Research on the combined use of multiple inheritance and interfaces in Java
Explore the use of multiple inheritance and interfaces in Java
In Java, multiple inheritance means that a class can inherit the characteristics and behaviors of multiple parent classes at the same time. However, since Java only supports single inheritance, this means that a class can only inherit from one parent class and cannot directly implement the interfaces of multiple parent classes. In order to solve this problem, Java provides the concept of interface (Interface), allowing a class to implement multiple interfaces, thereby indirectly achieving the effect of multiple inheritance. In this article, we will explore how to use multiple inheritance with interfaces in Java and provide specific code examples.
First, let’s understand the concepts and characteristics of multiple inheritance and interfaces.
- Multiple inheritance: Multiple inheritance means that a class can inherit the characteristics and behaviors of multiple parent classes at the same time. Through multiple inheritance, subclasses can inherit properties and methods from multiple parent classes to better meet their needs. However, the problem caused by multiple inheritance is that it is prone to naming conflicts and code complexity, so multiple inheritance is not directly supported in Java.
- Interface: An interface is an abstract class that only contains method declarations without specific implementations. By implementing an interface, a class can obtain the methods defined by the interface and implement them concretely. Interfaces in Java can be used to define specifications and constraints, while also increasing the reusability and extensibility of code.
Next, we use a specific example to demonstrate the use of multiple inheritance and interfaces.
Suppose we have an animal class (Animal) and a plant class (Plant), which have their own attributes and behaviors respectively. We want to create an Organism class that inherits both Animal and Plant classes and implements their properties and behaviors.
First, we create the Animal class (Animal) and the Plant class (Plant), and define their properties and behaviors respectively:
// 动物类 class Animal { protected String name; public Animal(String name) { this.name = name; } public void eat() { System.out.println(name + " is eating."); } public void sleep() { System.out.println(name + " is sleeping."); } } // 植物类 class Plant { protected String name; public Plant(String name) { this.name = name; } public void grow() { System.out.println(name + " is growing."); } public void bloom() { System.out.println(name + " is blooming."); } }
Then, we create the Organism class (Organism), through the interface To realize the characteristics and behaviors of animals and plants:
// 生物类 class Organism implements Animal, Plant { private String name; public Organism(String name) { this.name = name; } public void eat() { System.out.println(name + " is eating."); } public void sleep() { System.out.println(name + " is sleeping."); } public void grow() { System.out.println(name + " is growing."); } public void bloom() { System.out.println(name + " is blooming."); } }
In the above code, we obtain the interfaces (Animal and Plant) of animals and plants by letting the organism (Organism) Characteristics and behavior of animals and plants.
Now, we can create the organism object and call the corresponding method to verify the correctness of the code:
public class Main { public static void main(String[] args) { Organism organism = new Organism("Organism"); organism.eat(); // 输出:Organism is eating. organism.sleep(); // 输出:Organism is sleeping. organism.grow(); // 输出:Organism is growing. organism.bloom(); // 输出:Organism is blooming. } }
By running the above code, we can see that the organism object (Organism) is successful Inherits the characteristics and behaviors of animals and plants, achieving the effect of multiple inheritance.
To sum up, although Java does not directly support multiple inheritance, through interfaces, we can indirectly achieve the effect of multiple inheritance. In the above example, we successfully obtained the characteristics and behaviors of animal and plant classes by letting the biological class implement the interfaces of animal and plant classes at the same time. By rationally using the combination of multiple inheritance and interfaces, we can improve the reusability and scalability of the code and better meet the needs.
