


Summary of JavaScript types, values and variables_javascript skills
Foreword: JavaScript data types are divided into two categories: primitive types and object types. 5 primitive types: number, string, Boolean value, null (empty), undefined (undefined). An object is a collection of attributes, and each attribute is composed of a "name/value pair" (the value can be a primitive value or an object). Three special objects: global objects, arrays, and functions. The core of the JavaScript language also defines three useful classes: date (Date) class, regular (RegExp) class, and error (Error) class.
1 Number
JavaScript does not distinguish between integer values and floating point values. JavaScript can recognize decimal integer literals (the so-called literals are data values directly used in the program), and hexadecimal values (prefixed with 0x or 0X, which is the number 0 and not the letter o. Think about it if it is the letter o If so, then the hexadecimal value becomes an identifier.) Although the ECMAScript standard does not support octal literals, some implementations of JavaScript can use octal form to represent integers (prefixed with the number 0). The author uses octal to represent an integer in the three browsers of IE, Chrome, and FF on my computer. Variable assignment is no problem either. However, in the strict mode of ECMAScript6, octal literals are explicitly prohibited.
There are two ways to write floating point literals. ① Traditional way of writing real numbers: It consists of an integer part, a decimal point and a decimal part; ② Exponent counting method: that is, the real number is followed by the letter e or E, followed by a positive or negative sign, and then followed by an integer exponent.
1.1 Overflow of arithmetic operations
Arithmetic operations in JavaScript will not report errors when overflowing, underflowing, or being divisible by 0.
Overflow: When the operation result exceeds the upper limit of the number that JavaScript can represent, the result is positive infinity or negative infinity-Infinity. The behavioral characteristics of infinity values are also consistent with reality: the results of addition, subtraction, multiplication and division operations based on them are still infinite values (of course retaining their signs); underflow: when the operation result is infinitely close to zero and is smaller than the smallest value that JavaScript can represent What happened when the value was still small. In this case, 0 will be returned. The special value "negative zero" is returned when a negative number underflows. Negative zero and whole zero are essentially equal (can even be tested using strict equality ===), except as divisors:
var zero = 0; //正零值 var negz = -0; //负零值 zero === negz //表达式返回值为true 1/zero === 1/negz //表达式返回值false,等价于判断正无穷大和负无穷大是否严格相等
Divisibility by 0 will return positive infinity or negative infinity. But dividing 0 by 0 will return NaN (the value of the NaN property of the JavaScript predefined object Number). There are four situations where NaN is returned: ① 0 divided by 0 ② infinity divided by infinity ③ performing a square root operation on any negative number ④ when arithmetic operators are used with operands that are not numbers or cannot be converted to numbers.
There is something special about the NaN value: it is not equal to any value, including itself. There are two ways to judge whether a variable x is NaN: ① Use the function isNaN() ② Use x != x to judge. The expression result is true if and only if x is NaN. There is a similar function isFinite() in JavaScript, which returns true when the parameter is not NaN, Infinity or -Infinity.
1.2 Binary floating point numbers and rounding errors
There are countless real numbers, but JavaScript can only represent a limited number of them in the form of floating point numbers. In other words, when using real numbers in JavaScript, they are often just an approximate representation of a real value. JavaScript uses IEEE-754 floating point number representation, which is a binary representation that can accurately represent fractions such as 1/2, 1/8, and 1/1024, but decimal fractions 1/10, 1/10 etc. cannot be expressed accurately. For example:
var x = 0.3 -0.2; //x=0.09999999999999998 var y = 0.2 - 0.1; // y=0.1 x == y //false x == 0.1 //false y == 0.1 //true 0.1 == 0.1 //true var z = x + y; //z=0.19999999999999998
2 文本
2.1 字符串、字符集
字符串(string)是一组由16位值组成的不可变的有序序列,每个字符通常来自于Unicode字符集。字符串的长度(length)是其所含16位值得个数。JavaScript通过字符串类型来表示文本。注意:JavaScript中并没有表示单个字符的“字符型”。要表示一个16位值,只需将其赋值给字符串变量即可。
JavaScript采用UTF-16编码的Unicode字符集,JavaScript字符串是由一组无符号的16位值组成的序列。那些不能表示为16位的Unicode字符则遵循UTF-16编码规则——用两个16位值组成一个序列(或称作“代理项对”)表示。这意味着一个长度为2的JavaScript字符串有可能表示一个Unicode字符。注意:JavaScript定义的各式字符串的操作方法均作用于16位值,而非字符,且不会对代理项对做单独处理。书看到这里,又结合http://www.alloyteam.com/2013/12/js-calculate-the-number-of-bytes-occupied-by-a-string/上面所述,终于对Unicode字符集、UTF-8、UTF-16稍有理解。
字符串的定界符可以是单引号或者双引号。这两种形式的定界符可以嵌套,但是不能多层嵌套(比如,双引号可以包含单引号,这时单引号中不能再包含双引号了)。正如上篇所说,一个字符串值可以拆分为数行,每行必须以反斜线(\)结束,这时反斜线和行结束符都不算是字符串内容,即字符串本身并非是多行,只是写成了多行的形式。
注意:①在JavaScript中字符串是固定不变的(除非重新赋值),类似replace()和toUpperCase()的方法都返回新字符串,原字符串本身并没有变化;②字符串可以当做只读数组,除了使用charAt()方法来查询一个单一字符,也可以使用方括号的方式来访问字符串中的单个字符(16位值),例如:
s = "hello, world"; s[0] //=>"h"
2.2 Escape character
Escape character meaning
o NUL character (u0000)
b Backspace character (u0008)
t Horizontal tab (u0009)
n newline character (u000A)
v Vertical tab (u000B)
f form feed character (u000C)
r carriage return (u000D)
" Double quotes (u0022)
' Apostrophe or single quote (u0027)
\ Backslash(u005C)
xXX Latin-1 character specified by the two-digit hexadecimal number XX
uXXXX Unicode character specified by 4-digit hexadecimal number XXXX
Note: If the "" character precedes a character not listed in the table, the "" character is ignored. For example, "#" and "#" are equivalent. Don’t forget that backslashes also have a role in using backslashes at the end of each line in multi-line strings.
