Steps to write a simple neural network using Rust
Rust is a systems-level programming language focused on safety, performance, and concurrency. It aims to provide a safe and reliable programming language suitable for scenarios such as operating systems, network applications, and embedded systems.
Rust's security mainly comes from two aspects: the ownership system and the borrow checker. The ownership system enables the compiler to check code for memory errors at compile time, thus avoiding common memory safety issues. By forcing checking of variable ownership transfers at compile time, Rust ensures that memory resources are properly managed and released. The borrow checker analyzes the life cycle of the variable to ensure that the same variable will not be accessed by multiple threads at the same time, thus avoiding common concurrency security issues. Through the combination of these two mechanisms, Rust can provide a highly secure programming environment and help developers write more reliable software.
Rust's performance mainly comes from two aspects: zero-cost abstraction and no garbage collection. Zero-cost abstraction means that Rust provides abstract features of high-level languages, such as generics and pattern matching, without negatively affecting the execution efficiency of the code. No garbage collection means that Rust can effectively manage memory and avoid the performance loss caused by garbage collection. These features make Rust a high-performance and safe programming language.
Now let’s see how to build a simple neural network using Rust.
First, we need to choose a neural network framework. In Rust, there are many excellent neural network frameworks to choose from, such as TensorFlow, PyTorch, Caffe, etc. But here, we decided to use rustlearn. rustlearn is a lightweight machine learning library focusing on linear algebra and statistical calculations.
Next, we need to define the structure of the neural network. In rustlearn, we can use a structure called NeuralNet to define neural networks. The code is as follows:
let mut net = NeuralNet::new(&[2, 3, 1]);
This example defines a three-layer neural network, with 2 neurons in the input layer, 3 neurons in the hidden layer, and 1 neuron in the output layer.
Then, we need to define the training data for the neural network. In this example, we use a simple logic gate dataset. The code is as follows:
let x = Array::from_vec(vec![vec![0., 0.], vec![0., 1.], vec![1., 0.], vec![1., 1.]]); let y = Array::from_vec(vec![vec![0.], vec![1.], vec![1.], vec![0.]]);
This example defines a training data set containing 4 samples, each sample contains 2 features and 1 label.
Finally, we can use the train method in rustlearn to train the neural network. The code is as follows:
net.train(&x, &y, SGD::default(), Loss::MSE, 1000);
This example uses the stochastic gradient descent algorithm (SGD) and the mean square error loss function (MSE) to train the neural network for 1,000 times.
The complete code is as follows:
use rustlearn::prelude::*; use rustlearn::neural_network::{NeuralNet, SGD, Loss}; fn main() { let mut net = NeuralNet::new(&[2, 3, 1]); let x = Array::from_vec(vec![vec![0., 0.], vec![0., 1.], vec![1., 0.], vec![1., 1.]]); let y = Array::from_vec(vec![vec![0.], vec![1.], vec![1.], vec![0.]]); net.train(&x, &y, SGD::default(), Loss::MSE, 1000); }
The above is the detailed content of Steps to write a simple neural network using Rust. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
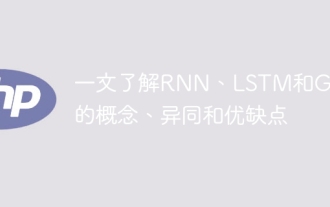
In time series data, there are dependencies between observations, so they are not independent of each other. However, traditional neural networks treat each observation as independent, which limits the model's ability to model time series data. To solve this problem, Recurrent Neural Network (RNN) was introduced, which introduced the concept of memory to capture the dynamic characteristics of time series data by establishing dependencies between data points in the network. Through recurrent connections, RNN can pass previous information into the current observation to better predict future values. This makes RNN a powerful tool for tasks involving time series data. But how does RNN achieve this kind of memory? RNN realizes memory through the feedback loop in the neural network. This is the difference between RNN and traditional neural network.
