Quickly develop web applications using SpringBoot and SpringMVC
Title: Rapidly develop web applications using Spring Boot and Spring MVC
Spring Boot and Spring MVC are modern Java web development frameworks that combine simplicity, efficiency and Flexible features can help developers quickly build reliable web applications. This article will introduce how to use Spring Boot and Spring MVC to develop a simple web application and provide specific code examples.
Environment settings
Before you start, you need to ensure that the following software has been installed on your computer:
- JDK 1.8 and above
- Maven 3 .x
- IDE (such as Eclipse or IntelliJ IDEA)
Create a Spring Boot project
First, we need to create a Spring Boot project. You can easily create a basic Spring Boot project skeleton using Maven commands or the IDE's create project wizard. When creating a project, you need to add relevant dependencies and plug-ins:
1 2 3 4 5 6 7 |
|
Create a controller
Next, we need to create a controller class to handle HTTP requests and responses. In Spring MVC, controllers are used to define URL routing and methods for handling requests.
1 2 3 4 5 6 7 8 |
|
In the above code, we use the @RestController
annotation to tell Spring that this is a controller class, and use the @GetMapping
annotation to define the processing/
URL method.
Start the application
Now, we need to write a startup class to start our Spring Boot application.
1 2 3 4 5 6 7 |
|
In the above code, we use the @SpringBootApplication
annotation to start the Spring Boot application and use the SpringApplication.run
method to run the application.
Testing the Application
Now we can launch the application and test our application by visiting http://localhost:8080
in the browser. If everything is OK, you will see a simple "Hello, world!" message.
Handling form submission
In addition to handling ordinary HTTP requests, Spring MVC can also handle form submission. The example below shows how to handle a simple form submission and store the data into the database.
First, we need to create a form page form.html
, including an input box and a submit button.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Then, we need to create a controller to handle the form submission and store the data into the database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
In the above code, the showForm
method is used to return the form page, while the saveData
method is used to handle form submission and store user data into the database . redirect:/form
is used to redirect to the form page.
Summary
By using Spring Boot and Spring MVC, we can quickly develop and deploy web applications. This article gives a simple example showing how to use Spring Boot and Spring MVC to handle HTTP requests and form submissions. I hope this article was helpful and I wish you success in web development!
The above are specific code examples for using Spring Boot and Spring MVC to quickly develop web applications. Through these examples, you can quickly get started with Spring Boot and Spring MVC, and develop efficient and flexible web applications. Happy coding!
The above is the detailed content of Quickly develop web applications using SpringBoot and SpringMVC. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
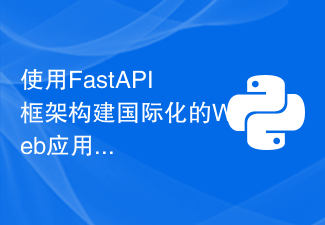
Use the FastAPI framework to build international Web applications. FastAPI is a high-performance Python Web framework that combines Python type annotations and high-performance asynchronous support to make developing Web applications simpler, faster, and more reliable. When building an international Web application, FastAPI provides convenient tools and concepts that can make the application easily support multiple languages. Below I will give a specific code example to introduce how to use the FastAPI framework to build
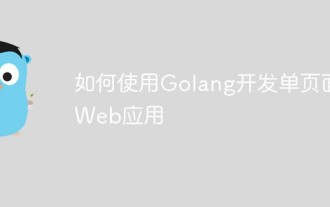
With the continuous development of the Internet, the demand for Web applications is also increasing. In the past, web applications were usually composed of multiple pages, but now more and more applications choose to use single page applications (SPA). Single-page applications are very suitable for mobile access, and users do not need to wait for the entire page to reload, which increases the user experience. In this article, we will introduce how to use Golang to develop SPA applications. What is a single page application? A single page application refers to a web application with only one HTML file. It uses Jav
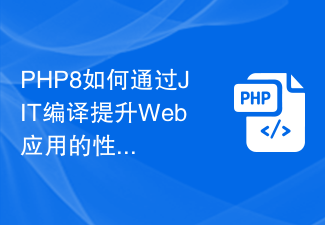
How does PHP8 improve the performance of web applications through JIT compilation? With the continuous development of Web applications and the increase in demand, improving the performance of Web applications has become one of the focuses of developers. As a commonly used server-side scripting language, PHP has always been loved by developers. The JIT (just-in-time compilation) compiler was introduced in PHP8, providing developers with a new performance optimization solution. This article will discuss in detail how PHP8 can improve the performance of web applications through JIT compilation, and provide specific code examples.
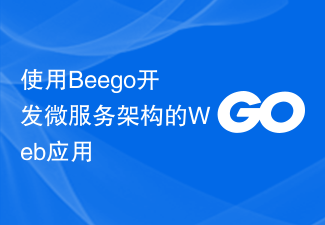
With the development of the Internet and the popularity of applications, the demand for Web applications has also continued to grow. In order to meet the needs of a large number of users, traditional web applications often face performance bottlenecks and scalability issues. In response to these problems, microservice architecture has gradually become a trend and solution for web application development. In the microservice architecture, the Beego framework has become the first choice of many developers. Its efficiency, flexibility, and ease of use are deeply loved by developers. This article will introduce the use of Beego framework to develop web applications with microservice architecture.
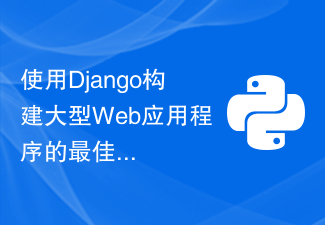
With the popularity and development of the Internet, Web applications have become an indispensable and important part of today's society. For large-scale web applications, an efficient, scalable, and maintainable framework is essential. Under such circumstances, Django has become a popular web framework because it adopts many best practices to help developers quickly build high-quality web applications. In this article, we will introduce some best practices for building large-scale web applications using Django.
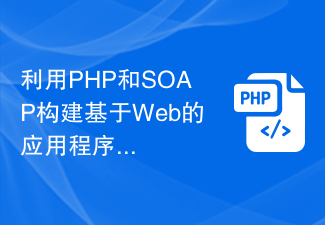
A complete guide to building web-based applications using PHP and SOAP In today's Internet era, web-based applications have become an important tool for managing and interacting with data. As a powerful development language, PHP can be seamlessly integrated with other technologies, while SOAP (Simple Object Access Protocol), as an XML-based communication protocol, provides us with a simple, standard and extensible method to build Web services. . This article will provide you with

With the continuous development of Internet technology, Web applications have become an indispensable part of people's lives and work. In web application development, choosing an appropriate framework can play an important role in improving development efficiency, speeding up development, and improving code quality. This article will analyze the choice of Go framework from three aspects: what is Go framework, the advantages of Go framework, and how to choose a suitable Go framework. 1. What is the Go framework? Go is an open source programming language ideal for building high-performance web applications.
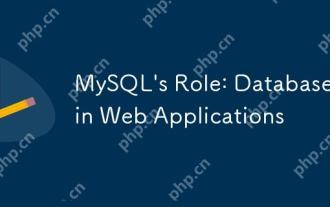
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