3 Boolean value
Values in JavaScript can be converted to Boolean values. Among them, null, undefined, 0, -0, NaN, "" (empty string), these 6 values will be converted to false, false and these six values are sometimes called "false values"; all other values, including Objects (arrays) will be converted to true, and true and these values are called "true values". Note: Boolean contains the toString() method, so you can use this method to convert a string to "true" or "false", but it does not contain other useful methods.The above is the entire content of this article, I hope you all like it.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
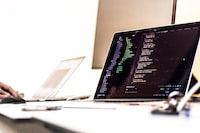
Python is widely used in a wide range of fields with its simple and easy-to-read syntax. It is crucial to master the basic structure of Python syntax, both to improve programming efficiency and to gain a deep understanding of how the code works. To this end, this article provides a comprehensive mind map detailing various aspects of Python syntax. Variables and Data Types Variables are containers used to store data in Python. The mind map shows common Python data types, including integers, floating point numbers, strings, Boolean values, and lists. Each data type has its own characteristics and operation methods. Operators Operators are used to perform various operations on data types. The mind map covers the different operator types in Python, such as arithmetic operators, ratio
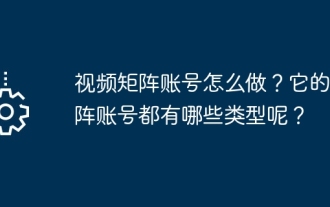
With the popularity of short video platforms, video matrix account marketing has become an emerging marketing method. By creating and managing multiple accounts on different platforms, businesses and individuals can achieve goals such as brand promotion, fan growth, and product sales. This article will discuss how to effectively use video matrix accounts and introduce different types of video matrix accounts. 1. How to create a video matrix account? To make a good video matrix account, you need to follow the following steps: First, you must clarify what the goal of your video matrix account is, whether it is for brand communication, fan growth or product sales. Having clear goals helps develop strategies accordingly. 2. Choose a platform: Choose an appropriate short video platform based on your target audience. The current mainstream short video platforms include Douyin, Kuaishou, Huoshan Video, etc.
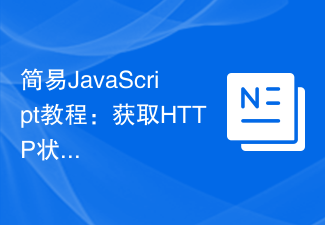
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
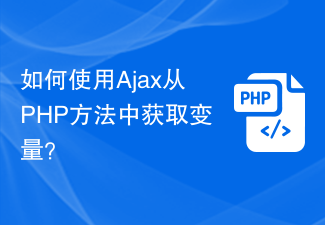
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
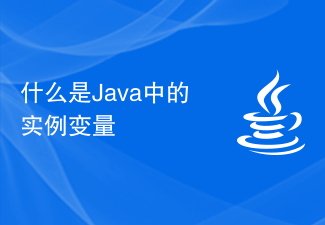
Instance variables in Java refer to variables defined in the class, not in the method or constructor. Instance variables are also called member variables. Each instance of a class has its own copy of the instance variable. Instance variables are initialized during object creation, and their state is saved and maintained throughout the object's lifetime. Instance variable definitions are usually placed at the top of the class and can be declared with any access modifier, which can be public, private, protected, or the default access modifier. It depends on what we want this to be
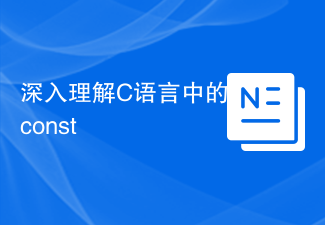
Detailed explanation and code examples of const in C In C language, the const keyword is used to define constants, which means that the value of the variable cannot be modified during program execution. The const keyword can be used to modify variables, function parameters, and function return values. This article will provide a detailed analysis of the use of the const keyword in C language and provide specific code examples. const modified variable When const is used to modify a variable, it means that the variable is a read-only variable and cannot be modified once it is assigned a value. For example: constint
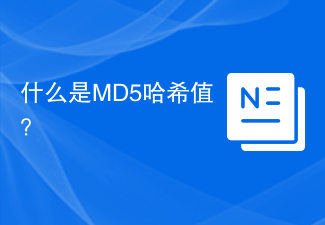
What is the MD5 value? In computer science, MD5 (MessageDigestAlgorithm5) is a commonly used hash function used to digest or encrypt messages. It produces a fixed-length 128-bit binary number, usually represented in 32-bit hexadecimal. The MD5 algorithm was designed by Ronald Rivest in 1991. Although the MD5 algorithm is considered no longer secure in the field of cryptography, it is still widely used in data integrity verification and file verification.
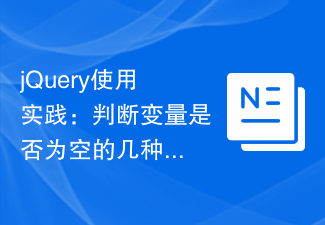
jQuery is a JavaScript library widely used in web development. It provides many simple and convenient methods to operate web page elements and handle events. In actual development, we often encounter situations where we need to determine whether a variable is empty. This article will introduce several common methods of using jQuery to determine whether a variable is empty, and attach specific code examples. Method 1: Use the if statement to determine varstr="";if(str){co
