An in-depth analysis of the array data structure in Go language
Array data structure:
An array is a basic data structure that contains a series of elements, each element has an index . The elements in an array can be of any type, including other arrays. The size of an array is determined when it is created and cannot be changed later.
Code example:
// 创建一个包含 5 个整数的数组 var numbers [5]int // 给数组中的元素赋值 numbers[0] = 1 numbers[1] = 2 numbers[2] = 3 numbers[3] = 4 numbers[4] = 5 // 遍历数组中的元素并打印出来 for i := 0; i < len(numbers); i++ { fmt.Println(numbers[i]) }
Output:
1 2 3 4 5
Length of array:
The length of the array can be obtained using the len()
function.
// 获取数组的长度 length := len(numbers) // 打印数组的长度 fmt.Println(length)
Output:
5
Elements of the array:
The elements of the array can be accessed by index. Indexing starts at 0 and ends at the length of the array minus one.
// 获取数组第一个元素 firstElement := numbers[0] // 打印第一个元素 fmt.Println(firstElement)
Output:
1
Array traversal:
Arrays can be traversed using for
.
// 遍历数组中的元素并打印出来 for i := 0; i < len(numbers); i++ { fmt.Println(numbers[i]) }
Output:
1 2 3 4 5
Multidimensional array:
The Go language also supports multidimensional arrays. Multidimensional arrays are arrays of arrays.
// 创建一个二维数组 var matrix [3][3]int // 给二维数组中的元素赋值 matrix[0][0] = 1 matrix[0][1] = 2 matrix[0][2] = 3 matrix[1][0] = 4 matrix[1][1] = 5 matrix[1][2] = 6 matrix[2][0] = 7 matrix[2][1] = 8 matrix[2][2] = 9 // 遍历二维数组中的元素并打印出来 for i := 0; i < len(matrix); i++ { for j := 0; j < len(matrix[i]); j++ { fmt.Println(matrix[i][j]) } }
Output:
1 2 3 4 5 6 7 8 9
Slice of array:
A slice of array is a part of the array. Slices can be created using the []
operator.
// 创建一个数组的切片 slice := numbers[1:3] // 打印切片中的元素 fmt.Println(slice)
Output:
[2 3]
Comparison of arrays:
Arrays can be used using ==
and !=
operator for comparison.
// 创建两个数组 var numbers1 = [5]int{1, 2, 3, 4, 5} var numbers2 = [5]int{1, 2, 3, 4, 5} // 比较两个数组是否相等 fmt.Println(numbers1 == numbers2)
Output:
true
Copy of array:
Arrays can be usedcopy()
function is copied.
// 创建一个数组 var numbers = [5]int{1, 2, 3, 4, 5} // 创建一个新的数组 var newNumbers = [5]int{} // 将数组拷贝到新的数组中 copy(newNumbers, numbers) // 打印新的数组 fmt.Println(newNumbers)
Output:
[1 2 3 4 5]
The above is the detailed content of An in-depth analysis of the array data structure in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
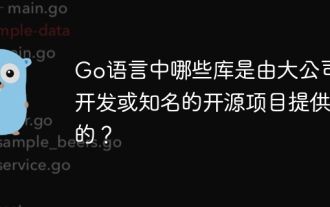
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
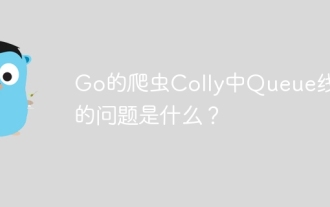
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
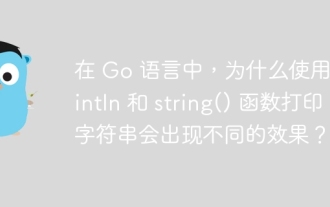
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
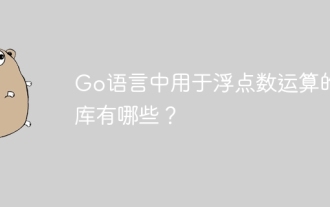
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
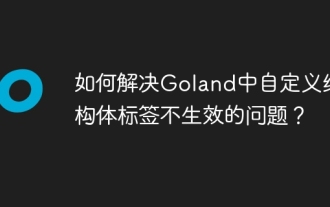
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
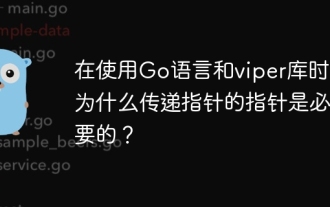
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
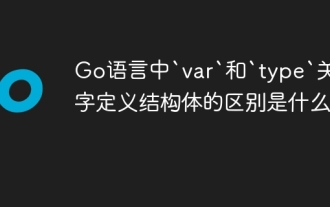
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
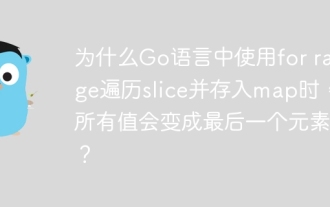
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
