


In-depth understanding of various AJAX request methods: Detailed explanation of different AJAX request methods
Overview of AJAX request methods: To fully understand the different AJAX request methods, specific code examples are required
With the rapid development of the Internet and the increasing complexity of Web applications, It has become very common to use AJAX (Asynchronous JavaScript and XML) to request data in front-end development. AJAX can update part of the page content without refreshing the entire page, improving user experience and page loading speed.
In this article, we will take a comprehensive look at different AJAX request methods and provide specific code examples to help readers better understand and apply them.
- XMLHttpRequest
XMLHttpRequest is the most basic request object of AJAX. Basically all AJAX libraries are encapsulated based on XMLHttpRequest. The following is a simple XMLHttpRequest request example:
var xhr = new XMLHttpRequest(); xhr.open('GET', 'http://example.com/api/data', true); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var response = JSON.parse(xhr.responseText); console.log(response); } }; xhr.send();
- jQuery AJAX
jQuery is a very popular JavaScript library that provides simple and easy-to-use AJAX methods. Here is an example of an AJAX request using jQuery:
$.ajax({ url: 'http://example.com/api/data', method: 'GET', success: function(response) { console.log(response); }, error: function(xhr, status, error) { console.error(error); } });
- fetch API
fetch API is a modern, Promise-based AJAX request method with a cleaner API and better processing capabilities. The following is an example of an AJAX request using the fetch API:
fetch('http://example.com/api/data') .then(function(response) { if (response.ok) { return response.json(); } else { throw new Error('Request failed.'); } }) .then(function(data) { console.log(data); }) .catch(function(error) { console.error(error); });
- Axios
Axios is a popular Promise based HTTP client that can be used in browsers and Node.js environments . The following is an example of an AJAX request using Axios:
axios.get('http://example.com/api/data') .then(function(response) { console.log(response.data); }) .catch(function(error) { console.error(error); });
- Vue Resource
Vue Resource is an officially recommended AJAX library for Vue.js and is designed to integrate seamlessly with Vue.js. The following is an example of an AJAX request using Vue Resource:
Vue.http.get('http://example.com/api/data') .then(function(response) { console.log(response.body); }) .catch(function(error) { console.error(error); });
The above are several commonly used AJAX request methods, each of which has its own characteristics and applicable scenarios. By mastering these different AJAX request methods and their specific usage, we can more flexibly handle data requests and interaction needs in various front-end development.
In actual development, according to project requirements and team technology stack selection, select the appropriate AJAX library or request method for development. At the same time, no matter which method is used, we need to pay attention to common problems such as errors, timeouts, and cross-domain handling of requests to ensure the stability of the application and user experience.
Summary:
This article introduces several commonly used AJAX request methods, including XMLHttpRequest, jQuery AJAX, fetch API, Axios and Vue Resource. Each method has its own characteristics and applicable scenarios. Developers can decide which method to use based on project needs and technology stack selection. By learning and mastering these AJAX request methods, we can better handle data requests and interaction needs in front-end development, and improve user experience and page loading speed.
(Note: The above code is only a demonstration example and is not complete or operational. It needs to be adjusted and improved according to the specific situation during actual development.)
The above is the detailed content of In-depth understanding of various AJAX request methods: Detailed explanation of different AJAX request methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


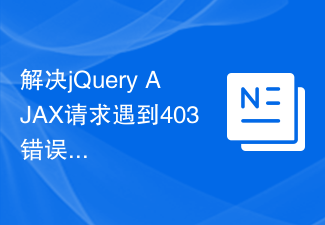
Title: Methods and code examples to resolve 403 errors in jQuery AJAX requests. The 403 error refers to a request that the server prohibits access to a resource. This error usually occurs because the request lacks permissions or is rejected by the server. When making jQueryAJAX requests, you sometimes encounter this situation. This article will introduce how to solve this problem and provide code examples. Solution: Check permissions: First ensure that the requested URL address is correct and verify that you have sufficient permissions to access the resource.
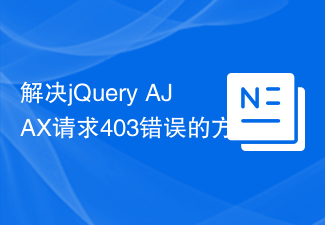
jQuery is a popular JavaScript library used to simplify client-side development. AJAX is a technology that sends asynchronous requests and interacts with the server without reloading the entire web page. However, when using jQuery to make AJAX requests, you sometimes encounter 403 errors. 403 errors are usually server-denied access errors, possibly due to security policy or permission issues. In this article, we will discuss how to resolve jQueryAJAX request encountering 403 error
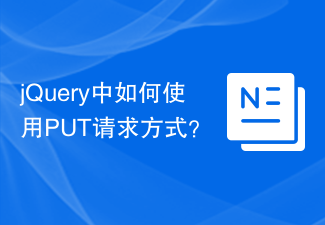
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
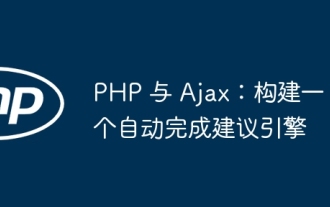
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
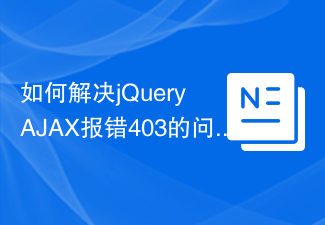
How to solve the problem of jQueryAJAX error 403? When developing web applications, jQuery is often used to send asynchronous requests. However, sometimes you may encounter error code 403 when using jQueryAJAX, indicating that access is forbidden by the server. This is usually caused by server-side security settings, but there are ways to work around it. This article will introduce how to solve the problem of jQueryAJAX error 403 and provide specific code examples. 1. to make
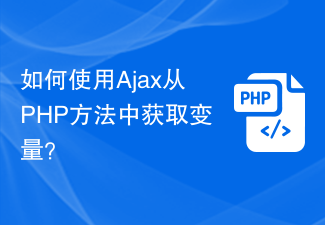
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
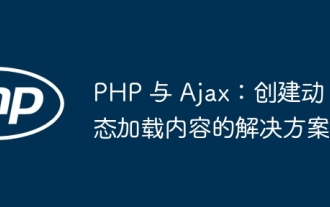
Ajax (Asynchronous JavaScript and XML) allows adding dynamic content without reloading the page. Using PHP and Ajax, you can dynamically load a product list: HTML creates a page with a container element, and the Ajax request adds the data to that element after loading it. JavaScript uses Ajax to send a request to the server through XMLHttpRequest to obtain product data in JSON format from the server. PHP uses MySQL to query product data from the database and encode it into JSON format. JavaScript parses the JSON data and displays it in the page container. Clicking the button triggers an Ajax request to load the product list.
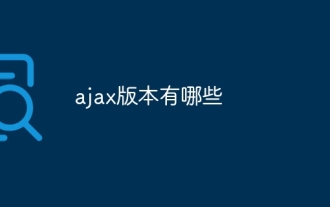
Ajax is not a specific version, but a technology that uses a collection of technologies to asynchronously load and update web page content. Ajax does not have a specific version number, but there are some variations or extensions of ajax: 1. jQuery AJAX; 2. Axios; 3. Fetch API; 4. JSONP; 5. XMLHttpRequest Level 2; 6. WebSockets; 7. Server-Sent Events; 8, GraphQL, etc.
