


In-depth analysis of the working principle and performance optimization techniques of Golang slicing
Interpretation of Golang slicing principle: slicing operation methods and performance optimization techniques
Introduction:
Golang is a high-performance programming language, and its slice (slice ) is a very important and commonly used data structure. Slicing not only efficiently manipulates data, but also saves memory space. This article will provide an in-depth explanation of the principles of Golang slicing, introduce how to operate slicing, and share some performance optimization techniques.
1. The principle of slicing
In Golang, a slice is a reference to the underlying array, and it also contains the length and capacity information of the array. The underlying array of a slice typically grows or shrinks dynamically as data is added or removed.
When the length of the slice exceeds the capacity of the underlying array, the slice will automatically expand to double the capacity of the underlying array. This is because Golang adopts a dynamic expansion strategy in order to avoid frequent memory allocation and reduce the generation of memory fragmentation.
When expanding, slicing will reallocate a larger underlying array and copy the original data to the new underlying array. This process involves memory allocation and data copying, which consumes a certain amount of time and resources. Therefore, when using slicing, we should minimize the frequency of capacity expansion to improve performance.
2. How to operate slices
-
Create a slice
Use the make function to create a slice and specify the length and capacity of the slice. For example:slice := make([]int, 5, 10)
Copy after loginThe above code creates an int type slice with an initial length of 5 and a capacity of 10.
Interception of slices
We can intercept part of the data through the subscript of the slice. For example, we can intercept the first three elements of a slice:newSlice := slice[:3]
Copy after loginIn this way, we get a new slice containing the first three elements of the original slice.
Append to slice
Use the append function to append elements to a slice. For example:slice = append(slice, 15)
Copy after loginThe above code will append 15 to the end of the slice.
Copying of slices
Use the copy function to copy the contents of one slice to another slice. For example:slice2 := make([]int, len(slice)) copy(slice2, slice)
Copy after loginThe above code copies the contents of slice to slice2.
3. Performance optimization skills
- Pre-allocation of slices
When creating a slice, if we know the final length of the slice, we can specify the slice in advance capacity instead of the default capacity. This can avoid frequent expansion operations and improve performance. - Reuse slices
If we need to use slices multiple times in a loop, we can consider reusing slices. By reassigning the length of the slice, you can reuse the existing underlying array, avoid frequent memory allocation and memory copy, and improve performance. - Use copy instead of append
When appending elements, if we already know the number of newly added elements, we can first expand the capacity of the underlying array, and then use the copy function to copy the new elements into the slice. This can avoid frequent expansion operations and improve performance. - Reasonably set the capacity of the slice
If we know the maximum capacity of the slice, we can directly specify the capacity of the slice when creating the slice to avoid frequent expansion of the underlying array and improve performance.
Conclusion:
Slicing is a very useful data structure in Golang. By understanding the principle of slicing, we can better use and optimize the operation method of slicing. In actual development, program performance can be improved by properly pre-allocating slices, reusing slices, using the copy function instead of appending, and setting the capacity of slices appropriately. I hope this article can help readers deeply understand the principles of Golang slicing and provide performance optimization skills.
The above is the detailed content of In-depth analysis of the working principle and performance optimization techniques of Golang slicing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


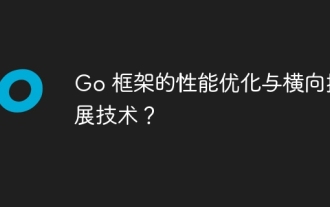
In order to improve the performance of Go applications, we can take the following optimization measures: Caching: Use caching to reduce the number of accesses to the underlying storage and improve performance. Concurrency: Use goroutines and channels to execute lengthy tasks in parallel. Memory Management: Manually manage memory (using the unsafe package) to further optimize performance. To scale out an application we can implement the following techniques: Horizontal Scaling (Horizontal Scaling): Deploying application instances on multiple servers or nodes. Load balancing: Use a load balancer to distribute requests to multiple application instances. Data sharding: Distribute large data sets across multiple databases or storage nodes to improve query performance and scalability.
