Easy-to-understand Tensor and Numpy conversion guide
Simple and easy-to-understand Tensor and Numpy conversion tutorial, specific code examples are required
Introduction:
In machine learning and deep learning, Tensorflow (TF for short) is a very popular deep learning library, and Numpy (Numerical Python) is an important library for scientific computing in Python. The underlying implementation of Tensorflow is Tensor, while Numpy uses multi-dimensional arrays. Due to the differences in data structures between Tensorflow and Numpy, we usually need to convert data types between the two. This article will introduce how to convert between Tensorflow and Numpy and provide specific code examples.
1. Convert Tensor to Numpy array
When we need to convert a Tensor to a Numpy array, we can use the numpy()
function provided by Tensorflow. The following is a simple example:
import tensorflow as tf import numpy as np # 创建一个Tensor tensor = tf.constant([[1, 2, 3], [4, 5, 6]]) # 将Tensor转换为Numpy数组 numpy_array = tensor.numpy() print(numpy_array)
In the above code, we first import the tensorflow
and numpy
libraries. Then, we created a 2x3 Tensor using the constant
function. Next, we use the numpy()
function to convert the Tensor to a Numpy array and assign the result to the numpy_array
variable. Finally, the result is output through the print
function.
2. Convert Numpy array to Tensor
When we need to convert a Numpy array to Tensor, we can use the convert_to_tensor()
function. The following is a simple example:
import tensorflow as tf import numpy as np # 创建一个Numpy数组 numpy_array = np.array([[1, 2, 3], [4, 5, 6]]) # 将Numpy数组转换为Tensor tensor = tf.convert_to_tensor(numpy_array) print(tensor)
In the above code, we first import the tensorflow
and numpy
libraries. Then, we created a 2x3 Numpy array, using the array
function. Next, we use the convert_to_tensor()
function to convert the Numpy array to Tensor and assign the result to the tensor
variable. Finally, the result is output through the print
function.
3. Sharing data between Tensor and Numpy
In actual use, we may need to share data between Tensor and Numpy, which can be achieved by modifying the value of the Tensor or Numpy array. The following is a simple example:
import tensorflow as tf import numpy as np # 创建一个Tensor tensor = tf.constant([[1, 2, 3], [4, 5, 6]]) # 将Tensor转换为Numpy数组 numpy_array = tensor.numpy() # 在Numpy数组上进行修改 numpy_array[0, 0] = 10 # 在Tensor上查看修改后的结果 print(tensor) # 在Tensor上进行修改 tensor[0, 1] = 20 # 在Numpy数组上查看修改后的结果 print(numpy_array)
In the above code, we first import the tensorflow
and numpy
libraries. Then, we created a 2x3 Tensor using the constant
function. Next, we use the numpy()
function to convert the Tensor to a Numpy array and assign the result to the numpy_array
variable. Then, we modified the value of the first element on the Numpy array and viewed the modified Tensor through the print
function. Next, we modified the value of the first element on the Tensor and viewed the modified Numpy array through the print
function.
Conclusion:
This article explains how to convert between Tensor and Numpy and provides specific code examples. Through the above examples, we can easily perform data type conversion between Tensor and Numpy, which facilitates data processing and analysis in machine learning and deep learning. Hope this article helps you!
The above is the detailed content of Easy-to-understand Tensor and Numpy conversion guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
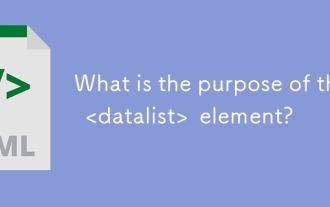
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
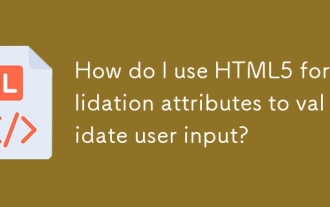
The article discusses using HTML5 form validation attributes like required, pattern, min, max, and length limits to validate user input directly in the browser.
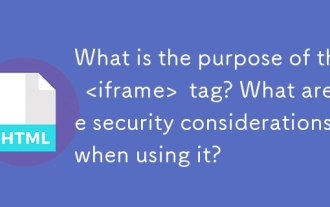
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.
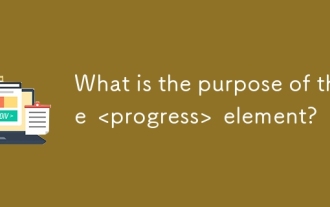
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
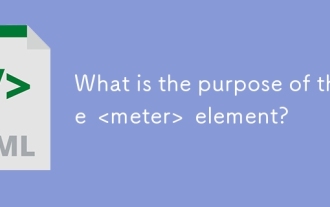
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
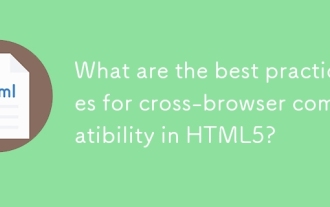
Article discusses best practices for ensuring HTML5 cross-browser compatibility, focusing on feature detection, progressive enhancement, and testing methods.
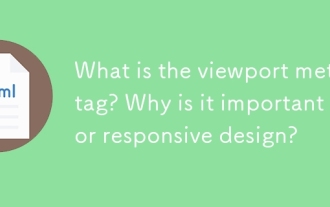
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
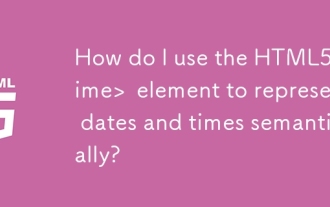
This article explains the HTML5 <time> element for semantic date/time representation. It emphasizes the importance of the datetime attribute for machine readability (ISO 8601 format) alongside human-readable text, boosting accessibilit
