


Go language database connection guide: guide you step by step to perform database operations
Go language is a relatively beginner-friendly programming language that is concise, efficient and easy to learn. As a developer, connecting and operating with databases is an essential part of our daily work. In this article, I will show you how to connect to a database using Go language and provide some specific code examples.
First, we need to import the database driver of Go language. Currently, Go language supports a variety of databases, such as MySQL, SQLite, PostgreSQL, etc. You can choose the appropriate driver based on your needs. In this article, we take MySQL as an example.
First, we need to import the MySQL driver package into the Go language project. You can add the following code in the go.mod file:
require github.com/go-sql-driver/mysql v1.7.0
Then, we can establish a connection with the MySQL database through the following code:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main() { // 数据库连接信息 username := "root" password := "123456" host := "127.0.0.1" port := "3306" database := "example" // 建立数据库连接 connectionString := fmt.Sprintf("%s:%s@tcp(%s:%s)/%s?charset=utf8&parseTime=True&loc=Local", username, password, host, port, database) db, err := sql.Open("mysql", connectionString) if err != nil { log.Fatal(err) } defer db.Close() }
In the above code, we used database/sql
package and github.com/go-sql-driver/mysql
package. sql.Open()
The function is used to establish a connection with the MySQL database. The first parameter is the driver name and the second parameter is the connection string. If an error occurs when connecting to the database, we will use the log.Fatal()
function to print the error message and exit the program.
Once the connection to the database is successfully established, we can perform various database operations. The following are some common database operation examples:
- Query data:
rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var id int var name string err := rows.Scan(&id, &name) if err != nil { log.Fatal(err) } fmt.Println(id, name) }
- Insert data:
stmt, err := db.Prepare("INSERT INTO users (name) VALUES (?)") if err != nil { log.Fatal(err) } defer stmt.Close() res, err := stmt.Exec("John") if err != nil { log.Fatal(err) } lastID, err := res.LastInsertId() if err != nil { log.Fatal(err) } fmt.Println("Inserted ID:", lastID)
- Update data:
stmt, err := db.Prepare("UPDATE users SET name = ? WHERE id = ?") if err != nil { log.Fatal(err) } defer stmt.Close() res, err := stmt.Exec("John Doe", 1) if err != nil { log.Fatal(err) } affectedRows, err := res.RowsAffected() if err != nil { log.Fatal(err) } fmt.Println("Affected Rows:", affectedRows)
- Delete data:
stmt, err := db.Prepare("DELETE FROM users WHERE id = ?") if err != nil { log.Fatal(err) } defer stmt.Close() res, err := stmt.Exec(1) if err != nil { log.Fatal(err) } affectedRows, err := res.RowsAffected() if err != nil { log.Fatal(err) } fmt.Println("Affected Rows:", affectedRows)
The above codes are just some basic database operation examples. In actual development, you may need more complex queries and transaction processing. However, through the above examples, you have already understood how to use Go language to connect to the database and perform some common database operations.
To summarize, this article introduces in detail how to use Go language to connect to the MySQL database and gives some specific code examples. I hope this will help you connect and operate with the database in Go language development. If you have any questions or confusion, you can leave a message below and I will try my best to answer it. I wish you a happy learning and write elegant and efficient Go language code!
The above is the detailed content of Go language database connection guide: guide you step by step to perform database operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
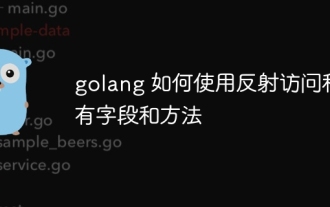
You can use reflection to access private fields and methods in Go language: To access private fields: obtain the reflection value of the value through reflect.ValueOf(), then use FieldByName() to obtain the reflection value of the field, and call the String() method to print the value of the field . Call a private method: also obtain the reflection value of the value through reflect.ValueOf(), then use MethodByName() to obtain the reflection value of the method, and finally call the Call() method to execute the method. Practical case: Modify private field values and call private methods through reflection to achieve object control and unit test coverage.
