


Understanding PHP caching mechanisms: exploring different implementations
Exploring the PHP caching mechanism: To understand different implementation methods, specific code examples are required
The caching mechanism is a very important part in web development and can greatly improve the website performance and responsiveness. As a popular server-side language, PHP also provides a variety of caching mechanisms to optimize performance. This article will explore PHP's caching mechanism, introduce different implementation methods, and provide specific code examples.
- File Cache
File cache is one of the simplest and most common PHP caching methods. Its principle is simple: store the calculation results in a file and read the file contents when needed instead of recalculating. The following is a sample code:
function getDataFromCache($cacheKey, $cacheTime) { $cacheFile = 'cache/' . $cacheKey . '.txt'; // 检查缓存文件是否存在并且未过期 if (file_exists($cacheFile) && (filemtime($cacheFile) + $cacheTime) > time()) { // 从缓存文件读取数据 $data = file_get_contents($cacheFile); return unserialize($data); } else { // 重新计算数据 $data = calculateData(); // 将数据写入缓存文件 file_put_contents($cacheFile, serialize($data)); return $data; } }
- Memcached cache
Memcached is a high-performance distributed memory object caching system and one of the commonly used caching methods in PHP. It stores data in memory and is faster and more efficient than file caching. The following is a sample code:
// 创建Memcached对象 $memcached = new Memcached(); $memcached->addServer('localhost', 11211); function getDataFromCache($cacheKey, $cacheTime) { global $memcached; // 尝试从Memcached中获取数据 $data = $memcached->get($cacheKey); if ($data !== false) { return $data; } else { // 重新计算数据 $data = calculateData(); // 将数据存入Memcached $memcached->set($cacheKey, $data, $cacheTime); return $data; } }
- APC Cache
APC (Alternative PHP Cache) is a built-in caching extension of PHP that can store data in shared memory, which is better than file caching And Memcached is faster. The following is a sample code:
// 开启APC缓存 apc_store('enable_cache', true); function getDataFromCache($cacheKey, $cacheTime) { // 检查APC缓存是否开启 if (apc_fetch('enable_cache')) { // 尝试从APC中获取数据 $data = apc_fetch($cacheKey); if ($data !== false) { return $data; } } // 重新计算数据 $data = calculateData(); // 将数据存入APC apc_store($cacheKey, $data, $cacheTime); return $data; }
- Redis Cache
Redis is an in-memory database that supports persistence and is also one of the commonly used caching methods in PHP. It has high performance and reliability and supports a variety of data structures. The following is a sample code:
// 创建Redis对象 $redis = new Redis(); $redis->connect('127.0.0.1', 6379); function getDataFromCache($cacheKey, $cacheTime) { global $redis; // 尝试从Redis中获取数据 $data = $redis->get($cacheKey); if ($data !== false) { return unserialize($data); } else { // 重新计算数据 $data = calculateData(); // 将数据存入Redis $redis->set($cacheKey, serialize($data)); $redis->expire($cacheKey, $cacheTime); return $data; } }
The above are sample codes for several common PHP caching methods. Choosing the appropriate caching method according to the actual situation, and performing corresponding configuration and optimization as needed can effectively improve website performance and response speed. In practical applications, in addition to caching data, database query results, page fragments, etc. can also be cached to further optimize performance.
The above is the detailed content of Understanding PHP caching mechanisms: exploring different implementations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


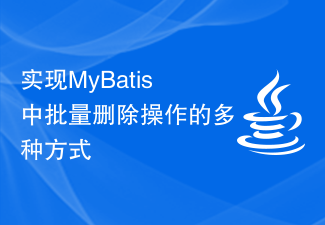
Several ways to implement batch deletion statements in MyBatis require specific code examples. In recent years, due to the increasing amount of data, batch operations have become an important part of database operations. In actual development, we often need to delete records in the database in batches. This article will focus on several ways to implement batch delete statements in MyBatis and provide corresponding code examples. Use the foreach tag to implement batch deletion. MyBatis provides the foreach tag, which can easily traverse a set.

