How to use Java generics
Java generics mainly include "define generic classes", "define generic interfaces", "define generic methods", "instantiate generic classes or interfaces", "use wildcards" and "use generics". Six uses of "type qualification": 1. Define a generic class and use
to represent type parameters; 2. Define a generic interface and use to represent type parameters; 3. Define a generic method , use to represent type parameters; 4. When instantiating a generic class or interface, specify specific type parameters; 5. Use wildcards to represent a subtype or supertype of a certain generic type.
Java generics are mainly used in the following ways:
- Define generic classes
You can define a generic class, use
public class MyList<T> { private T[] array; public MyList(T[] array) { this.array = array; } public T get(int index) { return array[index]; } }
- Define a generic interface
You can define a generic interface, use
public interface MyInterface<T> { T doSomething(); }
- Define a generic method
You can define a generic method, use
public <T> T doSomething(T param) { // ... }
- Instantiate a generic class or interface
When instantiating a generic class or interface, specific type parameters must be specified, for example:
MyList<String> list = new MyList<>(new String[]{"a", "b", "c"});
- Use wildcards
You can use wildcards to represent subtypes or supertypes of a certain generic type, including ?, ? extends T and ? super T. , for example:
MyList<? extends Number> list1 = new MyList<>(new Integer[]{1, 2, 3}); MyList<? super Integer> list2 = new MyList<>(new Number[]{1.0, 2.0, 3.0});
Among them, list1 can accept any type that is a subtype of Number (such as Integer, Float, etc.) as an element, while list2 can accept any type that is a supertype of Integer (such as Number, Object, etc.) as elements.
- Using generic qualification
You can use generic qualification to limit the scope of type parameters, including extends and super, for example:
public <T extends Number> void doSomething(T param) { // ... }
Among them,
The above is the detailed content of How to use Java generics. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


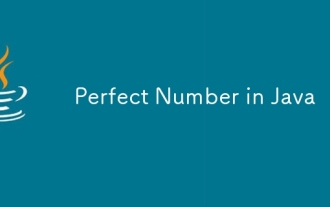
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
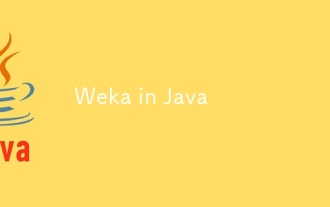
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
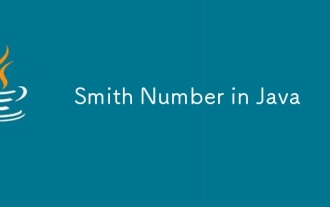
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
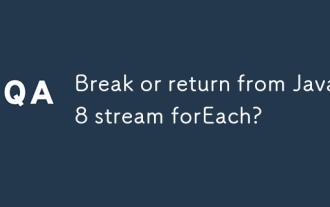
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
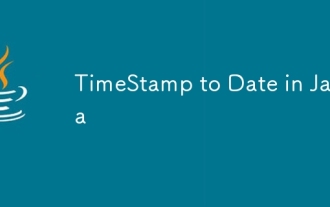
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
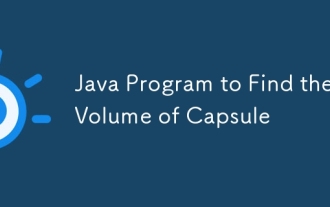
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
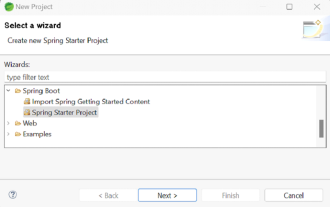
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