The above is the detailed content of Research on the combined use of multiple inheritance and interfaces in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


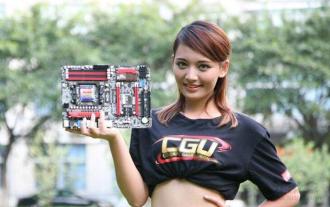
When we assemble the computer, although the installation process is simple, we often encounter problems in the wiring. Often, users mistakenly plug the power supply line of the CPU radiator into the SYS_FAN. Although the fan can rotate, it may not work when the computer is turned on. There will be an F1 error "CPUFanError", which also causes the CPU cooler to be unable to adjust the speed intelligently. Let's share the common knowledge about the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard. Popular science on the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard 1. CPU_FANCPU_FAN is a dedicated interface for the CPU radiator and works at 12V
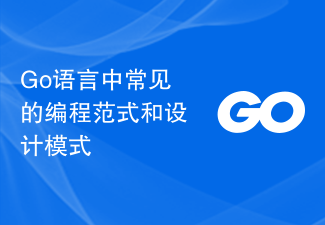
As a modern and efficient programming language, Go language has rich programming paradigms and design patterns that can help developers write high-quality, maintainable code. This article will introduce common programming paradigms and design patterns in the Go language and provide specific code examples. 1. Object-oriented programming In the Go language, you can use structures and methods to implement object-oriented programming. By defining a structure and binding methods to the structure, the object-oriented features of data encapsulation and behavior binding can be achieved. packagemaini
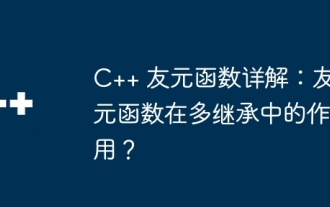
Friend functions allow non-member functions to access private members and play a role in multiple inheritance, allowing derived class functions to access private members of the base class.
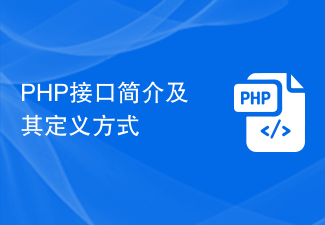
Introduction to PHP interface and how it is defined. PHP is an open source scripting language widely used in Web development. It is flexible, simple, and powerful. In PHP, an interface is a tool that defines common methods between multiple classes, achieving polymorphism and making code more flexible and reusable. This article will introduce the concept of PHP interfaces and how to define them, and provide specific code examples to demonstrate their usage. 1. PHP interface concept Interface plays an important role in object-oriented programming, defining the class application
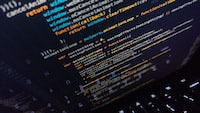
The reason for the error is in python. The reason why NotImplementedError() is thrown in Tornado may be because an abstract method or interface is not implemented. These methods or interfaces are declared in the parent class but not implemented in the child class. Subclasses need to implement these methods or interfaces to work properly. How to solve this problem is to implement the abstract method or interface declared by the parent class in the child class. If you are using a class to inherit from another class and you see this error, you should implement all the abstract methods declared in the parent class in the child class. If you are using an interface and you see this error, you should implement all methods declared in the interface in the class that implements the interface. If you are not sure which
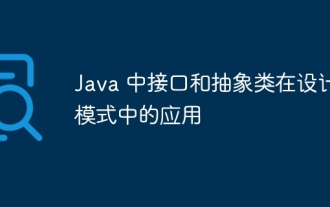
Interfaces and abstract classes are used in design patterns for decoupling and extensibility. Interfaces define method signatures, abstract classes provide partial implementation, and subclasses must implement unimplemented methods. In the strategy pattern, the interface is used to define the algorithm, and the abstract class or concrete class provides the implementation, allowing dynamic switching of algorithms. In the observer pattern, interfaces are used to define observer behavior, and abstract or concrete classes are used to subscribe and publish notifications. In the adapter pattern, interfaces are used to adapt existing classes. Abstract classes or concrete classes can implement compatible interfaces, allowing interaction with original code.
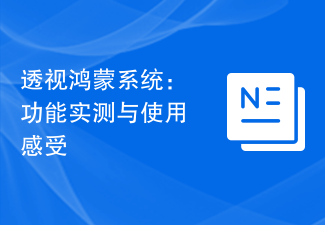
As a new operating system launched by Huawei, Hongmeng system has caused quite a stir in the industry. As a new attempt by Huawei after the US ban, Hongmeng system has high hopes and expectations. Recently, I was fortunate enough to get a Huawei mobile phone equipped with Hongmeng system. After a period of use and actual testing, I will share some functional testing and usage experience of Hongmeng system. First, let’s take a look at the interface and functions of Hongmeng system. The Hongmeng system adopts Huawei's own design style as a whole, which is simple, clear and smooth in operation. On the desktop, various

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
