Practical method of Chinese rewriting: implemented with Java software
A practical method of using Java software for Chinese rewriting requires specific code examples
In today's era of highly developed information, we often need to quickly obtain and process a large amount of text information. Among them, Chinese rewriting is a common requirement and can be used in application scenarios such as text deduplication, text similarity calculation, and text summary generation. In this article, we will introduce how to use Java software for Chinese rewriting and give specific code examples.
Chinese rewriting is to adjust the structure, semantics, vocabulary, etc. of the input Chinese sentence or text so that the rewritten text has a similar meaning to the original text, but with some changes. Specifically, we can achieve Chinese rewriting by replacing synonyms, adjusting sentence structure, changing word order, etc.
In order to achieve Chinese rewriting, we can use Java's natural language processing library, such as HanLP or NLPIR. The following is a sample code that uses HanLP for Chinese rewriting:
import com.hankcs.hanlp.HanLP; import com.hankcs.hanlp.seg.common.Term; import com.hankcs.hanlp.tokenizer.StandardTokenizer; import java.util.ArrayList; import java.util.List; public class ChineseParaphrase { public static String chineseToPinyin(String sentence) { List<Term> termList = StandardTokenizer.segment(sentence); StringBuilder sb = new StringBuilder(); for (Term term : termList) { sb.append(term.word).append(" "); } return sb.toString().trim(); } public static String paraphrase(String sentence) { List<String> pinyinList = new ArrayList<>(); List<Term> termList = StandardTokenizer.segment(sentence); for (Term term : termList) { String pinyin = HanLP.convertToPinyinString(term.word, " ", false); pinyinList.add(pinyin); } return String.join("", pinyinList); } public static void main(String[] args) { String sentence = "我爱中国"; String pinyin = chineseToPinyin(sentence); String paraphrase = paraphrase(sentence); System.out.println("拼音转换:" + pinyin); System.out.println("改写结果:" + paraphrase); } }
In the above code, we first use HanLP's standard word segmenter to segment the input sentence and obtain a word list. Then, use HanLP to convert each word into pinyin and save the results in a list. Finally, we concatenate all the pinyin in the list into a string, which is the rewritten result.
Take the input sentence "I love China" as an example, use the above code to rewrite it, the output result is as follows:
Pinyin conversion:
wo ai zhong guo
Rewritten result:
woai zhongguo
As you can see, the original sentence has been rewritten in Chinese and turned into pinyin. This is just a simple example of Chinese rewriting. In fact, Chinese rewriting can be more complex and flexible, and can be adjusted accordingly according to specific needs.
In addition to HanLP, there are other Chinese natural language processing libraries that can implement Chinese rewriting, such as NLPIR, jieba, etc. Using these libraries, we can use functions such as word segmentation, part-of-speech tagging, and keyword extraction to achieve more changes in Chinese rewriting.
To sum up, using Java software for Chinese rewriting is a practical technology that can be applied to all aspects of text processing. By rationally using the Chinese natural language processing library, we can easily implement Chinese rewriting and flexibly adjust it according to specific needs. I hope the sample code in this article will be helpful to readers.
The above is the detailed content of Practical method of Chinese rewriting: implemented with Java software. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
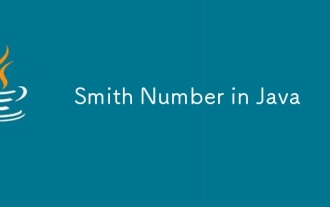
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
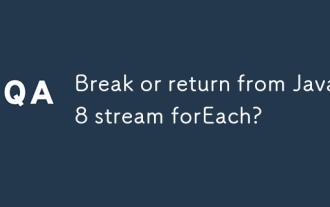
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
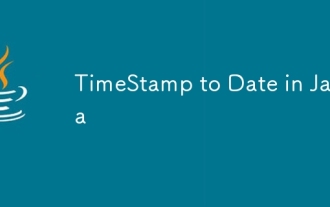
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
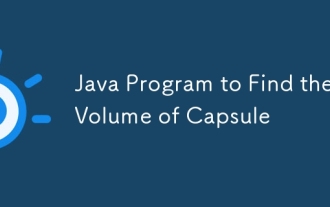
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
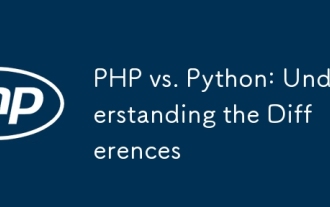
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
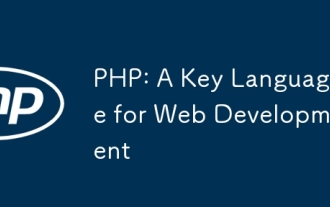
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
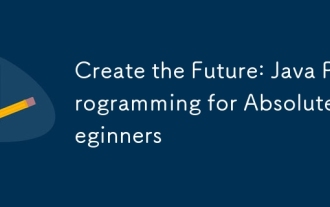
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