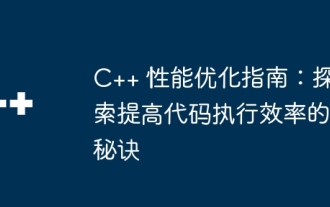
C++ performance optimization involves a variety of techniques, including: 1. Avoiding dynamic allocation; 2. Using compiler optimization flags; 3. Selecting optimized data structures; 4. Application caching; 5. Parallel programming. The optimization practical case shows how to apply these techniques when finding the longest ascending subsequence in an integer array, improving the algorithm efficiency from O(n^2) to O(nlogn).
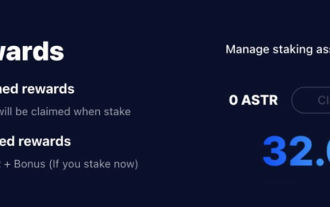
Table of Contents Astar Dapp Staking Principle Staking Revenue Dismantling of Potential Airdrop Projects: AlgemNeurolancheHealthreeAstar Degens DAOVeryLongSwap Staking Strategy & Operation "AstarDapp Staking" has been upgraded to the V3 version at the beginning of this year, and many adjustments have been made to the staking revenue rules. At present, the first staking cycle has ended, and the "voting" sub-cycle of the second staking cycle has just begun. To obtain the "extra reward" benefits, you need to grasp this critical stage (expected to last until June 26, with less than 5 days remaining). I will break down the Astar staking income in detail,
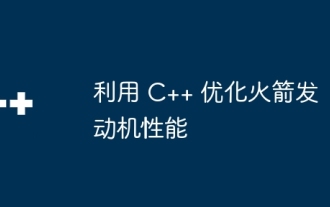
By building mathematical models, conducting simulations and optimizing parameters, C++ can significantly improve rocket engine performance: Build a mathematical model of a rocket engine and describe its behavior. Simulate engine performance and calculate key parameters such as thrust and specific impulse. Identify key parameters and search for optimal values using optimization algorithms such as genetic algorithms. Engine performance is recalculated based on optimized parameters to improve its overall efficiency.
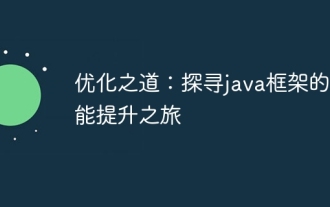
The performance of Java frameworks can be improved by implementing caching mechanisms, parallel processing, database optimization, and reducing memory consumption. Caching mechanism: Reduce the number of database or API requests and improve performance. Parallel processing: Utilize multi-core CPUs to execute tasks simultaneously to improve throughput. Database optimization: optimize queries, use indexes, configure connection pools, and improve database performance. Reduce memory consumption: Use lightweight frameworks, avoid leaks, and use analysis tools to reduce memory consumption.
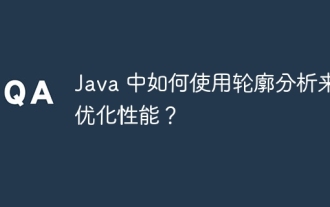
Profiling in Java is used to determine the time and resource consumption in application execution. Implement profiling using JavaVisualVM: Connect to the JVM to enable profiling, set the sampling interval, run the application, stop profiling, and the analysis results display a tree view of the execution time. Methods to optimize performance include: identifying hotspot reduction methods and calling optimization algorithms
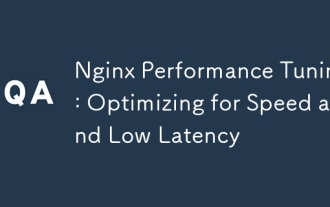
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
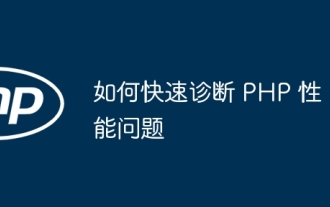
Effective techniques for quickly diagnosing PHP performance issues include using Xdebug to obtain performance data and then analyzing the Cachegrind output. Use Blackfire to view request traces and generate performance reports. Examine database queries to identify inefficient queries. Analyze memory usage, view memory allocations and peak usage.
