


Detailed analysis of the functions and usage of the Go language standard library
In-depth analysis: The functions and usage of the Go language standard library, specific code examples are required
Introduction:
The Go language is an open source statically typed programming language , designed to provide tools that make it easy to write performant, reliable, and concise code. The standard library of the Go language is the result of active development and contributions by its community, providing rich functions and convenient tools. This article will provide an in-depth analysis of the core functions and usage of the Go language standard library, and provide relevant code examples.
1. String processing:
In the standard library of Go language, the strings package provides us with various functions for processing strings. For example, we often need to check whether a string contains a certain substring. We can use the strings.Contains function:
import "strings" func main() { str := "hello world" if strings.Contains(str, "world") { fmt.Println("包含子串") } else { fmt.Println("不包含子串") } }
2. File operation:
The os package in the standard library of the Go language provides files and Functions for directory operations. We can use the os.Open function to open a file and perform read and write operations, as shown below:
import "os" func main() { file, err := os.Open("file.txt") if err != nil { fmt.Println("打开文件失败:", err) return } defer file.Close() // 进行文件读写操作 }
3. Network programming:
In terms of network programming, the standard library of the Go language provides the net package , you can easily perform various network operations. For example, we can use the net.Dial function to establish a TCP connection:
import "net" func main() { conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { fmt.Println("建立TCP连接失败:", err) return } defer conn.Close() // 进行网络通信操作 }
4. Concurrent programming:
Go language takes concurrent programming as its core, and the goroutine and channel in its standard library provide a Lightweight concurrency approach. We can use goroutine to start a new concurrent execution thread and use channel to communicate between threads. The following is an example of using goroutine and channel for concurrent programming:
import "fmt" func worker(done chan bool) { fmt.Println("正在处理任务...") // 执行一些任务 done <- true } func main() { done := make(chan bool) go worker(done) <-done fmt.Println("任务完成") }
5. Time and date processing:
The time package of Go language provides us with convenient time and date processing functions. For example, we can use the time.Now function to get the current time and date:
import "time" func main() { now := time.Now() fmt.Println("当前时间:", now) }
Conclusion:
This article provides an in-depth analysis of the core functions of the Go language standard library and provides relevant code examples. By learning and utilizing the standard library of the Go language, our development work can be made more efficient and convenient. At the same time, it is worth noting that the standard library of the Go language is only a piece of the ocean of the Go language. There are many excellent third-party libraries that can be used. I hope readers can continue to learn and explore and discover more efficient tools and technologies.
The above is the detailed content of Detailed analysis of the functions and usage of the Go language standard library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


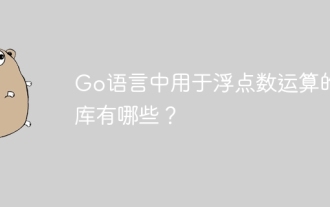
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
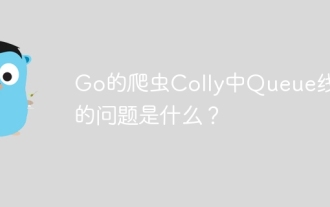
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
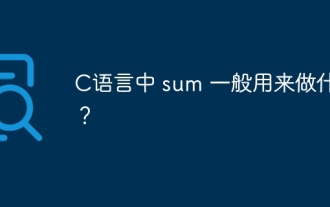
There is no function named "sum" in the C language standard library. "sum" is usually defined by programmers or provided in specific libraries, and its functionality depends on the specific implementation. Common scenarios are summing for arrays, and can also be used in other data structures, such as linked lists. In addition, "sum" is also used in fields such as image processing and statistical analysis. An excellent "sum" function should have good readability, robustness and efficiency.
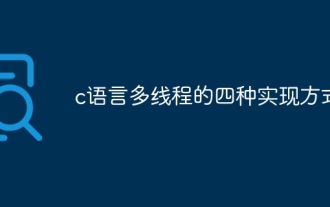
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
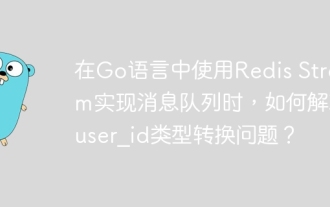
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
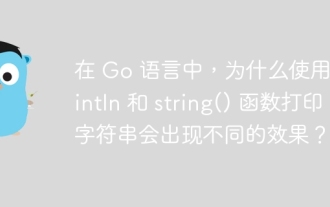
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
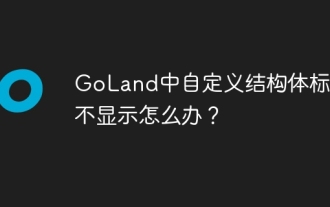
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
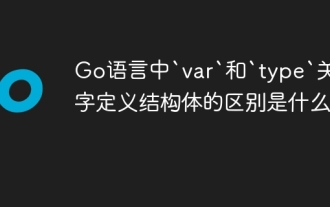
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