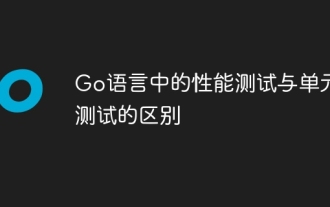
Performance tests evaluate an application's performance under different loads, while unit tests verify the correctness of a single unit of code. Performance testing focuses on measuring response time and throughput, while unit testing focuses on function output and code coverage. Performance tests simulate real-world environments with high load and concurrency, while unit tests run under low load and serial conditions. The goal of performance testing is to identify performance bottlenecks and optimize the application, while the goal of unit testing is to ensure code correctness and robustness.
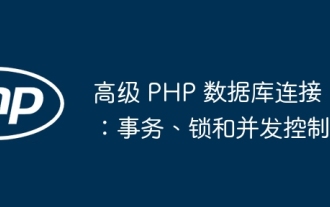
Advanced PHP database connections involve transactions, locks, and concurrency control to ensure data integrity and avoid errors. A transaction is an atomic unit of a set of operations, managed through the beginTransaction(), commit(), and rollback() methods. Locks prevent simultaneous access to data via PDO::LOCK_SHARED and PDO::LOCK_EXCLUSIVE. Concurrency control coordinates access to multiple transactions through MySQL isolation levels (read uncommitted, read committed, repeatable read, serialized). In practical applications, transactions, locks and concurrency control are used for product inventory management on shopping websites to ensure data integrity and avoid inventory problems.
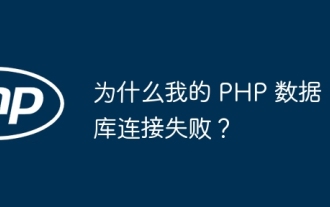
Reasons for a PHP database connection failure include: the database server is not running, incorrect hostname or port, incorrect database credentials, or lack of appropriate permissions. Solutions include: starting the server, checking the hostname and port, verifying credentials, modifying permissions, and adjusting firewall settings.
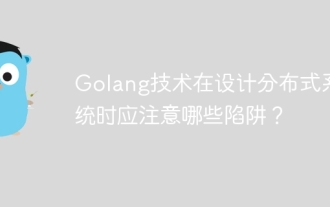
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
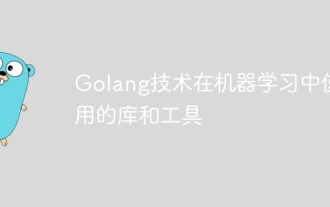
Libraries and tools for machine learning in the Go language include: TensorFlow: a popular machine learning library that provides tools for building, training, and deploying models. GoLearn: A series of classification, regression and clustering algorithms. Gonum: A scientific computing library that provides matrix operations and linear algebra functions.
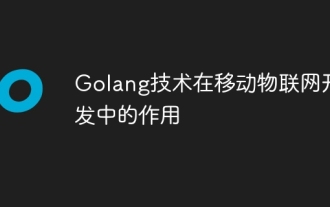
With its high concurrency, efficiency and cross-platform nature, Go language has become an ideal choice for mobile Internet of Things (IoT) application development. Go's concurrency model achieves a high degree of concurrency through goroutines (lightweight coroutines), which is suitable for handling a large number of IoT devices connected at the same time. Go's low resource consumption helps run applications efficiently on mobile devices with limited computing and storage. Additionally, Go’s cross-platform support enables IoT applications to be easily deployed on a variety of mobile devices. The practical case demonstrates using Go to build a BLE temperature sensor application, communicating with the sensor through BLE and processing incoming data to read and display temperature readings.
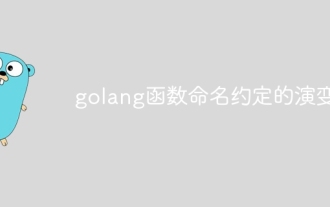
The evolution of Golang function naming convention is as follows: Early stage (Go1.0): There is no formal convention and camel naming is used. Underscore convention (Go1.5): Exported functions start with a capital letter and are prefixed with an underscore. Factory function convention (Go1.13): Functions that create new objects are represented by the "New" prefix.