Analysis of MyBatis' caching mechanism: The difference and application of first-level cache and second-level cache In the MyBatis framework, caching is a very important feature that can effectively improve the performance of database operations. Among them, first-level cache and second-level cache are two commonly used caching mechanisms in MyBatis. This article will analyze the differences and applications of first-level cache and second-level cache in detail, and provide specific code examples to illustrate. 1. Level 1 Cache Level 1 cache is also called local cache. It is enabled by default and cannot be turned off. The first level cache is SqlSes
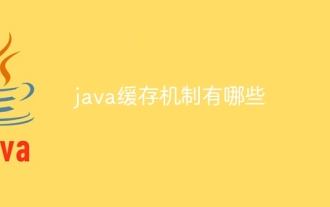
Java cache mechanisms include memory cache, data structure cache, cache framework, distributed cache, cache strategy, cache synchronization, cache invalidation mechanism, compression and encoding, etc. Detailed introduction: 1. Memory cache, Java's memory management mechanism will automatically cache frequently used objects to reduce the cost of memory allocation and garbage collection; 2. Data structure cache, Java's built-in data structures, such as HashMap, LinkedList, HashSet, etc. , with efficient caching mechanisms, these data structures use internal hash tables to store elements and more.
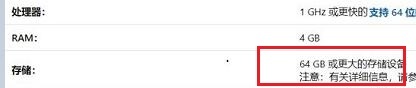
As we all know, if the system disk occupied is too large after the system installation is completed, it may cause system lags, delays, and even file loss. Therefore, before you install the win11 system, you need to know how much C drive space is required to upgrade win11. Let’s take a look with the editor. How much C drive space is required to upgrade win11: Answer: Upgrading win11 requires 20-30GB of C drive space. 1. According to Microsoft’s win11 configuration requirements, you can see that win11 installation requires 64GB of hard drive space. 2. But in fact, generally speaking, there is no need for such a large space. 3. According to feedback from users who have already installed win11, the win11 upgrade requires about 20-30GB of C drive space. 4. But if our door only has
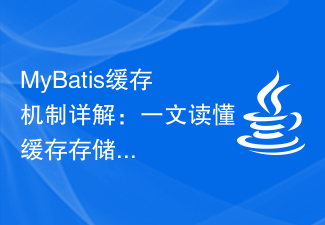
Detailed explanation of MyBatis caching mechanism: One article to understand the principle of cache storage Introduction When using MyBatis for database access, caching is a very important mechanism, which can effectively reduce access to the database and improve system performance. This article will introduce the caching mechanism of MyBatis in detail, including cache classification, storage principles and specific code examples. 1. Cache classification MyBatis cache is mainly divided into two types: first-level cache and second-level cache. The first-level cache is a SqlSession-level cache. When
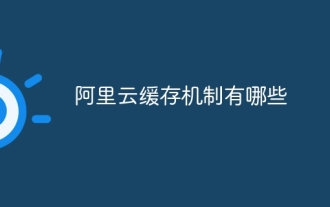
Alibaba Cloud caching mechanisms include Alibaba Cloud Redis, Alibaba Cloud Memcache, distributed cache service DSC, Alibaba Cloud Table Store, CDN, etc. Detailed introduction: 1. Alibaba Cloud Redis: A distributed memory database provided by Alibaba Cloud that supports high-speed reading and writing and data persistence. By storing data in memory, it can provide low-latency data access and high concurrency processing capabilities; 2. Alibaba Cloud Memcache: the cache system provided by Alibaba Cloud, etc.
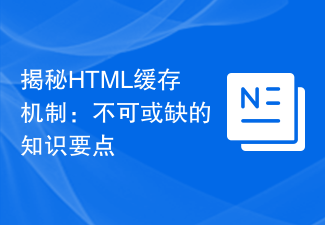
The secret of HTML caching mechanism: essential knowledge points, specific code examples are required In web development, performance has always been an important consideration. The HTML caching mechanism is one of the keys to improving the performance of web pages. This article will reveal the principles and practical skills of the HTML caching mechanism, and provide specific code examples. 1. Principle of HTML caching mechanism During the process of accessing a Web page, the browser requests the server to obtain the HTML page through the HTTP protocol. HTML caching mechanism is to cache HTML pages in the browser
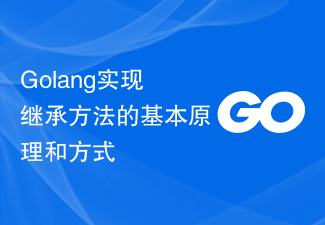
The basic principles and implementation methods of Golang inheritance methods In Golang, inheritance is one of the important features of object-oriented programming. Through inheritance, we can use the properties and methods of the parent class to achieve code reuse and extensibility. This article will introduce the basic principles and implementation methods of Golang inheritance methods, and provide specific code examples. The basic principle of inheritance methods In Golang, inheritance is implemented by embedding structures. When a structure is embedded in another structure, the embedded structure has embedded