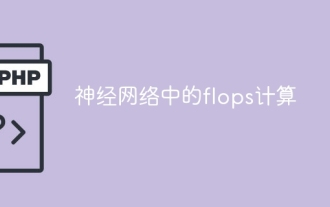
FLOPS is one of the standards for computer performance evaluation, used to measure the number of floating point operations per second. In neural networks, FLOPS is often used to evaluate the computational complexity of the model and the utilization of computing resources. It is an important indicator used to measure the computing power and efficiency of a computer. A neural network is a complex model composed of multiple layers of neurons used for tasks such as data classification, regression, and clustering. Training and inference of neural networks requires a large number of matrix multiplications, convolutions and other calculation operations, so the computational complexity is very high. FLOPS (FloatingPointOperationsperSecond) can be used to measure the computational complexity of neural networks to evaluate the computational resource usage efficiency of the model. FLOP
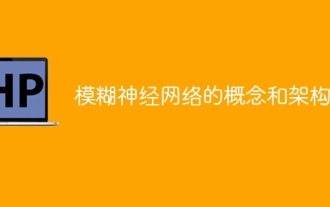
Fuzzy neural network is a hybrid model that combines fuzzy logic and neural networks to solve fuzzy or uncertain problems that are difficult to handle with traditional neural networks. Its design is inspired by the fuzziness and uncertainty in human cognition, so it is widely used in control systems, pattern recognition, data mining and other fields. The basic architecture of fuzzy neural network consists of fuzzy subsystem and neural subsystem. The fuzzy subsystem uses fuzzy logic to process input data and convert it into fuzzy sets to express the fuzziness and uncertainty of the input data. The neural subsystem uses neural networks to process fuzzy sets for tasks such as classification, regression or clustering. The interaction between the fuzzy subsystem and the neural subsystem makes the fuzzy neural network have more powerful processing capabilities and can
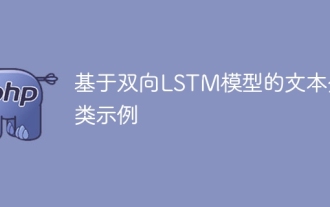
The bidirectional LSTM model is a neural network used for text classification. Below is a simple example demonstrating how to use bidirectional LSTM for text classification tasks. First, we need to import the required libraries and modules: importosimportnumpyasnpfromkeras.preprocessing.textimportTokenizerfromkeras.preprocessing.sequenceimportpad_sequencesfromkeras.modelsimportSequentialfromkeras.layersimportDense,Em

Siamese Neural Network is a unique artificial neural network structure. It consists of two identical neural networks that share the same parameters and weights. At the same time, the two networks also share the same input data. This design was inspired by twins, as the two neural networks are structurally identical. The principle of Siamese neural network is to complete specific tasks, such as image matching, text matching and face recognition, by comparing the similarity or distance between two input data. During training, the network attempts to map similar data to adjacent regions and dissimilar data to distant regions. In this way, the network can learn how to classify or match different data to achieve corresponding
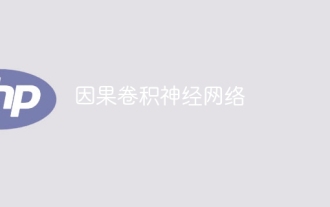
Causal convolutional neural network is a special convolutional neural network designed for causality problems in time series data. Compared with conventional convolutional neural networks, causal convolutional neural networks have unique advantages in retaining the causal relationship of time series and are widely used in the prediction and analysis of time series data. The core idea of causal convolutional neural network is to introduce causality in the convolution operation. Traditional convolutional neural networks can simultaneously perceive data before and after the current time point, but in time series prediction, this may lead to information leakage problems. Because the prediction results at the current time point will be affected by the data at future time points. The causal convolutional neural network solves this problem. It can only perceive the current time point and previous data, but cannot perceive future data.
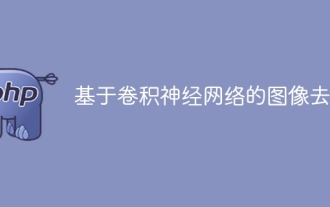
Convolutional neural networks perform well in image denoising tasks. It utilizes the learned filters to filter the noise and thereby restore the original image. This article introduces in detail the image denoising method based on convolutional neural network. 1. Overview of Convolutional Neural Network Convolutional neural network is a deep learning algorithm that uses a combination of multiple convolutional layers, pooling layers and fully connected layers to learn and classify image features. In the convolutional layer, the local features of the image are extracted through convolution operations, thereby capturing the spatial correlation in the image. The pooling layer reduces the amount of calculation by reducing the feature dimension and retains the main features. The fully connected layer is responsible for mapping learned features and labels to implement image classification or other tasks. The design of this network structure makes convolutional neural networks useful in image processing and recognition.
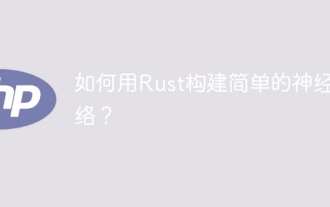
Rust is a systems-level programming language focused on safety, performance, and concurrency. It aims to provide a safe and reliable programming language suitable for scenarios such as operating systems, network applications, and embedded systems. Rust's security comes primarily from two aspects: the ownership system and the borrow checker. The ownership system enables the compiler to check code for memory errors at compile time, thus avoiding common memory safety issues. By forcing checking of variable ownership transfers at compile time, Rust ensures that memory resources are properly managed and released. The borrow checker analyzes the life cycle of the variable to ensure that the same variable will not be accessed by multiple threads at the same time, thereby avoiding common concurrency security issues. By combining these two mechanisms, Rust is able to provide
