In-depth exploration of the connotation of polymorphism in Golang
In-depth understanding of polymorphism in Golang requires specific code examples
Golang is an open source programming language with high performance and concurrency features. It is also a statically typed language and does not support the inheritance mechanism found in traditional object-oriented languages. However, through the use of interfaces, developers can achieve polymorphism in Golang.
Polymorphism is an important concept in object-oriented programming, which allows the same operation to be performed on different objects, and which specific implementation is performed based on the type of the actual object. In Golang, polymorphism is achieved through interfaces, which will be introduced in detail through sample code below.
First, we define an interface Shape, which contains a method Area() float64, which is used to calculate the area of the shape.
type Shape interface { Area() float64 }
Then, we create two structures, Circle and Rectangle, which respectively implement the Shape interface and provide their respective Area methods.
type Circle struct { radius float64 } type Rectangle struct { width float64 height float64 } func (c Circle) Area() float64 { return math.Pi * c.radius * c.radius } func (r Rectangle) Area() float64 { return r.width * r.height }
Next, we create a function PrintArea, which receives a parameter of type Shape and prints the area by calling its Area method.
func PrintArea(s Shape) { fmt.Printf("Area of shape is: %f ", s.Area()) }
Now, we can create Circle and Rectangle objects and pass them as parameters to the PrintArea function to test the effect of polymorphism.
func main() { c := Circle{radius: 3.0} r := Rectangle{width: 4.0, height: 5.0} PrintArea(c) PrintArea(r) }
In the sample code above, we created a circle with a radius of 3 and a rectangle with a width of 4 and a height of 5. Then, we pass them as parameters to the PrintArea function respectively. Since both the Circle and Rectangle types implement the Shape interface and both provide implementations of the Area method, the PrintArea function can correctly calculate and print out the area of the shape regardless of whether it is a circle or a rectangle.
Through this example, we can clearly see how polymorphism is implemented in Golang. Interfaces are the key to achieving polymorphism in Golang. By defining interfaces and implementing interface methods on different types, we can allow objects of different types to implement the same operation, thus achieving the effect of polymorphism.
In summary, polymorphism in Golang is achieved through the use of interfaces. An interface defines a set of methods, and a type can be considered an implementation type of the interface as long as it implements the methods defined in the interface. By assigning different types of objects to variables of the interface type and calling interface methods, polymorphic operations on different types of objects can be achieved.
Of course, this is just a simple example of polymorphism in Golang. In practical applications, polymorphism can also be used in combination with other features such as encapsulation and inheritance to further improve the scalability and maintainability of the code.
The above is the detailed content of In-depth exploration of the connotation of polymorphism in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
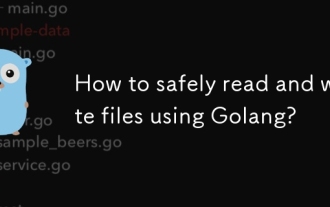
How to safely read and write files using Golang?
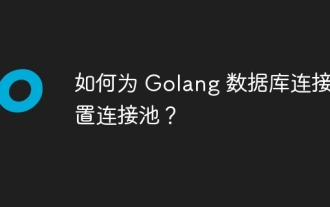
How to configure connection pool for Golang database connection?
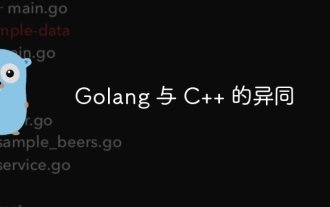
Similarities and Differences between Golang and C++
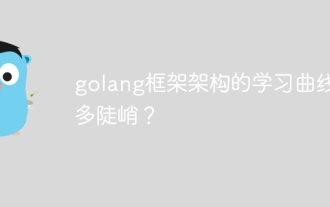
How steep is the learning curve of golang framework architecture?
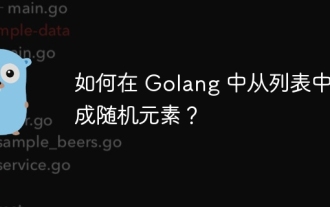
How to generate random elements from list in Golang?
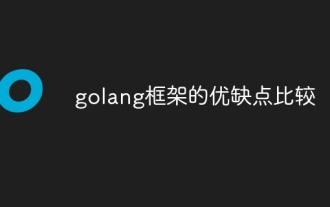
Comparison of advantages and disadvantages of golang framework

What are the best practices for error handling in Golang framework?
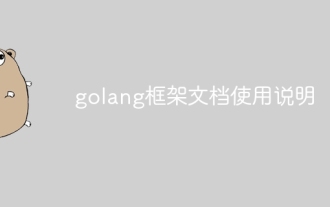
golang framework document usage instructions
