


How to increase the dimensions of an array in numpy: detailed steps
Jan 26, 2024 am 08:02 AMDetailed steps and code examples for adding dimensions in numpy
Introduction:
In data analysis and scientific computing, numpy is a widely used Python library , which provides efficient multi-dimensional array operation functions. In practical applications, it is often necessary to increase the dimension of an array to meet specific needs. This article will introduce the detailed steps of adding dimensions in numpy and provide specific code examples.
- Using the reshape function
The reshape function in numpy can be used to change the shape of an array, including increasing dimensions. The following is a sample code that uses the reshape function to increase the dimension:
import numpy as np # 定义一个二维数组 arr1 = np.array([[1, 2, 3], [4, 5, 6]]) # 使用reshape函数增加维度 arr2 = arr1.reshape((2, 3, 1)) print(arr2.shape) # 输出:(2, 3, 1) print(arr2) # 输出: # [[[1] # [2] # [3]] # [[4] # [5] # [6]]]
In the sample code, a two-dimensional array arr1 is first defined, and then the reshape function is used to modify its shape to (2, 3, 1 ), which adds a dimension. Finally, the shape and content of the modified array are output.
- Using the expand_dims function
The expand_dims function in numpy can be used to add dimensions at a specified position. The following is a sample code that uses the expand_dims function to add dimensions:
import numpy as np # 定义一个二维数组 arr1 = np.array([[1, 2, 3], [4, 5, 6]]) # 使用expand_dims函数增加维度 arr2 = np.expand_dims(arr1, axis=2) print(arr2.shape) # 输出:(2, 3, 1) print(arr2) # 输出: # [[[1] # [2] # [3]] # [[4] # [5] # [6]]]
In the sample code, a two-dimensional array arr1 is first defined, and then the expand_dims function is used to add a dimension at axis=2. Finally, the shape and content of the modified array are output.
- Use the newaxis keyword
The newaxis keyword in numpy can be used to increase dimensions. The following is a sample code that uses the newaxis keyword to increase the dimension:
import numpy as np # 定义一个二维数组 arr1 = np.array([[1, 2, 3], [4, 5, 6]]) # 使用newaxis关键字增加维度 arr2 = arr1[..., np.newaxis] print(arr2.shape) # 输出:(2, 3, 1) print(arr2) # 输出: # [[[1] # [2] # [3]] # [[4] # [5] # [6]]]
In the sample code, a two-dimensional array arr1 is first defined, and then the newaxis keyword is used to modify its shape to (2, 3 , 1), which adds one dimension. Finally, the shape and content of the modified array are output.
To sum up, this article introduces three methods and code examples for dimension increase operations in numpy. Readers can choose the appropriate method to add dimensions according to actual needs to meet their own data processing needs. Numpy's powerful functions and concise coding style make it an indispensable tool in data analysis and scientific computing.
The above is the detailed content of How to increase the dimensions of an array in numpy: detailed steps. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
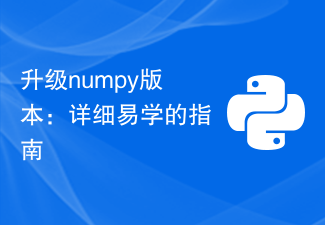
Upgrading numpy versions: a detailed and easy-to-follow guide
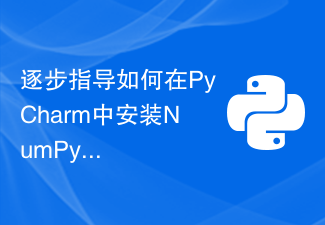
Step-by-step guide on how to install NumPy in PyCharm and get the most out of its features
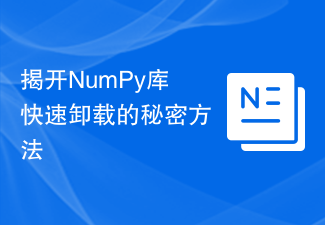
Uncover the secret method to quickly uninstall the NumPy library
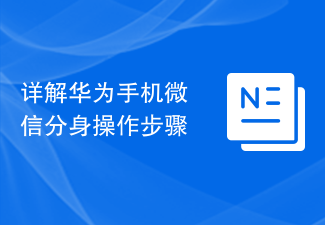
Detailed explanation of the operation steps of WeChat clone on Huawei mobile phone

Numpy installation guide: Solving installation problems in one article
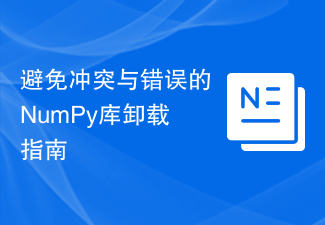
Guide to uninstalling the NumPy library to avoid conflicts and errors
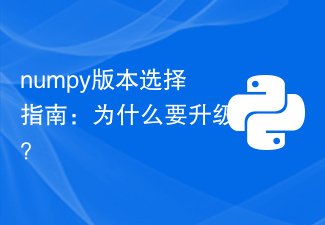
Numpy version selection guide: why upgrade?
