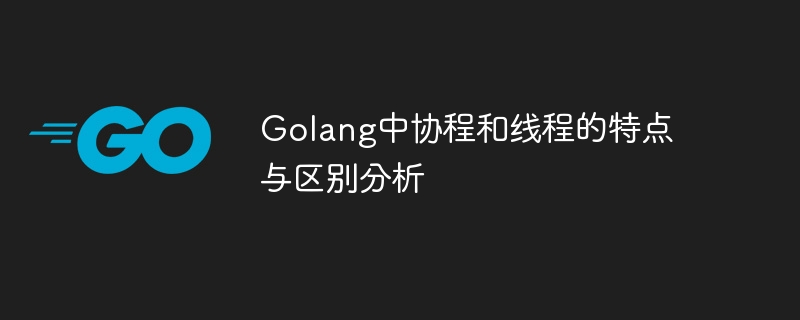
Analysis of the characteristics and differences between coroutines and threads in Golang
1. Introduction
Golang is a modern programming language with its simplicity, efficiency and Known for concurrency. In Golang, coroutines and threads are the two main ways to implement concurrent programming. This article will analyze the characteristics and differences between coroutines and threads, and provide specific code examples.
2. Characteristics of coroutines
- Lightweight
Coroutines are lightweight execution units. Each coroutine requires only a small amount of memory (about 2KB ), so more coroutines can be created under the same hardware resources.
- Concurrency
Coroutines in Golang are executed concurrently, which means that multiple coroutines can be executed at the same time. By using coroutines, we can implement highly concurrent programs and improve program execution efficiency.
- Communication mechanism
Golang provides channel as a communication mechanism between coroutines. By using channels, data can be transmitted and shared safely between coroutines, avoiding the problem of shared data in traditional multi-threaded programming.
- Scheduler
Golang's scheduler will automatically allocate coroutines to different threads for execution to achieve concurrency. The scheduler has intelligent scheduling and task switching capabilities, and can make full use of the system's multi-core processing capabilities.
- Lock Mechanism
Coroutines do not need to explicitly use the lock mechanism to ensure the synchronization of shared data. By using channels for communication, mutually exclusive access between coroutines can be guaranteed.
3. Sample code of coroutine
package main
import (
"fmt"
"time"
)
func worker(name string, ch <-chan string) {
for msg := range ch {
fmt.Printf("%s received message: %s
", name, msg)
time.Sleep(1 * time.Second)
}
}
func main() {
ch := make(chan string)
go worker("Worker 1", ch)
go worker("Worker 2", ch)
go worker("Worker 3", ch)
ch <- "Hello"
ch <- "World"
time.Sleep(3 * time.Second)
close(ch)
fmt.Println("All messages have been sent.")
}
Copy after login
4. Characteristics of threads
- Heavyweight
Threads are execution units at the operating system level , each thread requires a large memory overhead (usually several MB), so under the same hardware resources, the number of threads created is limited. - Concurrency
Threads execute concurrently, but in multi-threaded programming, due to the need to explicitly use the lock mechanism to ensure the synchronization of shared data, problems such as deadlocks and race conditions are prone to occur. - Scheduler
The operating system is responsible for thread scheduling. The scheduler is usually based on time slice rotation, which can easily lead to increased context switching overhead. - Lock Mechanism
Thread programming requires explicit use of locks to ensure synchronization of shared data, which increases the complexity of programming and easily causes a series of problems.
5. Thread sample code
package main
import (
"fmt"
"sync"
"time"
)
var mutex sync.Mutex
func worker(name string, ch <-chan string) {
for msg := range ch {
mutex.Lock()
fmt.Printf("%s received message: %s
", name, msg)
mutex.Unlock()
time.Sleep(1 * time.Second)
}
}
func main() {
ch := make(chan string)
go worker("Worker 1", ch)
go worker("Worker 2", ch)
go worker("Worker 3", ch)
ch <- "Hello"
ch <- "World"
time.Sleep(3 * time.Second)
close(ch)
fmt.Println("All messages have been sent.")
}
Copy after login
6. Summary of the difference between coroutines and threads
- Lightweight vs heavyweight: Coroutines are A lightweight execution unit, each coroutine requires little memory, while threads require large memory overhead.
- Concurrency: Coroutines are executed concurrently and can efficiently utilize hardware resources, while thread programming requires explicit use of lock mechanisms to ensure synchronization of shared data.
- Scheduler: Golang's scheduler will automatically allocate coroutines to different threads for execution, and has the ability to intelligently schedule. The operating system is responsible for thread scheduling, and context switching is expensive.
- Lock mechanism: Coroutines achieve safe data sharing and transmission by using channels. There is no need to explicitly use the lock mechanism, and threads need to use locks to ensure the synchronization of shared data.
7. Conclusion
In Golang, coroutine is an efficient concurrent programming mechanism, which has smaller memory overhead, higher concurrency and safer than traditional thread programming. of data sharing. By using coroutines appropriately, you can write scalable, highly concurrent programs. However, threaded programming is still a viable option in certain application scenarios, especially when integration with other languages or direct manipulation of operating system-level resources is required.
The above is the detailed content of Characteristics and differences analysis of coroutines and threads in Golang. For more information, please follow other related articles on the PHP Chinese website!