 生成JWT Token func GenerateJwtToken() (string, error) { // 加载私钥 privateKeyByte, err := ioutil.ReadFile("jwtRS256.key") if err != nil { return "", err } privateKey, err := jwt.ParseRSAPrivateKeyFromPEM(privateKeyByte) if err != nil { return "", err } // 设置Payload claims := jwt.MapClaims{ "username": "admin", "exp": time.Now().Add(time.Hour * 24).Unix(), // 过期时间 } // 生成JWT Token token := jwt.NewWithClaims(jwt.SigningMethodRS256, claims) tokenString, err := token.SignedString(privateKey) if err != nil { return "", err } // 返回生成的Token return tokenString, nil }
In the above example, we loaded a private key, then set the Payload, and used the private key signature to generate a JWT Token , and finally return this JWT Token.
Verify JWT Token
To verify the legitimacy of JWT Token in Go language, we need to first parse the Payload from the Token, and then use the public key to verify the Token.
The following is an example of verifying a JWT Token:
// 验证JWT Token func ParseJwtToken(tokenString string) (jwt.MapClaims, error) { // 加载公钥 publicKeyByte, err := ioutil.ReadFile("jwtRS256.pem") if err != nil { return nil, err } publicKey, err := jwt.ParseRSAPublicKeyFromPEM(publicKeyByte) if err != nil { return nil, err } // 解析JWT Token token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) { _, ok := token.Method.(*jwt.SigningMethodRSA) if !ok { return nil, fmt.Errorf("unexpected signing method: %v", token.Header["alg"]) } return publicKey, nil }) if err != nil { return nil, err } if claims, ok := token.Claims.(jwt.MapClaims); ok && token.Valid { return claims, nil } return nil, fmt.Errorf("invalid token") }
In the above example, we loaded a public key, then parsed a JWT Token, and verified the JWT using the public key The legality of the Token, and finally returns the Payload.
Summary
Using the jwt-go library to implement JWT Token authentication in the Go language is a simple and effective way. As a lightweight authentication and authorization method, JWT Token can securely transfer information between the server and the client. By using the jwt-go library, you can quickly generate, verify and parse JWT Token, and ensure security during the transmission process.
The above is the detailed content of Using jwt-go library to implement JWT Token verification and authentication in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


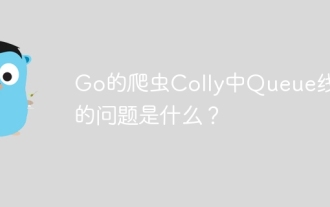
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
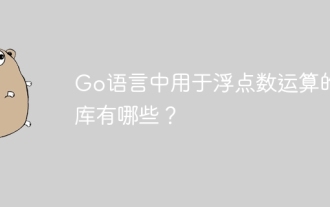
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
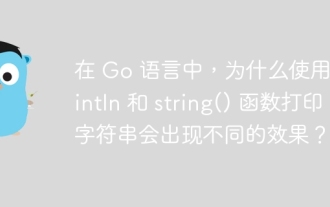
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
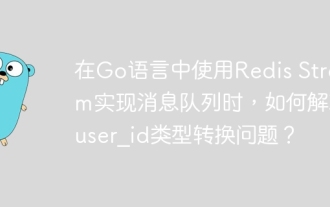
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
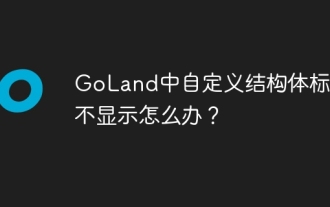
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
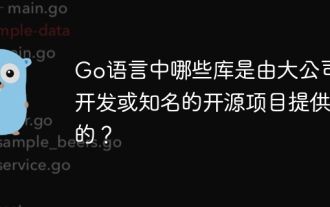
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
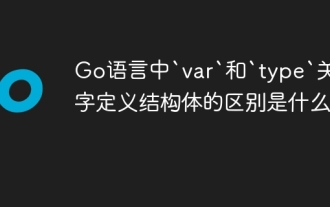
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
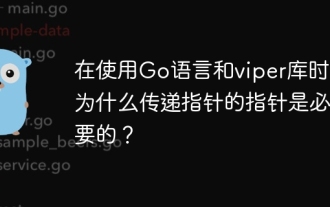
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
